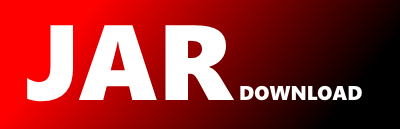
org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::DatabaseUser
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.921Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnDatabaseUser")
public class CfnDatabaseUser extends software.amazon.awscdk.CfnResource {
protected CfnDatabaseUser(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnDatabaseUser(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::DatabaseUser
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnDatabaseUser(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Attribute MongoDB::Atlas::DatabaseUser.UserCFNIdentifier
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrUserCFNIdentifier() {
return software.amazon.jsii.Kernel.get(this, "attrUserCFNIdentifier", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps.Builder();
}
/**
* MongoDB database against which the MongoDB database user authenticates.
*
* MongoDB database users must provide both a username and authentication database to log into MongoDB. Default value is admin
.
*
* @return {@code this}
* @param databaseName MongoDB database against which the MongoDB database user authenticates. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder databaseName(final java.lang.String databaseName) {
this.props.databaseName(databaseName);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies your Atlas Project.
*
* @return {@code this}
* @param projectId Unique 24-hexadecimal digit string that identifies your Atlas Project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* List that provides the pairings of one role with one applicable database.
*
* @return {@code this}
* @param roles List that provides the pairings of one role with one applicable database. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder roles(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.RoleDefinition> roles) {
this.props.roles(roles);
return this;
}
/**
* Human-readable label that represents the user that authenticates to MongoDB.
*
* The format of this label depends on the method of authentication. This will be USER_ARN or ROLE_ARN if AWSIAMType is USER or ROLE. Refer https://www.mongodb.com/docs/atlas/reference/api-resources-spec/#tag/Database-Users/operation/createDatabaseUser for details.
*
* @return {@code this}
* @param username Human-readable label that represents the user that authenticates to MongoDB. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder username(final java.lang.String username) {
this.props.username(username);
return this;
}
/**
* Human-readable label that indicates whether the new database user authenticates with the Amazon Web Services (AWS) Identity and Access Management (IAM) credentials associated with the user or the user's role.
*
* Default value is NONE
.
*
* @return {@code this}
* @param awsiamType Human-readable label that indicates whether the new database user authenticates with the Amazon Web Services (AWS) Identity and Access Management (IAM) credentials associated with the user or the user's role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder awsiamType(final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType awsiamType) {
this.props.awsiamType(awsiamType);
return this;
}
/**
* Date and time when MongoDB Cloud deletes the user.
*
* This parameter expresses its value in the ISO 8601 timestamp format in UTC and can include the time zone designation. You must specify a future date that falls within one week of making the Application Programming Interface (API) request.
*
* @return {@code this}
* @param deleteAfterDate Date and time when MongoDB Cloud deletes the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deleteAfterDate(final java.lang.String deleteAfterDate) {
this.props.deleteAfterDate(deleteAfterDate);
return this;
}
/**
* List that contains the key-value pairs for tagging and categorizing the MongoDB database user.
*
* The labels that you define do not appear in the console.
*
* @return {@code this}
* @param labels List that contains the key-value pairs for tagging and categorizing the MongoDB database user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.LabelDefinition> labels) {
this.props.labels(labels);
return this;
}
/**
* Method by which the provided username is authenticated.
*
* Default value is NONE
.
*
* @return {@code this}
* @param ldapAuthType Method by which the provided username is authenticated. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ldapAuthType(final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType ldapAuthType) {
this.props.ldapAuthType(ldapAuthType);
return this;
}
/**
* The user’s password.
*
* This field is not included in the entity returned from the server.
*
* @return {@code this}
* @param password The user’s password. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder password(final java.lang.String password) {
this.props.password(password);
return this;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default
is used.
*
* @return {@code this}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default
is used. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* List that contains clusters and MongoDB Atlas Data Lakes that this database user can access.
*
* If omitted, MongoDB Cloud grants the database user access to all the clusters and MongoDB Atlas Data Lakes in the project.
*
* @return {@code this}
* @param scopes List that contains clusters and MongoDB Atlas Data Lakes that this database user can access. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scopes(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ScopeDefinition> scopes) {
this.props.scopes(scopes);
return this;
}
/**
* Method that briefs who owns the certificate provided.
*
* Default value is NONE
.
*
* @return {@code this}
* @param x509Type Method that briefs who owns the certificate provided. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder x509Type(final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type x509Type) {
this.props.x509Type(x509Type);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUser(
this.scope,
this.id,
this.props.build()
);
}
}
}