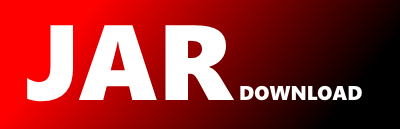
com.mongodb.hadoop.streaming.io.MongoOutputReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongo-hadoop-streaming Show documentation
Show all versions of mongo-hadoop-streaming Show documentation
The MongoDB Connector for Hadoop is a plugin for Hadoop that provides the ability to use MongoDB as an input source and/or an output destination.
package com.mongodb.hadoop.streaming.io;
import com.mongodb.BasicDBObject;
import com.mongodb.hadoop.io.BSONWritable;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.hadoop.streaming.PipeMapRed;
import org.apache.hadoop.streaming.io.OutputReader;
import java.io.DataInput;
import java.io.IOException;
public class MongoOutputReader extends OutputReader {
private DataInput in;
private static final Log LOG = LogFactory.getLog(MongoOutputReader.class);
private BSONWritable currentKey;
private BSONWritable currentValue;
@Override
public void initialize(final PipeMapRed pipeMapRed) throws IOException {
super.initialize(pipeMapRed);
in = pipeMapRed.getClientInput();
this.currentKey = new BSONWritable();
this.currentValue = new BSONWritable();
}
@Override
public boolean readKeyValue() throws IOException {
// Actually, just read the value as the key is embedded.
try {
currentValue.readFields(in);
Object id = currentValue.getDoc().get("_id");
currentKey.setDoc(new BasicDBObject("_id", id));
// If successful we'll have an _id field
return id != null;
} catch (IndexOutOfBoundsException e) {
// No more data
LOG.info("No more data; no key/value pair read.");
return false;
}
}
@Override
public BSONWritable getCurrentKey() throws IOException {
return currentKey;
}
@Override
public BSONWritable getCurrentValue() throws IOException {
return currentValue;
}
@Override
public String getLastOutput() {
return currentValue.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy