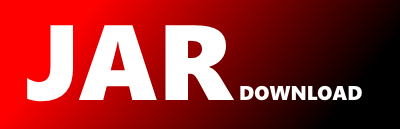
org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::FederatedDatabaseInstance
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.929Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnFederatedDatabaseInstance")
public class CfnFederatedDatabaseInstance extends software.amazon.awscdk.CfnResource {
protected CfnFederatedDatabaseInstance(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnFederatedDatabaseInstance(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::FederatedDatabaseInstance
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnFederatedDatabaseInstance(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstanceProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Attribute MongoDB::Atlas::FederatedDatabaseInstance.HostNames
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getAttrHostNames() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "attrHostNames", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class))));
}
/**
* Attribute MongoDB::Atlas::FederatedDatabaseInstance.State
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrState() {
return software.amazon.jsii.Kernel.get(this, "attrState", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstanceProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstanceProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstanceProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstanceProps.Builder();
}
/**
* Unique 24-hexadecimal digit string that identifies your project.
*
* @return {@code this}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* Human-readable label that identifies the data federation.
*
* @return {@code this}
* @param tenantName Human-readable label that identifies the data federation. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tenantName(final java.lang.String tenantName) {
this.props.tenantName(tenantName);
return this;
}
/**
* Cloud provider linked to this data lake.
*
* @return {@code this}
* @param cloudProviderConfig Cloud provider linked to this data lake. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudProviderConfig(final org.mongodb.awscdk.resources.mongodbatlas.CloudProviderConfig cloudProviderConfig) {
this.props.cloudProviderConfig(cloudProviderConfig);
return this;
}
/**
* Information about the cloud provider region to which the data lake routes client connections.
*
* MongoDB Cloud supports AWS only.
*
* @return {@code this}
* @param dataProcessRegion Information about the cloud provider region to which the data lake routes client connections. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dataProcessRegion(final org.mongodb.awscdk.resources.mongodbatlas.DataProcessRegion dataProcessRegion) {
this.props.dataProcessRegion(dataProcessRegion);
return this;
}
/**
* The profile is defined in AWS Secret manager.
*
* See Secret Manager Profile setup.
*
* @return {@code this}
* @param profile The profile is defined in AWS Secret manager. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* Flag that indicates whether this request should check if the requesting IAM role can read from the S3 bucket.
*
* AWS checks if the role can list the objects in the bucket before writing to it. Some IAM roles only need write permissions. This flag allows you to skip that check.
*
* @return {@code this}
* @param skipRoleValidation Flag that indicates whether this request should check if the requesting IAM role can read from the S3 bucket. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder skipRoleValidation(final java.lang.Boolean skipRoleValidation) {
this.props.skipRoleValidation(skipRoleValidation);
return this;
}
/**
* Configuration information for each data store and its mapping to MongoDB Cloud databases.
*
* @return {@code this}
* @param storage Configuration information for each data store and its mapping to MongoDB Cloud databases. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder storage(final org.mongodb.awscdk.resources.mongodbatlas.Storage storage) {
this.props.storage(storage);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedDatabaseInstance(
this.scope,
this.id,
this.props.build()
);
}
}
}