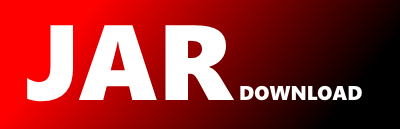
org.mongodb.awscdk.resources.mongodbatlas.CfnLdapConfigurationProps Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Returns, edits, verifies, and removes LDAP configurations.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.937Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnLdapConfigurationProps")
@software.amazon.jsii.Jsii.Proxy(CfnLdapConfigurationProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnLdapConfigurationProps extends software.amazon.jsii.JsiiSerializable {
/**
* Password that MongoDB Cloud uses to authenticate the bindUsername.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getBindPassword();
/**
* Full Distinguished Name (DN) of the Lightweight Directory Access Protocol (LDAP) user that MongoDB Cloud uses to connect to the LDAP host.
*
* LDAP distinguished names must be formatted according to RFC 2253.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getBindUsername();
/**
* Human-readable label that identifies the hostname or Internet Protocol (IP) address of the Lightweight Directory Access Protocol (LDAP) host.
*
* This host must have access to the internet or have a Virtual Private Cloud (VPC) peering connection to your cluster.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getHostname();
/**
* Port to which the Lightweight Directory Access Protocol (LDAP) host listens for client connections.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getPort();
/**
* Unique 24-hexadecimal digit string that identifies your project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getProjectId();
/**
* Flag that indicates whether users can authenticate using an Lightweight Directory Access Protocol (LDAP) host.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAuthenticationEnabled() {
return null;
}
/**
* Flag that indicates whether users can authorize access to MongoDB Cloud resources using an Lightweight Directory Access Protocol (LDAP) host.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAuthorizationEnabled() {
return null;
}
/**
* Lightweight Directory Access Protocol (LDAP) query template that MongoDB Cloud runs to obtain the LDAP groups associated with the authenticated user.
*
* MongoDB Cloud uses this parameter only for user authorization. Use the {USER}
placeholder in the Uniform Resource Locator (URL) to substitute the authenticated username. The query relates to the host specified with the hostname. Format this query according to RFC 4515 and RFC 4516.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAuthzQueryTemplate() {
return null;
}
/**
* Certificate Authority (CA) certificate that MongoDB Cloud uses to verify the identity of the Lightweight Directory Access Protocol (LDAP) host.
*
* MongoDB Cloud allows self-signed certificates. To delete an assigned value, pass an empty string: "caCertificate": ""
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCaCertificate() {
return null;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
* The current status of the LDAP over TLS/SSL configuration.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getStatus() {
return null;
}
/**
* User-to-Distinguished Name (DN) map that MongoDB Cloud uses to transform a Lightweight Directory Access Protocol (LDAP) username into an LDAP DN.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getUserToDnMapping() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnLdapConfigurationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnLdapConfigurationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String bindPassword;
java.lang.String bindUsername;
java.lang.String hostname;
java.lang.Number port;
java.lang.String projectId;
java.lang.Boolean authenticationEnabled;
java.lang.Boolean authorizationEnabled;
java.lang.String authzQueryTemplate;
java.lang.String caCertificate;
java.lang.String profile;
java.lang.String status;
java.util.List userToDnMapping;
/**
* Sets the value of {@link CfnLdapConfigurationProps#getBindPassword}
* @param bindPassword Password that MongoDB Cloud uses to authenticate the bindUsername. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bindPassword(java.lang.String bindPassword) {
this.bindPassword = bindPassword;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getBindUsername}
* @param bindUsername Full Distinguished Name (DN) of the Lightweight Directory Access Protocol (LDAP) user that MongoDB Cloud uses to connect to the LDAP host. This parameter is required.
* LDAP distinguished names must be formatted according to RFC 2253.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bindUsername(java.lang.String bindUsername) {
this.bindUsername = bindUsername;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getHostname}
* @param hostname Human-readable label that identifies the hostname or Internet Protocol (IP) address of the Lightweight Directory Access Protocol (LDAP) host. This parameter is required.
* This host must have access to the internet or have a Virtual Private Cloud (VPC) peering connection to your cluster.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder hostname(java.lang.String hostname) {
this.hostname = hostname;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getPort}
* @param port Port to which the Lightweight Directory Access Protocol (LDAP) host listens for client connections. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder port(java.lang.Number port) {
this.port = port;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getProjectId}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getAuthenticationEnabled}
* @param authenticationEnabled Flag that indicates whether users can authenticate using an Lightweight Directory Access Protocol (LDAP) host.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authenticationEnabled(java.lang.Boolean authenticationEnabled) {
this.authenticationEnabled = authenticationEnabled;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getAuthorizationEnabled}
* @param authorizationEnabled Flag that indicates whether users can authorize access to MongoDB Cloud resources using an Lightweight Directory Access Protocol (LDAP) host.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authorizationEnabled(java.lang.Boolean authorizationEnabled) {
this.authorizationEnabled = authorizationEnabled;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getAuthzQueryTemplate}
* @param authzQueryTemplate Lightweight Directory Access Protocol (LDAP) query template that MongoDB Cloud runs to obtain the LDAP groups associated with the authenticated user.
* MongoDB Cloud uses this parameter only for user authorization. Use the {USER}
placeholder in the Uniform Resource Locator (URL) to substitute the authenticated username. The query relates to the host specified with the hostname. Format this query according to RFC 4515 and RFC 4516.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authzQueryTemplate(java.lang.String authzQueryTemplate) {
this.authzQueryTemplate = authzQueryTemplate;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getCaCertificate}
* @param caCertificate Certificate Authority (CA) certificate that MongoDB Cloud uses to verify the identity of the Lightweight Directory Access Protocol (LDAP) host.
* MongoDB Cloud allows self-signed certificates. To delete an assigned value, pass an empty string: "caCertificate": ""
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder caCertificate(java.lang.String caCertificate) {
this.caCertificate = caCertificate;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getProfile}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getStatus}
* @param status The current status of the LDAP over TLS/SSL configuration.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder status(java.lang.String status) {
this.status = status;
return this;
}
/**
* Sets the value of {@link CfnLdapConfigurationProps#getUserToDnMapping}
* @param userToDnMapping User-to-Distinguished Name (DN) map that MongoDB Cloud uses to transform a Lightweight Directory Access Protocol (LDAP) username into an LDAP DN.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder userToDnMapping(java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.ApiAtlasNdsUserToDnMappingView> userToDnMapping) {
this.userToDnMapping = (java.util.List)userToDnMapping;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnLdapConfigurationProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnLdapConfigurationProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnLdapConfigurationProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnLdapConfigurationProps {
private final java.lang.String bindPassword;
private final java.lang.String bindUsername;
private final java.lang.String hostname;
private final java.lang.Number port;
private final java.lang.String projectId;
private final java.lang.Boolean authenticationEnabled;
private final java.lang.Boolean authorizationEnabled;
private final java.lang.String authzQueryTemplate;
private final java.lang.String caCertificate;
private final java.lang.String profile;
private final java.lang.String status;
private final java.util.List userToDnMapping;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bindPassword = software.amazon.jsii.Kernel.get(this, "bindPassword", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.bindUsername = software.amazon.jsii.Kernel.get(this, "bindUsername", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.hostname = software.amazon.jsii.Kernel.get(this, "hostname", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.port = software.amazon.jsii.Kernel.get(this, "port", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.projectId = software.amazon.jsii.Kernel.get(this, "projectId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.authenticationEnabled = software.amazon.jsii.Kernel.get(this, "authenticationEnabled", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.authorizationEnabled = software.amazon.jsii.Kernel.get(this, "authorizationEnabled", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.authzQueryTemplate = software.amazon.jsii.Kernel.get(this, "authzQueryTemplate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.caCertificate = software.amazon.jsii.Kernel.get(this, "caCertificate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.status = software.amazon.jsii.Kernel.get(this, "status", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.userToDnMapping = software.amazon.jsii.Kernel.get(this, "userToDnMapping", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.ApiAtlasNdsUserToDnMappingView.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bindPassword = java.util.Objects.requireNonNull(builder.bindPassword, "bindPassword is required");
this.bindUsername = java.util.Objects.requireNonNull(builder.bindUsername, "bindUsername is required");
this.hostname = java.util.Objects.requireNonNull(builder.hostname, "hostname is required");
this.port = java.util.Objects.requireNonNull(builder.port, "port is required");
this.projectId = java.util.Objects.requireNonNull(builder.projectId, "projectId is required");
this.authenticationEnabled = builder.authenticationEnabled;
this.authorizationEnabled = builder.authorizationEnabled;
this.authzQueryTemplate = builder.authzQueryTemplate;
this.caCertificate = builder.caCertificate;
this.profile = builder.profile;
this.status = builder.status;
this.userToDnMapping = (java.util.List)builder.userToDnMapping;
}
@Override
public final java.lang.String getBindPassword() {
return this.bindPassword;
}
@Override
public final java.lang.String getBindUsername() {
return this.bindUsername;
}
@Override
public final java.lang.String getHostname() {
return this.hostname;
}
@Override
public final java.lang.Number getPort() {
return this.port;
}
@Override
public final java.lang.String getProjectId() {
return this.projectId;
}
@Override
public final java.lang.Boolean getAuthenticationEnabled() {
return this.authenticationEnabled;
}
@Override
public final java.lang.Boolean getAuthorizationEnabled() {
return this.authorizationEnabled;
}
@Override
public final java.lang.String getAuthzQueryTemplate() {
return this.authzQueryTemplate;
}
@Override
public final java.lang.String getCaCertificate() {
return this.caCertificate;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final java.lang.String getStatus() {
return this.status;
}
@Override
public final java.util.List getUserToDnMapping() {
return this.userToDnMapping;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bindPassword", om.valueToTree(this.getBindPassword()));
data.set("bindUsername", om.valueToTree(this.getBindUsername()));
data.set("hostname", om.valueToTree(this.getHostname()));
data.set("port", om.valueToTree(this.getPort()));
data.set("projectId", om.valueToTree(this.getProjectId()));
if (this.getAuthenticationEnabled() != null) {
data.set("authenticationEnabled", om.valueToTree(this.getAuthenticationEnabled()));
}
if (this.getAuthorizationEnabled() != null) {
data.set("authorizationEnabled", om.valueToTree(this.getAuthorizationEnabled()));
}
if (this.getAuthzQueryTemplate() != null) {
data.set("authzQueryTemplate", om.valueToTree(this.getAuthzQueryTemplate()));
}
if (this.getCaCertificate() != null) {
data.set("caCertificate", om.valueToTree(this.getCaCertificate()));
}
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getStatus() != null) {
data.set("status", om.valueToTree(this.getStatus()));
}
if (this.getUserToDnMapping() != null) {
data.set("userToDnMapping", om.valueToTree(this.getUserToDnMapping()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CfnLdapConfigurationProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnLdapConfigurationProps.Jsii$Proxy that = (CfnLdapConfigurationProps.Jsii$Proxy) o;
if (!bindPassword.equals(that.bindPassword)) return false;
if (!bindUsername.equals(that.bindUsername)) return false;
if (!hostname.equals(that.hostname)) return false;
if (!port.equals(that.port)) return false;
if (!projectId.equals(that.projectId)) return false;
if (this.authenticationEnabled != null ? !this.authenticationEnabled.equals(that.authenticationEnabled) : that.authenticationEnabled != null) return false;
if (this.authorizationEnabled != null ? !this.authorizationEnabled.equals(that.authorizationEnabled) : that.authorizationEnabled != null) return false;
if (this.authzQueryTemplate != null ? !this.authzQueryTemplate.equals(that.authzQueryTemplate) : that.authzQueryTemplate != null) return false;
if (this.caCertificate != null ? !this.caCertificate.equals(that.caCertificate) : that.caCertificate != null) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
if (this.status != null ? !this.status.equals(that.status) : that.status != null) return false;
return this.userToDnMapping != null ? this.userToDnMapping.equals(that.userToDnMapping) : that.userToDnMapping == null;
}
@Override
public final int hashCode() {
int result = this.bindPassword.hashCode();
result = 31 * result + (this.bindUsername.hashCode());
result = 31 * result + (this.hostname.hashCode());
result = 31 * result + (this.port.hashCode());
result = 31 * result + (this.projectId.hashCode());
result = 31 * result + (this.authenticationEnabled != null ? this.authenticationEnabled.hashCode() : 0);
result = 31 * result + (this.authorizationEnabled != null ? this.authorizationEnabled.hashCode() : 0);
result = 31 * result + (this.authzQueryTemplate != null ? this.authzQueryTemplate.hashCode() : 0);
result = 31 * result + (this.caCertificate != null ? this.caCertificate.hashCode() : 0);
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.status != null ? this.status.hashCode() : 0);
result = 31 * result + (this.userToDnMapping != null ? this.userToDnMapping.hashCode() : 0);
return result;
}
}
}