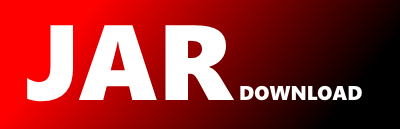
org.infinispan.schematic.internal.document.Utility Maven / Gradle / Ivy
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.infinispan.schematic.internal.document;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.Set;
import org.infinispan.schematic.document.Array;
import org.infinispan.schematic.document.Document;
class Utility {
public static Array unwrap( Array array ) {
if (array instanceof ArrayEditor) {
return unwrap(((ArrayEditor)array).unwrap());
}
return array;
}
public static Document unwrap( Document document ) {
if (document instanceof DocumentEditor) {
return unwrap(((DocumentEditor)document).unwrap());
}
return document;
}
public static Object unwrap( Object value ) {
if (value instanceof DocumentEditor) {
return unwrap(((DocumentEditor)value).unwrap());
}
if (value instanceof ArrayEditor) {
return unwrap(((ArrayEditor)value).unwrap());
}
return value;
}
@SuppressWarnings( "unchecked" )
public static Map extends String, ? extends Object> unwrapValues( Map extends String, ? extends Object> map ) {
if (map == null || map.isEmpty()) return map;
Map newMap = (Map)map; // just cast
for (Map.Entry extends String, ? extends Object> entry : map.entrySet()) {
Object orig = entry.getValue();
Object unwrapped = unwrap(orig);
if (orig != unwrapped) {
String key = entry.getKey();
newMap.put(key, unwrapped);
}
}
return newMap;
}
@SuppressWarnings( "unchecked" )
public static Collection extends Object> unwrapValues( Collection> c ) {
if (c == null || c.isEmpty()) return c;
if (c instanceof Set>) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy