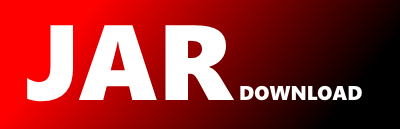
org.modeshape.jcr.security.JaasProvider Maven / Gradle / Ivy
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.modeshape.jcr.security;
import java.lang.reflect.Method;
import java.security.AccessController;
import java.security.PrivilegedActionException;
import java.security.PrivilegedExceptionAction;
import java.util.HashMap;
import java.util.Map;
import javax.jcr.Credentials;
import javax.jcr.SimpleCredentials;
import javax.security.auth.Subject;
import javax.security.auth.login.LoginContext;
import javax.security.auth.login.LoginException;
import org.modeshape.common.logging.Logger;
import org.modeshape.common.util.CheckArg;
import org.modeshape.jcr.ExecutionContext;
import org.modeshape.jcr.JcrI18n;
import org.modeshape.jcr.api.JaasCredentials;
/**
* An implementation of {@link AuthenticationProvider} that uses a supplied JAAS policy to perform all authentication and
* role-based authorization.
*/
public class JaasProvider implements AuthenticationProvider {
private final String policyName;
private final SubjectResolver subjectResolver;
/**
* Create a JAAS provider for authentication and authorization, using the supplied name for the login configuration.
*
* @param policyName the name that will be used for the login context
* @exception LoginException if the caller-specified name
does not appear in the Configuration
and
* there is no Configuration
entry for "other", or if the
* auth.login.defaultCallbackHandler security property was set, but the implementation class could not be
* loaded.
*
*/
public JaasProvider( String policyName ) throws LoginException {
CheckArg.isNotNull(policyName, "policyName");
this.policyName = policyName;
// verify that the login context is valid ...
new LoginContext(policyName);
// Per MODE-1270, see if the JACC API is available (if so, we're running in an J2EE container
// and need to provide a way to properly resolve the JAAS Subject)...
JaasProvider.SubjectResolver subjectResolver = null;
try {
// Try to find the JACC PolicyContext class, which is entirely optional and provided only in J2EE containers ...
getClass().getClassLoader().loadClass("javax.security.jacc.PolicyContext");
subjectResolver = new JaccSubjectResolver();
Logger.getLogger(getClass())
.debug("Enabling optional JACC approach for resolving the JAAS Subject (typically in J2EE containers)");
} catch (ClassNotFoundException cnfe) {
// Must not be able to load the class ...
Logger.getLogger(getClass())
.debug("Failed to find 'javax.security.jacc.PolicyContext', so assuming not in a J2EE container.");
}
this.subjectResolver = subjectResolver;
}
@Override
public ExecutionContext authenticate( final Credentials credentials,
String repositoryName,
String workspaceName,
ExecutionContext repositoryContext,
Map sessionAttributes ) {
try {
if (credentials == null) {
// There are no credentials, so see if there is an authenticated Subject ...
Subject subject = Subject.getSubject(AccessController.getContext());
if (subject != null) {
// There is, so use this subject ...
return repositoryContext.with(new JaasSecurityContext(subject));
}
if (subjectResolver != null) {
// The Subject is still null (see MODE-1270), so try to resolve it ...
subject = subjectResolver.resolveSubject();
if (subject != null) {
return repositoryContext.with(new JaasSecurityContext(subject));
}
}
// There is no authenticated JAAS Subject and no credentials, so we can do nothing ...
return null;
}
if (credentials instanceof SimpleCredentials) {
SimpleCredentials simple = (SimpleCredentials)credentials;
String[] attributeNames = simple.getAttributeNames();
if (attributeNames != null && attributeNames.length != 0) {
sessionAttributes = new HashMap();
for (String attributeName : simple.getAttributeNames()) {
Object attributeValue = simple.getAttribute(attributeName);
sessionAttributes.put(attributeName, attributeValue);
}
}
return repositoryContext.with(new JaasSecurityContext(policyName, simple.getUserID(), simple.getPassword()));
}
LoginContext loginContext = null;
if (credentials instanceof JaasCredentials) {
// Call directly ...
loginContext = ((JaasCredentials)credentials).getLoginContext();
} else {
// Look for a getter method ...
try {
final Method method = credentials.getClass().getMethod("getLoginContext");
Object result = AccessController.doPrivileged(new PrivilegedExceptionAction