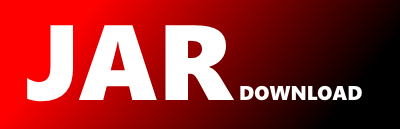
org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::FederatedQueryLimit
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.931Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnFederatedQueryLimit")
public class CfnFederatedQueryLimit extends software.amazon.awscdk.CfnResource {
protected CfnFederatedQueryLimit(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnFederatedQueryLimit(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::FederatedQueryLimit
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnFederatedQueryLimit(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Attribute MongoDB::Atlas::FederatedQueryLimit.CurrentUsage
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrCurrentUsage() {
return software.amazon.jsii.Kernel.get(this, "attrCurrentUsage", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute MongoDB::Atlas::FederatedQueryLimit.DefaultLimit
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrDefaultLimit() {
return software.amazon.jsii.Kernel.get(this, "attrDefaultLimit", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute MongoDB::Atlas::FederatedQueryLimit.LastModifiedDate
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrLastModifiedDate() {
return software.amazon.jsii.Kernel.get(this, "attrLastModifiedDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Attribute MongoDB::Atlas::FederatedQueryLimit.MaximumLimit
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrMaximumLimit() {
return software.amazon.jsii.Kernel.get(this, "attrMaximumLimit", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitProps.Builder();
}
/**
* Human-readable label that identifies this data federation instance limit.
*
* @return {@code this}
* @param limitName Human-readable label that identifies this data federation instance limit. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder limitName(final org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimitPropsLimitName limitName) {
this.props.limitName(limitName);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies your project.
*
* @return {@code this}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* Human-readable label that identifies the data federated database instance to which the query limit applies.
*
* @return {@code this}
* @param tenantName Human-readable label that identifies the data federated database instance to which the query limit applies. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tenantName(final java.lang.String tenantName) {
this.props.tenantName(tenantName);
return this;
}
/**
* Amount to set the limit to.
*
* @return {@code this}
* @param value Amount to set the limit to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(final java.lang.String value) {
this.props.value(value);
return this;
}
/**
* Only used for Data Federation limits.
*
* Action to take when the usage limit is exceeded. If limit span is set to QUERY, this is ignored because MongoDB Cloud stops the query when it exceeds the usage limit. "enum" : [ "BLOCK", "BLOCK_AND_KILL" ]
*
* @return {@code this}
* @param overrunPolicy Only used for Data Federation limits. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder overrunPolicy(final java.lang.String overrunPolicy) {
this.props.overrunPolicy(overrunPolicy);
return this;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*
* @return {@code this}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnFederatedQueryLimit(
this.scope,
this.id,
this.props.build()
);
}
}
}