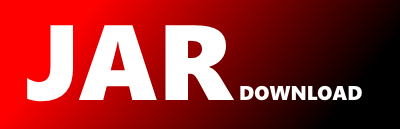
org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::CloudBackupSchedule
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.900Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnCloudBackupSchedule")
public class CfnCloudBackupSchedule extends software.amazon.awscdk.CfnResource {
protected CfnCloudBackupSchedule(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnCloudBackupSchedule(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::CloudBackupSchedule
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnCloudBackupSchedule(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupScheduleProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Attribute MongoDB::Atlas::CloudBackupSchedule.ClusterId
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrClusterId() {
return software.amazon.jsii.Kernel.get(this, "attrClusterId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute MongoDB::Atlas::CloudBackupSchedule.NextSnapshot
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrNextSnapshot() {
return software.amazon.jsii.Kernel.get(this, "attrNextSnapshot", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupScheduleProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupScheduleProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupScheduleProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupScheduleProps.Builder();
}
/**
* Flag that indicates whether automatic export of cloud backup snapshots to the AWS bucket is enabled.
*
* @return {@code this}
* @param autoExportEnabled Flag that indicates whether automatic export of cloud backup snapshots to the AWS bucket is enabled. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder autoExportEnabled(final java.lang.Boolean autoExportEnabled) {
this.props.autoExportEnabled(autoExportEnabled);
return this;
}
/**
* The name of the Atlas cluster that contains the snapshots you want to retrieve.
*
* @return {@code this}
* @param clusterName The name of the Atlas cluster that contains the snapshots you want to retrieve. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder clusterName(final java.lang.String clusterName) {
this.props.clusterName(clusterName);
return this;
}
/**
* List that contains a document for each copy setting item in the desired backup policy.
*
* @return {@code this}
* @param copySettings List that contains a document for each copy setting item in the desired backup policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder copySettings(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ApiAtlasDiskBackupCopySettingView> copySettings) {
this.props.copySettings(copySettings);
return this;
}
/**
* List that contains a document for each deleted copy setting whose backup copies you want to delete.
*
* @return {@code this}
* @param deleteCopiedBackups List that contains a document for each deleted copy setting whose backup copies you want to delete. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deleteCopiedBackups(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ApiDeleteCopiedBackupsView> deleteCopiedBackups) {
this.props.deleteCopiedBackups(deleteCopiedBackups);
return this;
}
/**
* Policy for automatically exporting cloud backup snapshots.
*
* @return {@code this}
* @param export Policy for automatically exporting cloud backup snapshots. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder export(final org.mongodb.awscdk.resources.mongodbatlas.Export export) {
this.props.export(export);
return this;
}
/**
* Unique identifier of the snapshot.
*
* @return {@code this}
* @param id Unique identifier of the snapshot. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder id(final java.lang.String id) {
this.props.id(id);
return this;
}
/**
* List of one or more Uniform Resource Locators (URLs) that point to API sub-resources, related API resources, or both.
*
* RFC 5988 outlines these relationships.
*
* @return {@code this}
* @param links List of one or more Uniform Resource Locators (URLs) that point to API sub-resources, related API resources, or both. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder links(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.Link> links) {
this.props.links(links);
return this;
}
/**
* Rules set for this backup schedule.
*
* @return {@code this}
* @param policies Rules set for this backup schedule. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder policies(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ApiPolicyView> policies) {
this.props.policies(policies);
return this;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*
* @return {@code this}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* The unique identifier of the project for the Atlas cluster.
*
* @return {@code this}
* @param projectId The unique identifier of the project for the Atlas cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* UTC Hour of day between 0 and 23 representing which hour of the day that Atlas takes a snapshot.
*
* @return {@code this}
* @param referenceHourOfDay UTC Hour of day between 0 and 23 representing which hour of the day that Atlas takes a snapshot. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder referenceHourOfDay(final java.lang.Number referenceHourOfDay) {
this.props.referenceHourOfDay(referenceHourOfDay);
return this;
}
/**
* UTC Minute of day between 0 and 59 representing which minute of the referenceHourOfDay that Atlas takes the snapshot.
*
* @return {@code this}
* @param referenceMinuteOfHour UTC Minute of day between 0 and 59 representing which minute of the referenceHourOfDay that Atlas takes the snapshot. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder referenceMinuteOfHour(final java.lang.Number referenceMinuteOfHour) {
this.props.referenceMinuteOfHour(referenceMinuteOfHour);
return this;
}
/**
* Number of days back in time you can restore to with Continuous Cloud Backup accuracy.
*
* Must be a positive, non-zero integer.
*
* @return {@code this}
* @param restoreWindowDays Number of days back in time you can restore to with Continuous Cloud Backup accuracy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder restoreWindowDays(final java.lang.Number restoreWindowDays) {
this.props.restoreWindowDays(restoreWindowDays);
return this;
}
/**
* Flag indicating if updates to retention in the backup policy were applied to snapshots that Atlas took earlier.
*
* @return {@code this}
* @param updateSnapshots Flag indicating if updates to retention in the backup policy were applied to snapshots that Atlas took earlier. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder updateSnapshots(final java.lang.Boolean updateSnapshots) {
this.props.updateSnapshots(updateSnapshots);
return this;
}
/**
* Specify true to use organization and project names instead of organization and project UUIDs in the path for the metadata files that Atlas uploads to your S3 bucket after it finishes exporting the snapshots.
*
* @return {@code this}
* @param useOrgAndGroupNamesInExportPrefix Specify true to use organization and project names instead of organization and project UUIDs in the path for the metadata files that Atlas uploads to your S3 bucket after it finishes exporting the snapshots. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder useOrgAndGroupNamesInExportPrefix(final java.lang.Boolean useOrgAndGroupNamesInExportPrefix) {
this.props.useOrgAndGroupNamesInExportPrefix(useOrgAndGroupNamesInExportPrefix);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnCloudBackupSchedule(
this.scope,
this.id,
this.props.build()
);
}
}
}