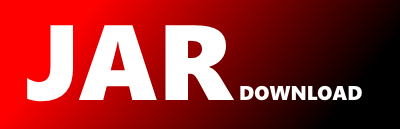
org.molgenis.data.rest.EntityMetaDataResponse Maven / Gradle / Ivy
package org.molgenis.data.rest;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.elasticsearch.common.collect.Lists;
import org.molgenis.data.AttributeMetaData;
import org.molgenis.data.DataService;
import org.molgenis.data.EntityMetaData;
import org.molgenis.data.RepositoryCapability;
import org.molgenis.data.i18n.LanguageService;
import org.molgenis.security.core.MolgenisPermissionService;
import org.molgenis.security.core.Permission;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Iterables;
import com.google.common.collect.Sets;
public class EntityMetaDataResponse
{
private final String href;
private final String hrefCollection;
private final String name;
private final String label;
private final String description;
private final Map attributes;
private final String labelAttribute;
private final String idAttribute;
private final List lookupAttributes;
private final Boolean isAbstract;
private String languageCode;
/**
* Is this user allowed to add/update/delete entities of this type and has the repo the capability?
*/
private final Boolean writable;
/**
* @param meta
*/
public EntityMetaDataResponse(EntityMetaData meta, MolgenisPermissionService permissionService,
DataService dataService, LanguageService languageService)
{
this(meta, null, null, permissionService, dataService, languageService);
}
/**
*
* @param meta
* @param attributesSet
* set of lowercase attribute names to include in response
* @param attributeExpandsSet
* set of lowercase attribute names to expand in response
*/
public EntityMetaDataResponse(EntityMetaData meta, Set attributesSet,
Map> attributeExpandsSet, MolgenisPermissionService permissionService,
DataService dataService, LanguageService languageService)
{
String name = meta.getName();
this.href = Href.concatMetaEntityHref(RestController.BASE_URI, name);
this.hrefCollection = String.format("%s/%s", RestController.BASE_URI, name); // FIXME apply Href escaping fix
this.languageCode = languageService.getCurrentUserLanguageCode();
if (attributesSet == null || attributesSet.contains("name".toLowerCase()))
{
this.name = name;
}
else this.name = null;
if (attributesSet == null || attributesSet.contains("description".toLowerCase()))
{
this.description = meta.getDescription(languageService.getCurrentUserLanguageCode());
}
else this.description = null;
if (attributesSet == null || attributesSet.contains("label".toLowerCase()))
{
label = meta.getLabel(languageService.getCurrentUserLanguageCode());
}
else this.label = null;
if (attributesSet == null || attributesSet.contains("attributes".toLowerCase()))
{
this.attributes = new LinkedHashMap();
//the newArraylist is a fix for concurrency trouble
//FIXME properly fix this by making metadata immutable
for (AttributeMetaData attr : Lists.newArrayList(meta.getAttributes()))
{
if (!attr.getName().equals("__Type"))
{
if (attributeExpandsSet != null && attributeExpandsSet.containsKey("attributes".toLowerCase()))
{
Set subAttributesSet = attributeExpandsSet.get("attributes".toLowerCase());
this.attributes.put(attr.getName(),
new AttributeMetaDataResponse(name, meta, attr, subAttributesSet,
Collections.singletonMap("refEntity".toLowerCase(),
Sets.newHashSet("idattribute")),
permissionService, dataService, languageService));
}
else
{
String attrHref = Href.concatMetaAttributeHref(RestController.BASE_URI, name, attr.getName());
this.attributes.put(attr.getName(), Collections.singletonMap("href", attrHref));
}
}
}
}
else this.attributes = null;
if (attributesSet == null || attributesSet.contains("labelAttribute".toLowerCase()))
{
AttributeMetaData labelAttribute = meta.getLabelAttribute(this.languageCode);
this.labelAttribute = labelAttribute != null ? labelAttribute.getName() : null;
}
else this.labelAttribute = null;
if (attributesSet == null || attributesSet.contains("idAttribute".toLowerCase()))
{
AttributeMetaData idAttribute = meta.getIdAttribute();
this.idAttribute = idAttribute != null ? idAttribute.getName() : null;
}
else this.idAttribute = null;
if (attributesSet == null || attributesSet.contains("lookupAttributes".toLowerCase()))
{
Iterable lookupAttributes = meta.getLookupAttributes();
this.lookupAttributes = lookupAttributes != null
? Lists.newArrayList(Iterables.transform(lookupAttributes, new Function()
{
@Override
public String apply(AttributeMetaData attribute)
{
return attribute.getName();
}
})) : null;
}
else this.lookupAttributes = null;
if (attributesSet == null || attributesSet.contains("abstract".toLowerCase()))
{
isAbstract = meta.isAbstract();
}
else this.isAbstract = null;
this.writable = permissionService.hasPermissionOnEntity(name, Permission.WRITE)
&& dataService.getCapabilities(name).contains(RepositoryCapability.WRITABLE);
}
public String getHref()
{
return href;
}
public String getHrefCollection()
{
return hrefCollection;
}
public String getName()
{
return name;
}
public String getLabel()
{
return label;
}
public String getDescription()
{
return description;
}
public String getIdAttribute()
{
return idAttribute;
}
public List getLookupAttributes()
{
return lookupAttributes;
}
public Map getAttributes()
{
return ImmutableMap.copyOf(attributes);
}
public String getLabelAttribute()
{
return labelAttribute;
}
public boolean isAbstract()
{
return isAbstract;
}
public Boolean getWritable()
{
return writable;
}
public String getLanguageCode()
{
return languageCode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy