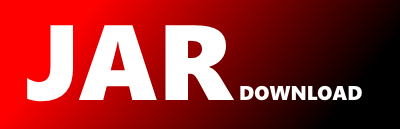
org.mongodb.awscdk.resources.mongodbatlas.ApiAtlasNdsUserToDnMappingView Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.865Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.ApiAtlasNdsUserToDnMappingView")
@software.amazon.jsii.Jsii.Proxy(ApiAtlasNdsUserToDnMappingView.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ApiAtlasNdsUserToDnMappingView extends software.amazon.jsii.JsiiSerializable {
/**
* Lightweight Directory Access Protocol (LDAP) query template that inserts the LDAP name that the regular expression matches into an LDAP query Uniform Resource Identifier (URI).
*
* The formatting for the query must conform to RFC 4515 and RFC 4516.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getLdapQuery() {
return null;
}
/**
* Regular expression that MongoDB Cloud uses to match against the provided Lightweight Directory Access Protocol (LDAP) username.
*
* Each parenthesis-enclosed section represents a regular expression capture group that the substitution or ldapQuery
template uses.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getMatch() {
return null;
}
/**
* Lightweight Directory Access Protocol (LDAP) Distinguished Name (DN) template that converts the LDAP username that matches regular expression in the match parameter into an LDAP Distinguished Name (DN).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSubstitution() {
return null;
}
/**
* @return a {@link Builder} of {@link ApiAtlasNdsUserToDnMappingView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ApiAtlasNdsUserToDnMappingView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String ldapQuery;
java.lang.String match;
java.lang.String substitution;
/**
* Sets the value of {@link ApiAtlasNdsUserToDnMappingView#getLdapQuery}
* @param ldapQuery Lightweight Directory Access Protocol (LDAP) query template that inserts the LDAP name that the regular expression matches into an LDAP query Uniform Resource Identifier (URI).
* The formatting for the query must conform to RFC 4515 and RFC 4516.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ldapQuery(java.lang.String ldapQuery) {
this.ldapQuery = ldapQuery;
return this;
}
/**
* Sets the value of {@link ApiAtlasNdsUserToDnMappingView#getMatch}
* @param match Regular expression that MongoDB Cloud uses to match against the provided Lightweight Directory Access Protocol (LDAP) username.
* Each parenthesis-enclosed section represents a regular expression capture group that the substitution or ldapQuery
template uses.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder match(java.lang.String match) {
this.match = match;
return this;
}
/**
* Sets the value of {@link ApiAtlasNdsUserToDnMappingView#getSubstitution}
* @param substitution Lightweight Directory Access Protocol (LDAP) Distinguished Name (DN) template that converts the LDAP username that matches regular expression in the match parameter into an LDAP Distinguished Name (DN).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder substitution(java.lang.String substitution) {
this.substitution = substitution;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ApiAtlasNdsUserToDnMappingView}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ApiAtlasNdsUserToDnMappingView build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ApiAtlasNdsUserToDnMappingView}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ApiAtlasNdsUserToDnMappingView {
private final java.lang.String ldapQuery;
private final java.lang.String match;
private final java.lang.String substitution;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.ldapQuery = software.amazon.jsii.Kernel.get(this, "ldapQuery", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.match = software.amazon.jsii.Kernel.get(this, "match", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.substitution = software.amazon.jsii.Kernel.get(this, "substitution", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.ldapQuery = builder.ldapQuery;
this.match = builder.match;
this.substitution = builder.substitution;
}
@Override
public final java.lang.String getLdapQuery() {
return this.ldapQuery;
}
@Override
public final java.lang.String getMatch() {
return this.match;
}
@Override
public final java.lang.String getSubstitution() {
return this.substitution;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getLdapQuery() != null) {
data.set("ldapQuery", om.valueToTree(this.getLdapQuery()));
}
if (this.getMatch() != null) {
data.set("match", om.valueToTree(this.getMatch()));
}
if (this.getSubstitution() != null) {
data.set("substitution", om.valueToTree(this.getSubstitution()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.ApiAtlasNdsUserToDnMappingView"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ApiAtlasNdsUserToDnMappingView.Jsii$Proxy that = (ApiAtlasNdsUserToDnMappingView.Jsii$Proxy) o;
if (this.ldapQuery != null ? !this.ldapQuery.equals(that.ldapQuery) : that.ldapQuery != null) return false;
if (this.match != null ? !this.match.equals(that.match) : that.match != null) return false;
return this.substitution != null ? this.substitution.equals(that.substitution) : that.substitution == null;
}
@Override
public final int hashCode() {
int result = this.ldapQuery != null ? this.ldapQuery.hashCode() : 0;
result = 31 * result + (this.match != null ? this.match.hashCode() : 0);
result = 31 * result + (this.substitution != null ? this.substitution.hashCode() : 0);
return result;
}
}
}