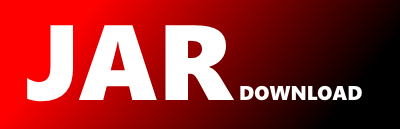
org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserProps Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Returns, adds, edits, and removes database users.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.922Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnDatabaseUserProps")
@software.amazon.jsii.Jsii.Proxy(CfnDatabaseUserProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnDatabaseUserProps extends software.amazon.jsii.JsiiSerializable {
/**
* MongoDB database against which the MongoDB database user authenticates.
*
* MongoDB database users must provide both a username and authentication database to log into MongoDB. Default value is admin
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDatabaseName();
/**
* Unique 24-hexadecimal digit string that identifies your Atlas Project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getProjectId();
/**
* List that provides the pairings of one role with one applicable database.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getRoles();
/**
* Human-readable label that represents the user that authenticates to MongoDB.
*
* The format of this label depends on the method of authentication. This will be USER_ARN or ROLE_ARN if AWSIAMType is USER or ROLE. Refer https://www.mongodb.com/docs/atlas/reference/api-resources-spec/#tag/Database-Users/operation/createDatabaseUser for details.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getUsername();
/**
* Human-readable label that indicates whether the new database user authenticates with the Amazon Web Services (AWS) Identity and Access Management (IAM) credentials associated with the user or the user's role.
*
* Default value is NONE
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType getAwsiamType() {
return null;
}
/**
* Date and time when MongoDB Cloud deletes the user.
*
* This parameter expresses its value in the ISO 8601 timestamp format in UTC and can include the time zone designation. You must specify a future date that falls within one week of making the Application Programming Interface (API) request.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDeleteAfterDate() {
return null;
}
/**
* List that contains the key-value pairs for tagging and categorizing the MongoDB database user.
*
* The labels that you define do not appear in the console.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getLabels() {
return null;
}
/**
* Method by which the provided username is authenticated.
*
* Default value is NONE
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType getLdapAuthType() {
return null;
}
/**
* The user’s password.
*
* This field is not included in the entity returned from the server.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getPassword() {
return null;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default
is used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
* List that contains clusters and MongoDB Atlas Data Lakes that this database user can access.
*
* If omitted, MongoDB Cloud grants the database user access to all the clusters and MongoDB Atlas Data Lakes in the project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getScopes() {
return null;
}
/**
* Method that briefs who owns the certificate provided.
*
* Default value is NONE
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type getX509Type() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnDatabaseUserProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnDatabaseUserProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String databaseName;
java.lang.String projectId;
java.util.List roles;
java.lang.String username;
org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType awsiamType;
java.lang.String deleteAfterDate;
java.util.List labels;
org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType ldapAuthType;
java.lang.String password;
java.lang.String profile;
java.util.List scopes;
org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type x509Type;
/**
* Sets the value of {@link CfnDatabaseUserProps#getDatabaseName}
* @param databaseName MongoDB database against which the MongoDB database user authenticates. This parameter is required.
* MongoDB database users must provide both a username and authentication database to log into MongoDB. Default value is admin
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder databaseName(java.lang.String databaseName) {
this.databaseName = databaseName;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getProjectId}
* @param projectId Unique 24-hexadecimal digit string that identifies your Atlas Project. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getRoles}
* @param roles List that provides the pairings of one role with one applicable database. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder roles(java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.RoleDefinition> roles) {
this.roles = (java.util.List)roles;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getUsername}
* @param username Human-readable label that represents the user that authenticates to MongoDB. This parameter is required.
* The format of this label depends on the method of authentication. This will be USER_ARN or ROLE_ARN if AWSIAMType is USER or ROLE. Refer https://www.mongodb.com/docs/atlas/reference/api-resources-spec/#tag/Database-Users/operation/createDatabaseUser for details.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder username(java.lang.String username) {
this.username = username;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getAwsiamType}
* @param awsiamType Human-readable label that indicates whether the new database user authenticates with the Amazon Web Services (AWS) Identity and Access Management (IAM) credentials associated with the user or the user's role.
* Default value is NONE
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder awsiamType(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType awsiamType) {
this.awsiamType = awsiamType;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getDeleteAfterDate}
* @param deleteAfterDate Date and time when MongoDB Cloud deletes the user.
* This parameter expresses its value in the ISO 8601 timestamp format in UTC and can include the time zone designation. You must specify a future date that falls within one week of making the Application Programming Interface (API) request.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deleteAfterDate(java.lang.String deleteAfterDate) {
this.deleteAfterDate = deleteAfterDate;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getLabels}
* @param labels List that contains the key-value pairs for tagging and categorizing the MongoDB database user.
* The labels that you define do not appear in the console.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder labels(java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.LabelDefinition> labels) {
this.labels = (java.util.List)labels;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getLdapAuthType}
* @param ldapAuthType Method by which the provided username is authenticated.
* Default value is NONE
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ldapAuthType(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType ldapAuthType) {
this.ldapAuthType = ldapAuthType;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getPassword}
* @param password The user’s password.
* This field is not included in the entity returned from the server.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder password(java.lang.String password) {
this.password = password;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getProfile}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default
is used.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getScopes}
* @param scopes List that contains clusters and MongoDB Atlas Data Lakes that this database user can access.
* If omitted, MongoDB Cloud grants the database user access to all the clusters and MongoDB Atlas Data Lakes in the project.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder scopes(java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ScopeDefinition> scopes) {
this.scopes = (java.util.List)scopes;
return this;
}
/**
* Sets the value of {@link CfnDatabaseUserProps#getX509Type}
* @param x509Type Method that briefs who owns the certificate provided.
* Default value is NONE
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder x509Type(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type x509Type) {
this.x509Type = x509Type;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnDatabaseUserProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnDatabaseUserProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnDatabaseUserProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnDatabaseUserProps {
private final java.lang.String databaseName;
private final java.lang.String projectId;
private final java.util.List roles;
private final java.lang.String username;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType awsiamType;
private final java.lang.String deleteAfterDate;
private final java.util.List labels;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType ldapAuthType;
private final java.lang.String password;
private final java.lang.String profile;
private final java.util.List scopes;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type x509Type;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.databaseName = software.amazon.jsii.Kernel.get(this, "databaseName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.projectId = software.amazon.jsii.Kernel.get(this, "projectId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.roles = software.amazon.jsii.Kernel.get(this, "roles", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.RoleDefinition.class)));
this.username = software.amazon.jsii.Kernel.get(this, "username", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.awsiamType = software.amazon.jsii.Kernel.get(this, "awsiamType", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType.class));
this.deleteAfterDate = software.amazon.jsii.Kernel.get(this, "deleteAfterDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.labels = software.amazon.jsii.Kernel.get(this, "labels", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.LabelDefinition.class)));
this.ldapAuthType = software.amazon.jsii.Kernel.get(this, "ldapAuthType", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType.class));
this.password = software.amazon.jsii.Kernel.get(this, "password", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.scopes = software.amazon.jsii.Kernel.get(this, "scopes", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.ScopeDefinition.class)));
this.x509Type = software.amazon.jsii.Kernel.get(this, "x509Type", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.databaseName = java.util.Objects.requireNonNull(builder.databaseName, "databaseName is required");
this.projectId = java.util.Objects.requireNonNull(builder.projectId, "projectId is required");
this.roles = (java.util.List)java.util.Objects.requireNonNull(builder.roles, "roles is required");
this.username = java.util.Objects.requireNonNull(builder.username, "username is required");
this.awsiamType = builder.awsiamType;
this.deleteAfterDate = builder.deleteAfterDate;
this.labels = (java.util.List)builder.labels;
this.ldapAuthType = builder.ldapAuthType;
this.password = builder.password;
this.profile = builder.profile;
this.scopes = (java.util.List)builder.scopes;
this.x509Type = builder.x509Type;
}
@Override
public final java.lang.String getDatabaseName() {
return this.databaseName;
}
@Override
public final java.lang.String getProjectId() {
return this.projectId;
}
@Override
public final java.util.List getRoles() {
return this.roles;
}
@Override
public final java.lang.String getUsername() {
return this.username;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsAwsiamType getAwsiamType() {
return this.awsiamType;
}
@Override
public final java.lang.String getDeleteAfterDate() {
return this.deleteAfterDate;
}
@Override
public final java.util.List getLabels() {
return this.labels;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsLdapAuthType getLdapAuthType() {
return this.ldapAuthType;
}
@Override
public final java.lang.String getPassword() {
return this.password;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final java.util.List getScopes() {
return this.scopes;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CfnDatabaseUserPropsX509Type getX509Type() {
return this.x509Type;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("databaseName", om.valueToTree(this.getDatabaseName()));
data.set("projectId", om.valueToTree(this.getProjectId()));
data.set("roles", om.valueToTree(this.getRoles()));
data.set("username", om.valueToTree(this.getUsername()));
if (this.getAwsiamType() != null) {
data.set("awsiamType", om.valueToTree(this.getAwsiamType()));
}
if (this.getDeleteAfterDate() != null) {
data.set("deleteAfterDate", om.valueToTree(this.getDeleteAfterDate()));
}
if (this.getLabels() != null) {
data.set("labels", om.valueToTree(this.getLabels()));
}
if (this.getLdapAuthType() != null) {
data.set("ldapAuthType", om.valueToTree(this.getLdapAuthType()));
}
if (this.getPassword() != null) {
data.set("password", om.valueToTree(this.getPassword()));
}
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getScopes() != null) {
data.set("scopes", om.valueToTree(this.getScopes()));
}
if (this.getX509Type() != null) {
data.set("x509Type", om.valueToTree(this.getX509Type()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CfnDatabaseUserProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnDatabaseUserProps.Jsii$Proxy that = (CfnDatabaseUserProps.Jsii$Proxy) o;
if (!databaseName.equals(that.databaseName)) return false;
if (!projectId.equals(that.projectId)) return false;
if (!roles.equals(that.roles)) return false;
if (!username.equals(that.username)) return false;
if (this.awsiamType != null ? !this.awsiamType.equals(that.awsiamType) : that.awsiamType != null) return false;
if (this.deleteAfterDate != null ? !this.deleteAfterDate.equals(that.deleteAfterDate) : that.deleteAfterDate != null) return false;
if (this.labels != null ? !this.labels.equals(that.labels) : that.labels != null) return false;
if (this.ldapAuthType != null ? !this.ldapAuthType.equals(that.ldapAuthType) : that.ldapAuthType != null) return false;
if (this.password != null ? !this.password.equals(that.password) : that.password != null) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
if (this.scopes != null ? !this.scopes.equals(that.scopes) : that.scopes != null) return false;
return this.x509Type != null ? this.x509Type.equals(that.x509Type) : that.x509Type == null;
}
@Override
public final int hashCode() {
int result = this.databaseName.hashCode();
result = 31 * result + (this.projectId.hashCode());
result = 31 * result + (this.roles.hashCode());
result = 31 * result + (this.username.hashCode());
result = 31 * result + (this.awsiamType != null ? this.awsiamType.hashCode() : 0);
result = 31 * result + (this.deleteAfterDate != null ? this.deleteAfterDate.hashCode() : 0);
result = 31 * result + (this.labels != null ? this.labels.hashCode() : 0);
result = 31 * result + (this.ldapAuthType != null ? this.ldapAuthType.hashCode() : 0);
result = 31 * result + (this.password != null ? this.password.hashCode() : 0);
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.scopes != null ? this.scopes.hashCode() : 0);
result = 31 * result + (this.x509Type != null ? this.x509Type.hashCode() : 0);
return result;
}
}
}