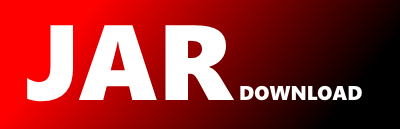
org.molgenis.data.rest.v2.EntityAggregatesResponse Maven / Gradle / Ivy
package org.molgenis.data.rest.v2;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.molgenis.data.AggregateAnonymizer;
import org.molgenis.data.AggregateResult;
import org.molgenis.data.AnonymizedAggregateResult;
import org.molgenis.data.AttributeMetaData;
import org.molgenis.data.Entity;
public class EntityAggregatesResponse extends EntityCollectionResponseV2
{
private final AggregateResultResponse aggs;
private final AttributeMetaDataResponseV2 xAttr;
private final AttributeMetaDataResponseV2 yAttr;
public EntityAggregatesResponse(AggregateResult aggs, AttributeMetaDataResponseV2 xAttr,
AttributeMetaDataResponseV2 yAttr, String href)
{
super(href);
this.aggs = checkNotNull(AggregateResultResponse.toResponse(aggs));
this.xAttr = xAttr;
this.yAttr = yAttr;
}
public AggregateResultResponse getAggs()
{
return aggs;
}
public AttributeMetaDataResponseV2 getXAttr()
{
return xAttr;
}
public AttributeMetaDataResponseV2 getYAttr()
{
return yAttr;
}
public static class AggregateResultResponse
{
private final List> matrix;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy