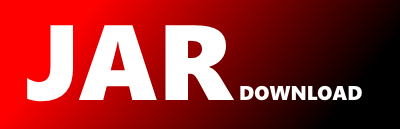
org.molgenis.migrate.version.v1_21.Step30MigrateJobExecutionUser Maven / Gradle / Ivy
package org.molgenis.migrate.version.v1_21;
import static java.util.Objects.requireNonNull;
import javax.sql.DataSource;
import org.molgenis.framework.MolgenisUpgrade;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
/**
*
* - Changes JobExecution user columns from foreign key to MolgenisUser to a simple userName.
*
*/
public class Step30MigrateJobExecutionUser extends MolgenisUpgrade
{
private static final Logger LOG = LoggerFactory.getLogger(Step30MigrateJobExecutionUser.class);
private final JdbcTemplate jdbcTemplate;
@Autowired
public Step30MigrateJobExecutionUser(DataSource dataSource)
{
super(29, 30);
requireNonNull(dataSource);
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public void upgrade()
{
if (checkPreviouslyUpgraded())
{
LOG.info(
"Skipping the upgrading of JobExecution entities because it has already been done in a previous version.");
}
else
{
LOG.info("Upgrade user attribute in JobExecution entities...");
updateDataType("JobExecution", "user", "string");
dropForeignKey("SortaJobExecution");
dropForeignKey("FileIngestJobExecution");
dropForeignKey("AnnotationJobExecution");
dropForeignKey("GavinJobExecution");
LOG.info("Done.");
}
}
/**
* Checks if this migration step needs to be executed for the current version. There is the possibility a previous
* version has already done this upgrade because this migration step and its corresponding fix might be added to
* older versions.
*/
private boolean checkPreviouslyUpgraded()
{
int count = jdbcTemplate.queryForObject(
"SELECT COUNT(*) FROM entities_attributes ea JOIN attributes a ON ea.attributes = a.identifier WHERE ea.fullName = 'JobExecution' AND a.name = 'user' AND dataType = 'xref'",
Integer.class);
return (count == 0);
}
private void updateDataType(String entityFullName, String attributeName, String newDataType)
{
LOG.info("Update data type of {}.{} to {}...", entityFullName, attributeName, newDataType);
String attributeId = jdbcTemplate.queryForObject(
"SELECT a.identifier FROM entities_attributes ea JOIN attributes a ON ea.attributes = a.identifier WHERE ea.fullName = '"
+ entityFullName + "' AND a.name='" + attributeName + "'",
String.class);
jdbcTemplate.update("UPDATE attributes SET dataType = '" + newDataType
+ "', refEntity = NULL WHERE identifier = '" + attributeId + "'");
}
private void dropForeignKey(String entityFullName)
{
LOG.info("Drop foreign key and index from {} to MolgenisUser...", entityFullName);
jdbcTemplate.update("ALTER TABLE `" + entityFullName + "` DROP FOREIGN KEY `" + entityFullName + "_ibfk_1`");
jdbcTemplate.update("DROP INDEX user ON `" + entityFullName + "`");
jdbcTemplate.update("UPDATE `" + entityFullName
+ "` SET user = (SELECT userName from MolgenisUser WHERE MolgenisUser.ID = `" + entityFullName
+ "`.user)");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy