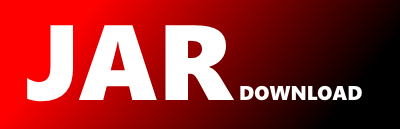
org.modeshape.jcr.query.Tuples Maven / Gradle / Ivy
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.modeshape.jcr.query;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import org.infinispan.schematic.internal.HashCode;
import org.mapdb.Serializer;
import org.modeshape.common.util.ObjectUtil;
import org.modeshape.jcr.query.model.TypeSystem.TypeFactory;
import org.modeshape.jcr.value.ValueFormatException;
/**
* A simple set of classes for working with tuples of data.
*
* @author Randall Hauch ([email protected])
*/
public class Tuples {
private Tuples() {
}
/**
* Create a tuple with the given two values.
*
* @param v1 the first value
* @param v2 the second value
* @return the tuple; never null
*/
public static Tuple2 tuple( T1 v1,
T2 v2 ) {
return new Tuple2(v1, v2);
}
/**
* Create a tuple with the given three values.
*
* @param v1 the first value
* @param v2 the second value
* @param v3 the third value
* @return the tuple; never null
*/
public static Tuple3 tuple( T1 v1,
T2 v2,
T3 v3 ) {
return new Tuple3(v1, v2, v3);
}
/**
* Create a tuple with the given four values.
*
* @param v1 the first value
* @param v2 the second value
* @param v3 the third value
* @param v4 the fourth value
* @return the tuple; never null
*/
public static Tuple4 tuple( T1 v1,
T2 v2,
T3 v3,
T4 v4 ) {
return new Tuple4(v1, v2, v3, v4);
}
/**
* Create a tuple with the given values. Note that this always creates a n-ary tuple, so use {@link #tuple(Object, Object)},
* {@link #tuple(Object, Object, Object)} or {@link #tuple(Object, Object, Object, Object)} for tuples smaller than 5.
*
* @param values the values
* @return the tuple; never null
*/
public static TupleN tuple( Object[] values ) {
return new TupleN(values);
}
/**
* Create a type factory for tuples of size 2.
*
* @param type1 the first type; may not be null
* @param type2 the second type; may not be null
* @return the type factory; never null
*/
public static TypeFactory> typeFactory( TypeFactory type1,
TypeFactory type2 ) {
return new Tuple2TypeFactory(type1, type2);
}
/**
* Create a type factory for tuples of size 3.
*
* @param type1 the first type; may not be null
* @param type2 the second type; may not be null
* @param type3 the third type; may not be null
* @return the type factory; never null
*/
public static TypeFactory> typeFactory( TypeFactory type1,
TypeFactory type2,
TypeFactory type3 ) {
return new Tuple3TypeFactory(type1, type2, type3);
}
/**
* Create a type factory for tuples of size 4.
*
* @param type1 the first type; may not be null
* @param type2 the second type; may not be null
* @param type3 the third type; may not be null
* @param type4 the fourth type; may not be null
* @return the type factory; never null
*/
public static TypeFactory> typeFactory( TypeFactory type1,
TypeFactory type2,
TypeFactory type3,
TypeFactory type4 ) {
return new Tuple4TypeFactory(type1, type2, type3, type4);
}
/**
* Create a type factory for n-ary tuples.
*
* @param types the collection of types for each slot in the tuple
* @return the type factory; never null
*/
public static TypeFactory> typeFactory( Collection> types ) {
return new TupleNTypeFactory(types);
}
/**
* Create a type factory for uniform tuples.
*
* @param type the type of each/every slot in the tuples
* @param tupleSize the size of the tuples
* @return the type factory; never null
*/
public static TypeFactory> typeFactory( TypeFactory> type,
int tupleSize ) {
if (tupleSize <= 1) return type;
if (tupleSize == 2) return typeFactory(type, type);
if (tupleSize == 3) return typeFactory(type, type, type);
if (tupleSize == 4) return typeFactory(type, type, type, type);
Collection> types = new ArrayList>(tupleSize);
for (int i = 0; i != tupleSize; ++i) {
types.add(type);
}
return new TupleNTypeFactory(types);
}
/**
* Create a {@link Serializer} for tuples of size 2.
*
* @param first the serializer for the first slot
* @param second the serializer for the second slot
* @return the serializer; never null
*/
public static Serializer> serializer( Serializer first,
Serializer second ) {
return new Tuple2Serializer(first, second);
}
/**
* Create a {@link Serializer} for tuples of size 3.
*
* @param first the serializer for the first slot
* @param second the serializer for the second slot
* @param third the serializer for the third slot
* @return the serializer; never null
*/
public static Serializer> serializer( Serializer first,
Serializer second,
Serializer third ) {
return new Tuple3Serializer(first, second, third);
}
/**
* Create a {@link Serializer} for tuples of size 4.
*
* @param first the serializer for the first slot
* @param second the serializer for the second slot
* @param third the serializer for the third slot
* @param fourth the serializer for the fourth slot
* @return the serializer; never null
*/
public static Serializer> serializer( Serializer first,
Serializer second,
Serializer third,
Serializer fourth ) {
return new Tuple4Serializer(first, second, third, fourth);
}
/**
* Create a {@link Serializer} for n-ary tuples
*
* @param serializers the serializers for each slot in the tuples
* @return the serializer; never null
*/
public static Serializer serializer( Serializer>[] serializers ) {
return new TupleNSerializer(serializers);
}
/**
* Create a {@link Serializer} for uniform tuples.
*
* @param serializer the serializer of each/every slot in the tuples
* @param tupleSize the size of the tuples
* @return the type factory; never null
*/
public static Serializer> serializer( Serializer> serializer,
int tupleSize ) {
if (tupleSize <= 1) return serializer;
if (tupleSize == 2) return serializer(serializer, serializer);
if (tupleSize == 3) return serializer(serializer, serializer, serializer);
if (tupleSize == 4) return serializer(serializer, serializer, serializer, serializer);
Serializer>[] serializers = new Serializer>[tupleSize];
Arrays.fill(serializers, serializer);
return new TupleNSerializer(serializers);
}
public static final class Tuple2Serializer implements Serializer>, Serializable {
private static final long serialVersionUID = 1L;
private final Serializer serializer1;
private final Serializer serializer2;
public Tuple2Serializer( Serializer serializer1,
Serializer serializer2 ) {
this.serializer1 = serializer1;
this.serializer2 = serializer2;
}
@Override
public int fixedSize() {
return 1; // not fixed size
}
@Override
public void serialize( DataOutput out,
Tuple2 value ) throws IOException {
serializer1.serialize(out, value.v1);
serializer2.serialize(out, value.v2);
}
@Override
public Tuple2 deserialize( DataInput in,
int available ) throws IOException {
T1 v1 = serializer1.deserialize(in, available);
T2 v2 = serializer2.deserialize(in, available);
return tuple(v1, v2);
}
}
public static final class Tuple3Serializer implements Serializer>, Serializable {
private static final long serialVersionUID = 1L;
private final Serializer serializer1;
private final Serializer serializer2;
private final Serializer serializer3;
public Tuple3Serializer( Serializer serializer1,
Serializer serializer2,
Serializer serializer3 ) {
this.serializer1 = serializer1;
this.serializer2 = serializer2;
this.serializer3 = serializer3;
}
@Override
public int fixedSize() {
return 1; // not fixed size
}
@Override
public void serialize( DataOutput out,
Tuple3 value ) throws IOException {
serializer1.serialize(out, value.v1);
serializer2.serialize(out, value.v2);
serializer3.serialize(out, value.v3);
}
@Override
public Tuple3 deserialize( DataInput in,
int available ) throws IOException {
T1 v1 = serializer1.deserialize(in, available);
T2 v2 = serializer2.deserialize(in, available);
T3 v3 = serializer3.deserialize(in, available);
return tuple(v1, v2, v3);
}
}
public static final class Tuple4Serializer implements Serializer>, Serializable {
private static final long serialVersionUID = 1L;
private final Serializer serializer1;
private final Serializer serializer2;
private final Serializer serializer3;
private final Serializer serializer4;
public Tuple4Serializer( Serializer serializer1,
Serializer serializer2,
Serializer serializer3,
Serializer serializer4 ) {
this.serializer1 = serializer1;
this.serializer2 = serializer2;
this.serializer3 = serializer3;
this.serializer4 = serializer4;
}
@Override
public int fixedSize() {
return 1; // not fixed size
}
@Override
public void serialize( DataOutput out,
Tuple4 value ) throws IOException {
serializer1.serialize(out, value.v1);
serializer2.serialize(out, value.v2);
serializer3.serialize(out, value.v3);
serializer4.serialize(out, value.v4);
}
@Override
public Tuple4 deserialize( DataInput in,
int available ) throws IOException {
T1 v1 = serializer1.deserialize(in, available);
T2 v2 = serializer2.deserialize(in, available);
T3 v3 = serializer3.deserialize(in, available);
T4 v4 = serializer4.deserialize(in, available);
return tuple(v1, v2, v3, v4);
}
}
public static final class TupleNSerializer implements Serializer, Serializable {
private static final long serialVersionUID = 1L;
private final Serializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy