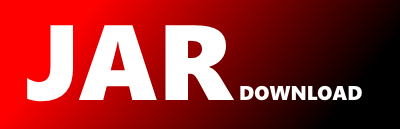
org.mongodb.awscdk.resources.mongodbatlas.CriteriaView Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.94.0 (build b380f01)", date = "2024-02-05T16:04:31.919Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CriteriaView")
@software.amazon.jsii.Jsii.Proxy(CriteriaView.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CriteriaView extends software.amazon.jsii.JsiiSerializable {
/**
* Indexed database parameter that stores the date that determines when data moves to the online archive.
*
* MongoDB Cloud archives the data when the current date exceeds the date in this database parameter plus the number of days specified through the expireAfterDays parameter. Set this parameter when you set "criteria.type" : "DATE".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDateField() {
return null;
}
/**
* Syntax used to write the date after which data moves to the online archive.
*
* Date can be expressed as ISO 8601 or Epoch timestamps. The Epoch timestamp can be expressed as nanoseconds, milliseconds, or seconds. Set this parameter when "criteria.type" : "DATE". You must set "criteria.type" : "DATE" if "collectionType": "TIMESERIES".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat getDateFormat() {
return null;
}
/**
* Number of days after the value in the criteria.dateField when MongoDB Cloud archives data in the specified cluster. Set this parameter when you set "criteria.type" : "DATE".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getExpireAfterDays() {
return null;
}
/**
* MongoDB find query that selects documents to archive.
*
* The specified query follows the syntax of the db.collection.find(query) command. This query can't use the empty document ({}) to return all documents. Set this parameter when "criteria.type" : "CUSTOM".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getQuery() {
return null;
}
/**
* Means by which MongoDB Cloud selects data to archive.
*
* Data can be chosen using the age of the data or a MongoDB query.
* DATE selects documents to archive based on a date.
* CUSTOM selects documents to archive based on a custom JSON query. MongoDB Cloud doesn't support CUSTOM when "collectionType": "TIMESERIES"
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType getType() {
return null;
}
/**
* @return a {@link Builder} of {@link CriteriaView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CriteriaView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String dateField;
org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat dateFormat;
java.lang.Number expireAfterDays;
java.lang.String query;
org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType type;
/**
* Sets the value of {@link CriteriaView#getDateField}
* @param dateField Indexed database parameter that stores the date that determines when data moves to the online archive.
* MongoDB Cloud archives the data when the current date exceeds the date in this database parameter plus the number of days specified through the expireAfterDays parameter. Set this parameter when you set "criteria.type" : "DATE".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dateField(java.lang.String dateField) {
this.dateField = dateField;
return this;
}
/**
* Sets the value of {@link CriteriaView#getDateFormat}
* @param dateFormat Syntax used to write the date after which data moves to the online archive.
* Date can be expressed as ISO 8601 or Epoch timestamps. The Epoch timestamp can be expressed as nanoseconds, milliseconds, or seconds. Set this parameter when "criteria.type" : "DATE". You must set "criteria.type" : "DATE" if "collectionType": "TIMESERIES".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dateFormat(org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat dateFormat) {
this.dateFormat = dateFormat;
return this;
}
/**
* Sets the value of {@link CriteriaView#getExpireAfterDays}
* @param expireAfterDays Number of days after the value in the criteria.dateField when MongoDB Cloud archives data in the specified cluster. Set this parameter when you set "criteria.type" : "DATE".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder expireAfterDays(java.lang.Number expireAfterDays) {
this.expireAfterDays = expireAfterDays;
return this;
}
/**
* Sets the value of {@link CriteriaView#getQuery}
* @param query MongoDB find query that selects documents to archive.
* The specified query follows the syntax of the db.collection.find(query) command. This query can't use the empty document ({}) to return all documents. Set this parameter when "criteria.type" : "CUSTOM".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder query(java.lang.String query) {
this.query = query;
return this;
}
/**
* Sets the value of {@link CriteriaView#getType}
* @param type Means by which MongoDB Cloud selects data to archive.
* Data can be chosen using the age of the data or a MongoDB query.
* DATE selects documents to archive based on a date.
* CUSTOM selects documents to archive based on a custom JSON query. MongoDB Cloud doesn't support CUSTOM when "collectionType": "TIMESERIES"
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType type) {
this.type = type;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CriteriaView}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CriteriaView build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CriteriaView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CriteriaView {
private final java.lang.String dateField;
private final org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat dateFormat;
private final java.lang.Number expireAfterDays;
private final java.lang.String query;
private final org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType type;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.dateField = software.amazon.jsii.Kernel.get(this, "dateField", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.dateFormat = software.amazon.jsii.Kernel.get(this, "dateFormat", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat.class));
this.expireAfterDays = software.amazon.jsii.Kernel.get(this, "expireAfterDays", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.query = software.amazon.jsii.Kernel.get(this, "query", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.dateField = builder.dateField;
this.dateFormat = builder.dateFormat;
this.expireAfterDays = builder.expireAfterDays;
this.query = builder.query;
this.type = builder.type;
}
@Override
public final java.lang.String getDateField() {
return this.dateField;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewDateFormat getDateFormat() {
return this.dateFormat;
}
@Override
public final java.lang.Number getExpireAfterDays() {
return this.expireAfterDays;
}
@Override
public final java.lang.String getQuery() {
return this.query;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CriteriaViewType getType() {
return this.type;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDateField() != null) {
data.set("dateField", om.valueToTree(this.getDateField()));
}
if (this.getDateFormat() != null) {
data.set("dateFormat", om.valueToTree(this.getDateFormat()));
}
if (this.getExpireAfterDays() != null) {
data.set("expireAfterDays", om.valueToTree(this.getExpireAfterDays()));
}
if (this.getQuery() != null) {
data.set("query", om.valueToTree(this.getQuery()));
}
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CriteriaView"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CriteriaView.Jsii$Proxy that = (CriteriaView.Jsii$Proxy) o;
if (this.dateField != null ? !this.dateField.equals(that.dateField) : that.dateField != null) return false;
if (this.dateFormat != null ? !this.dateFormat.equals(that.dateFormat) : that.dateFormat != null) return false;
if (this.expireAfterDays != null ? !this.expireAfterDays.equals(that.expireAfterDays) : that.expireAfterDays != null) return false;
if (this.query != null ? !this.query.equals(that.query) : that.query != null) return false;
return this.type != null ? this.type.equals(that.type) : that.type == null;
}
@Override
public final int hashCode() {
int result = this.dateField != null ? this.dateField.hashCode() : 0;
result = 31 * result + (this.dateFormat != null ? this.dateFormat.hashCode() : 0);
result = 31 * result + (this.expireAfterDays != null ? this.expireAfterDays.hashCode() : 0);
result = 31 * result + (this.query != null ? this.query.hashCode() : 0);
result = 31 * result + (this.type != null ? this.type.hashCode() : 0);
return result;
}
}
}