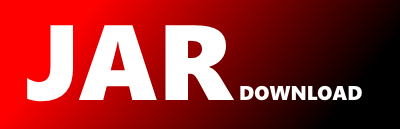
org.modeshape.jcr.federation.FederatedDocumentWriter Maven / Gradle / Ivy
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.modeshape.jcr.federation;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.infinispan.schematic.DocumentFactory;
import org.infinispan.schematic.document.Document;
import org.infinispan.schematic.document.EditableArray;
import org.infinispan.schematic.document.EditableDocument;
import org.modeshape.jcr.JcrLexicon;
import org.modeshape.jcr.cache.document.DocumentTranslator;
import org.modeshape.jcr.spi.federation.Connector;
import org.modeshape.jcr.spi.federation.DocumentWriter;
import org.modeshape.jcr.spi.federation.PageKey;
import org.modeshape.jcr.value.Name;
import org.modeshape.jcr.value.Property;
import org.modeshape.jcr.value.basic.BasicMultiValueProperty;
import org.modeshape.jcr.value.basic.BasicSingleValueProperty;
/**
* Default implementation of the {@link DocumentWriter} interface.
*
* @author Horia Chiorean ([email protected])
*/
public class FederatedDocumentWriter implements DocumentWriter {
private final EditableDocument federatedDocument;
private final DocumentTranslator translator;
public FederatedDocumentWriter( DocumentTranslator translator,
Document document ) {
this.federatedDocument = document != null ? DocumentFactory.newDocument(document) : DocumentFactory.newDocument();
this.translator = translator;
}
public FederatedDocumentWriter( DocumentTranslator translator ) {
this(translator, null);
}
@Override
public FederatedDocumentWriter setId( String id ) {
assert id != null;
translator.setKey(federatedDocument, id);
return this;
}
protected final Name nameFrom( String name ) {
return translator.getNameFactory().create(name);
}
@Override
public DocumentWriter setPrimaryType( Name name ) {
return addProperty(JcrLexicon.PRIMARY_TYPE, name);
}
@Override
public DocumentWriter setPrimaryType( String name ) {
return setPrimaryType(nameFrom(name));
}
@Override
public DocumentWriter addMixinType( Name name ) {
return addPropertyValue(JcrLexicon.MIXIN_TYPES, name);
}
@Override
public DocumentWriter addMixinType( String name ) {
return addMixinType(nameFrom(name));
}
@Override
public DocumentWriter addPropertyValue( Name name,
Object value ) {
boolean knownToBeMultiple = false;
translator.addPropertyValues(federatedDocument, name, knownToBeMultiple, Collections.singleton(value), null, null);
return this;
}
@Override
public DocumentWriter addPropertyValue( String name,
Object value ) {
return addPropertyValue(nameFrom(name), value);
}
@Override
public DocumentWriter addProperty( String name,
Object value ) {
Name nameObj = nameFrom(name);
return addProperty(nameObj, value);
}
@Override
public DocumentWriter addProperty( String name,
Object[] values ) {
if (values == null) return this;
Name nameObj = nameFrom(name);
return addProperty(nameObj, values);
}
@Override
public DocumentWriter addProperty( String name,
Object firstValue,
Object... additionalValues ) {
Name nameObj = nameFrom(name);
return addProperty(nameObj, firstValue, additionalValues);
}
@Override
public DocumentWriter addProperty( Name name,
Object value ) {
if (value == null) {
return this;
}
translator.setProperty(federatedDocument, new BasicSingleValueProperty(name, value), null, null);
return this;
}
@Override
public DocumentWriter addProperty( Name name,
Object[] values ) {
if (values == null) return this;
int len = values.length;
if (len == 0) return this;
if (len == 1) {
translator.setProperty(federatedDocument, new BasicSingleValueProperty(name, values[0]), null, null);
} else {
translator.setProperty(federatedDocument, new BasicMultiValueProperty(name, values), null, null);
}
return this;
}
@Override
public DocumentWriter addProperty( Name name,
Object firstValue,
Object... additionalValues ) {
if (additionalValues.length == 0) {
translator.setProperty(federatedDocument, new BasicSingleValueProperty(name, firstValue), null, null);
} else {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy