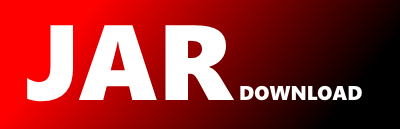
org.mongodb.awscdk.resources.mongodbatlas.AwsKmsConfig Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Specifies AWS KMS configuration details and whether Encryption at Rest is enabled for an Atlas project.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.888Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.AwsKmsConfig")
@software.amazon.jsii.Jsii.Proxy(AwsKmsConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface AwsKmsConfig extends software.amazon.jsii.JsiiSerializable {
/**
* The AWS customer master key used to encrypt and decrypt the MongoDB master keys.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCustomerMasterKeyId() {
return null;
}
/**
* Specifies whether Encryption at Rest is enabled for an Atlas project.
*
* To disable Encryption at Rest, pass only this parameter with a value of false. When you disable Encryption at Rest, Atlas also removes the configuration details.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEnabled() {
return null;
}
/**
* The AWS region in which the AWS customer master key exists.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRegion() {
return null;
}
/**
* ID of an AWS IAM role authorized to manage an AWS customer master key.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRoleId() {
return null;
}
/**
* @return a {@link Builder} of {@link AwsKmsConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AwsKmsConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String customerMasterKeyId;
java.lang.Boolean enabled;
java.lang.String region;
java.lang.String roleId;
/**
* Sets the value of {@link AwsKmsConfig#getCustomerMasterKeyId}
* @param customerMasterKeyId The AWS customer master key used to encrypt and decrypt the MongoDB master keys.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder customerMasterKeyId(java.lang.String customerMasterKeyId) {
this.customerMasterKeyId = customerMasterKeyId;
return this;
}
/**
* Sets the value of {@link AwsKmsConfig#getEnabled}
* @param enabled Specifies whether Encryption at Rest is enabled for an Atlas project.
* To disable Encryption at Rest, pass only this parameter with a value of false. When you disable Encryption at Rest, Atlas also removes the configuration details.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link AwsKmsConfig#getRegion}
* @param region The AWS region in which the AWS customer master key exists.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of {@link AwsKmsConfig#getRoleId}
* @param roleId ID of an AWS IAM role authorized to manage an AWS customer master key.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roleId(java.lang.String roleId) {
this.roleId = roleId;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AwsKmsConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public AwsKmsConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link AwsKmsConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements AwsKmsConfig {
private final java.lang.String customerMasterKeyId;
private final java.lang.Boolean enabled;
private final java.lang.String region;
private final java.lang.String roleId;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.customerMasterKeyId = software.amazon.jsii.Kernel.get(this, "customerMasterKeyId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.enabled = software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.region = software.amazon.jsii.Kernel.get(this, "region", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.roleId = software.amazon.jsii.Kernel.get(this, "roleId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.customerMasterKeyId = builder.customerMasterKeyId;
this.enabled = builder.enabled;
this.region = builder.region;
this.roleId = builder.roleId;
}
@Override
public final java.lang.String getCustomerMasterKeyId() {
return this.customerMasterKeyId;
}
@Override
public final java.lang.Boolean getEnabled() {
return this.enabled;
}
@Override
public final java.lang.String getRegion() {
return this.region;
}
@Override
public final java.lang.String getRoleId() {
return this.roleId;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getCustomerMasterKeyId() != null) {
data.set("customerMasterKeyId", om.valueToTree(this.getCustomerMasterKeyId()));
}
if (this.getEnabled() != null) {
data.set("enabled", om.valueToTree(this.getEnabled()));
}
if (this.getRegion() != null) {
data.set("region", om.valueToTree(this.getRegion()));
}
if (this.getRoleId() != null) {
data.set("roleId", om.valueToTree(this.getRoleId()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.AwsKmsConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AwsKmsConfig.Jsii$Proxy that = (AwsKmsConfig.Jsii$Proxy) o;
if (this.customerMasterKeyId != null ? !this.customerMasterKeyId.equals(that.customerMasterKeyId) : that.customerMasterKeyId != null) return false;
if (this.enabled != null ? !this.enabled.equals(that.enabled) : that.enabled != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
return this.roleId != null ? this.roleId.equals(that.roleId) : that.roleId == null;
}
@Override
public final int hashCode() {
int result = this.customerMasterKeyId != null ? this.customerMasterKeyId.hashCode() : 0;
result = 31 * result + (this.enabled != null ? this.enabled.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.roleId != null ? this.roleId.hashCode() : 0);
return result;
}
}
}