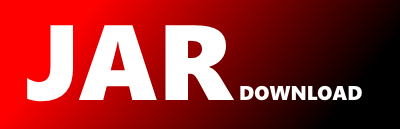
org.mongodb.awscdk.resources.mongodbatlas.CfnNetworkContainerProps Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Returns, adds, edits, and removes network peering containers.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.94.0 (build b380f01)", date = "2024-02-05T16:04:31.857Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnNetworkContainerProps")
@software.amazon.jsii.Jsii.Proxy(CfnNetworkContainerProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnNetworkContainerProps extends software.amazon.jsii.JsiiSerializable {
/**
* IP addresses expressed in Classless Inter-Domain Routing (CIDR) notation that MongoDB Cloud uses for the network peering containers in your project.
*
* MongoDB Cloud assigns all of the project's clusters deployed to this cloud provider an IP address from this range. MongoDB Cloud locks this value if an M10 or greater cluster or a network peering connection exists in this project.
* These CIDR blocks must fall within the ranges reserved per RFC 1918. AWS further limits the block to between the /24 and /21 ranges.
* To modify the CIDR block, the target project cannot have:
*
*
* - Any M10 or greater clusters
* - Any other VPC peering connections
* You can also create a new project and create a network peering connection to set the desired MongoDB Cloud network peering container CIDR block for that project. MongoDB Cloud limits the number of MongoDB nodes per network peering connection based on the CIDR block and the region selected for the project.
* Example: A project in an Amazon Web Services (AWS) region supporting three availability zones and an MongoDB CIDR network peering container block of limit of /24 equals 27 three-node replica sets.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAtlasCidrBlock();
/**
* Unique 24-hexadecimal digit string that identifies your project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getProjectId();
/**
* Geographic area that Amazon Web Services (AWS) defines to which MongoDB Cloud deployed this network peering container.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRegionName();
/**
* The profile is defined in AWS Secret manager.
*
* See Secret Manager Profile setup.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
* Boolean flag that indicates whether MongoDB Cloud clusters exist in the specified network peering container.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getProvisioned() {
return null;
}
/**
* Unique string that identifies the MongoDB Cloud VPC on AWS.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getVpcId() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnNetworkContainerProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnNetworkContainerProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String atlasCidrBlock;
java.lang.String projectId;
java.lang.String regionName;
java.lang.String profile;
java.lang.Boolean provisioned;
java.lang.String vpcId;
/**
* Sets the value of {@link CfnNetworkContainerProps#getAtlasCidrBlock}
* @param atlasCidrBlock IP addresses expressed in Classless Inter-Domain Routing (CIDR) notation that MongoDB Cloud uses for the network peering containers in your project. This parameter is required.
* MongoDB Cloud assigns all of the project's clusters deployed to this cloud provider an IP address from this range. MongoDB Cloud locks this value if an M10 or greater cluster or a network peering connection exists in this project.
* These CIDR blocks must fall within the ranges reserved per RFC 1918. AWS further limits the block to between the /24 and /21 ranges.
* To modify the CIDR block, the target project cannot have:
*
*
* - Any M10 or greater clusters
* - Any other VPC peering connections
* You can also create a new project and create a network peering connection to set the desired MongoDB Cloud network peering container CIDR block for that project. MongoDB Cloud limits the number of MongoDB nodes per network peering connection based on the CIDR block and the region selected for the project.
* Example: A project in an Amazon Web Services (AWS) region supporting three availability zones and an MongoDB CIDR network peering container block of limit of /24 equals 27 three-node replica sets.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder atlasCidrBlock(java.lang.String atlasCidrBlock) {
this.atlasCidrBlock = atlasCidrBlock;
return this;
}
/**
* Sets the value of {@link CfnNetworkContainerProps#getProjectId}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the value of {@link CfnNetworkContainerProps#getRegionName}
* @param regionName Geographic area that Amazon Web Services (AWS) defines to which MongoDB Cloud deployed this network peering container. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder regionName(java.lang.String regionName) {
this.regionName = regionName;
return this;
}
/**
* Sets the value of {@link CfnNetworkContainerProps#getProfile}
* @param profile The profile is defined in AWS Secret manager.
* See Secret Manager Profile setup.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link CfnNetworkContainerProps#getProvisioned}
* @param provisioned Boolean flag that indicates whether MongoDB Cloud clusters exist in the specified network peering container.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder provisioned(java.lang.Boolean provisioned) {
this.provisioned = provisioned;
return this;
}
/**
* Sets the value of {@link CfnNetworkContainerProps#getVpcId}
* @param vpcId Unique string that identifies the MongoDB Cloud VPC on AWS.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcId(java.lang.String vpcId) {
this.vpcId = vpcId;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnNetworkContainerProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnNetworkContainerProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnNetworkContainerProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnNetworkContainerProps {
private final java.lang.String atlasCidrBlock;
private final java.lang.String projectId;
private final java.lang.String regionName;
private final java.lang.String profile;
private final java.lang.Boolean provisioned;
private final java.lang.String vpcId;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.atlasCidrBlock = software.amazon.jsii.Kernel.get(this, "atlasCidrBlock", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.projectId = software.amazon.jsii.Kernel.get(this, "projectId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.regionName = software.amazon.jsii.Kernel.get(this, "regionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.provisioned = software.amazon.jsii.Kernel.get(this, "provisioned", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.vpcId = software.amazon.jsii.Kernel.get(this, "vpcId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.atlasCidrBlock = java.util.Objects.requireNonNull(builder.atlasCidrBlock, "atlasCidrBlock is required");
this.projectId = java.util.Objects.requireNonNull(builder.projectId, "projectId is required");
this.regionName = java.util.Objects.requireNonNull(builder.regionName, "regionName is required");
this.profile = builder.profile;
this.provisioned = builder.provisioned;
this.vpcId = builder.vpcId;
}
@Override
public final java.lang.String getAtlasCidrBlock() {
return this.atlasCidrBlock;
}
@Override
public final java.lang.String getProjectId() {
return this.projectId;
}
@Override
public final java.lang.String getRegionName() {
return this.regionName;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final java.lang.Boolean getProvisioned() {
return this.provisioned;
}
@Override
public final java.lang.String getVpcId() {
return this.vpcId;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("atlasCidrBlock", om.valueToTree(this.getAtlasCidrBlock()));
data.set("projectId", om.valueToTree(this.getProjectId()));
data.set("regionName", om.valueToTree(this.getRegionName()));
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getProvisioned() != null) {
data.set("provisioned", om.valueToTree(this.getProvisioned()));
}
if (this.getVpcId() != null) {
data.set("vpcId", om.valueToTree(this.getVpcId()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CfnNetworkContainerProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnNetworkContainerProps.Jsii$Proxy that = (CfnNetworkContainerProps.Jsii$Proxy) o;
if (!atlasCidrBlock.equals(that.atlasCidrBlock)) return false;
if (!projectId.equals(that.projectId)) return false;
if (!regionName.equals(that.regionName)) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
if (this.provisioned != null ? !this.provisioned.equals(that.provisioned) : that.provisioned != null) return false;
return this.vpcId != null ? this.vpcId.equals(that.vpcId) : that.vpcId == null;
}
@Override
public final int hashCode() {
int result = this.atlasCidrBlock.hashCode();
result = 31 * result + (this.projectId.hashCode());
result = 31 * result + (this.regionName.hashCode());
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.provisioned != null ? this.provisioned.hashCode() : 0);
result = 31 * result + (this.vpcId != null ? this.vpcId.hashCode() : 0);
return result;
}
}
}