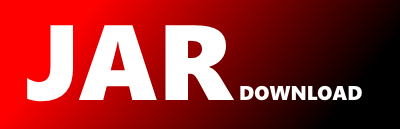
org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::LDAPConfiguration
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.936Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnLdapConfiguration")
public class CfnLdapConfiguration extends software.amazon.awscdk.CfnResource {
protected CfnLdapConfiguration(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnLdapConfiguration(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::LDAPConfiguration
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnLdapConfiguration(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps.Builder();
}
/**
* Password that MongoDB Cloud uses to authenticate the bindUsername.
*
* @return {@code this}
* @param bindPassword Password that MongoDB Cloud uses to authenticate the bindUsername. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bindPassword(final java.lang.String bindPassword) {
this.props.bindPassword(bindPassword);
return this;
}
/**
* Full Distinguished Name (DN) of the Lightweight Directory Access Protocol (LDAP) user that MongoDB Cloud uses to connect to the LDAP host.
*
* LDAP distinguished names must be formatted according to RFC 2253.
*
* @return {@code this}
* @param bindUsername Full Distinguished Name (DN) of the Lightweight Directory Access Protocol (LDAP) user that MongoDB Cloud uses to connect to the LDAP host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bindUsername(final java.lang.String bindUsername) {
this.props.bindUsername(bindUsername);
return this;
}
/**
* Human-readable label that identifies the hostname or Internet Protocol (IP) address of the Lightweight Directory Access Protocol (LDAP) host.
*
* This host must have access to the internet or have a Virtual Private Cloud (VPC) peering connection to your cluster.
*
* @return {@code this}
* @param hostname Human-readable label that identifies the hostname or Internet Protocol (IP) address of the Lightweight Directory Access Protocol (LDAP) host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder hostname(final java.lang.String hostname) {
this.props.hostname(hostname);
return this;
}
/**
* Port to which the Lightweight Directory Access Protocol (LDAP) host listens for client connections.
*
* @return {@code this}
* @param port Port to which the Lightweight Directory Access Protocol (LDAP) host listens for client connections. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(final java.lang.Number port) {
this.props.port(port);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies your project.
*
* @return {@code this}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* Flag that indicates whether users can authenticate using an Lightweight Directory Access Protocol (LDAP) host.
*
* @return {@code this}
* @param authenticationEnabled Flag that indicates whether users can authenticate using an Lightweight Directory Access Protocol (LDAP) host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authenticationEnabled(final java.lang.Boolean authenticationEnabled) {
this.props.authenticationEnabled(authenticationEnabled);
return this;
}
/**
* Flag that indicates whether users can authorize access to MongoDB Cloud resources using an Lightweight Directory Access Protocol (LDAP) host.
*
* @return {@code this}
* @param authorizationEnabled Flag that indicates whether users can authorize access to MongoDB Cloud resources using an Lightweight Directory Access Protocol (LDAP) host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authorizationEnabled(final java.lang.Boolean authorizationEnabled) {
this.props.authorizationEnabled(authorizationEnabled);
return this;
}
/**
* Lightweight Directory Access Protocol (LDAP) query template that MongoDB Cloud runs to obtain the LDAP groups associated with the authenticated user.
*
* MongoDB Cloud uses this parameter only for user authorization. Use the {USER}
placeholder in the Uniform Resource Locator (URL) to substitute the authenticated username. The query relates to the host specified with the hostname. Format this query according to RFC 4515 and RFC 4516.
*
* @return {@code this}
* @param authzQueryTemplate Lightweight Directory Access Protocol (LDAP) query template that MongoDB Cloud runs to obtain the LDAP groups associated with the authenticated user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authzQueryTemplate(final java.lang.String authzQueryTemplate) {
this.props.authzQueryTemplate(authzQueryTemplate);
return this;
}
/**
* Certificate Authority (CA) certificate that MongoDB Cloud uses to verify the identity of the Lightweight Directory Access Protocol (LDAP) host.
*
* MongoDB Cloud allows self-signed certificates. To delete an assigned value, pass an empty string: "caCertificate": ""
*
* @return {@code this}
* @param caCertificate Certificate Authority (CA) certificate that MongoDB Cloud uses to verify the identity of the Lightweight Directory Access Protocol (LDAP) host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caCertificate(final java.lang.String caCertificate) {
this.props.caCertificate(caCertificate);
return this;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*
* @return {@code this}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* The current status of the LDAP over TLS/SSL configuration.
*
* @return {@code this}
* @param status The current status of the LDAP over TLS/SSL configuration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder status(final java.lang.String status) {
this.props.status(status);
return this;
}
/**
* User-to-Distinguished Name (DN) map that MongoDB Cloud uses to transform a Lightweight Directory Access Protocol (LDAP) username into an LDAP DN.
*
* @return {@code this}
* @param userToDnMapping User-to-Distinguished Name (DN) map that MongoDB Cloud uses to transform a Lightweight Directory Access Protocol (LDAP) username into an LDAP DN. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userToDnMapping(final java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ApiAtlasNdsUserToDnMappingView> userToDnMapping) {
this.props.userToDnMapping(userToDnMapping);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfiguration(
this.scope,
this.id,
this.props.build()
);
}
}
}