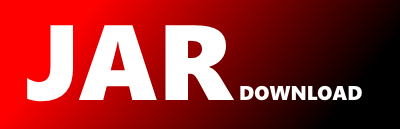
org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchiveProps Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Returns, adds, edits, or removes an online archive.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.947Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnOnlineArchiveProps")
@software.amazon.jsii.Jsii.Proxy(CfnOnlineArchiveProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnOnlineArchiveProps extends software.amazon.jsii.JsiiSerializable {
/**
* Human-readable label that identifies the cluster that contains the collection from which you want to remove an online archive.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getClusterName();
/**
* Rules by which MongoDB MongoDB Cloud archives data.
*
* Use the criteria.type field to choose how MongoDB Cloud selects data to archive. Choose data using the age of the data or a MongoDB query.
* "criteria.type": "DATE" selects documents to archive based on a date.
* "criteria.type": "CUSTOM" selects documents to archive based on a custom JSON query. MongoDB Cloud doesn't support "criteria.type": "CUSTOM" when "collectionType": "TIMESERIES".
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CriteriaView getCriteria();
/**
* Classification of MongoDB database collection that you want to return.
*
* If you set this parameter to TIMESERIES
, set "criteria.type" : "date"
and "criteria.dateFormat" : "ISODATE"
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType getCollectionType() {
return null;
}
/**
* Human-readable label that identifies the collection for which you created the online archive.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getCollName() {
return null;
}
/**
* Human-readable label of the database that contains the collection that contains the online archive.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDbName() {
return null;
}
/**
* Flag that indicates whether the response returns the total number of items (totalCount) in the response.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getIncludeCount() {
return null;
}
/**
* Number of items that the response returns per page.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getItemsPerPage() {
return null;
}
/**
* Number of the page that displays the current set of the total objects that the response returns.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getPageNum() {
return null;
}
/**
* List that contains document parameters to use to logically divide data within a collection.
*
* Partitions provide a coarse level of filtering of the underlying collection data. To divide your data, specify up to two parameters that you frequently query. Any queries that don't use these parameters result in a full collection scan of all archived documents. This takes more time and increase your costs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getPartitionFields() {
return null;
}
/**
* The profile is defined in AWS Secret manager.
*
* See Secret Manager Profile setup.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
* Unique 24-hexadecimal digit string that identifies your project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProjectId() {
return null;
}
/**
* Regular frequency and duration when archiving process occurs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.ScheduleView getSchedule() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnOnlineArchiveProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnOnlineArchiveProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String clusterName;
org.mongodb.awscdk.resources.mongodbatlas.CriteriaView criteria;
org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType collectionType;
java.lang.String collName;
java.lang.String dbName;
java.lang.Boolean includeCount;
java.lang.Number itemsPerPage;
java.lang.Number pageNum;
java.util.List partitionFields;
java.lang.String profile;
java.lang.String projectId;
org.mongodb.awscdk.resources.mongodbatlas.ScheduleView schedule;
/**
* Sets the value of {@link CfnOnlineArchiveProps#getClusterName}
* @param clusterName Human-readable label that identifies the cluster that contains the collection from which you want to remove an online archive. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder clusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getCriteria}
* @param criteria Rules by which MongoDB MongoDB Cloud archives data. This parameter is required.
* Use the criteria.type field to choose how MongoDB Cloud selects data to archive. Choose data using the age of the data or a MongoDB query.
* "criteria.type": "DATE" selects documents to archive based on a date.
* "criteria.type": "CUSTOM" selects documents to archive based on a custom JSON query. MongoDB Cloud doesn't support "criteria.type": "CUSTOM" when "collectionType": "TIMESERIES".
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder criteria(org.mongodb.awscdk.resources.mongodbatlas.CriteriaView criteria) {
this.criteria = criteria;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getCollectionType}
* @param collectionType Classification of MongoDB database collection that you want to return.
* If you set this parameter to TIMESERIES
, set "criteria.type" : "date"
and "criteria.dateFormat" : "ISODATE"
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder collectionType(org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType collectionType) {
this.collectionType = collectionType;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getCollName}
* @param collName Human-readable label that identifies the collection for which you created the online archive.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder collName(java.lang.String collName) {
this.collName = collName;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getDbName}
* @param dbName Human-readable label of the database that contains the collection that contains the online archive.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dbName(java.lang.String dbName) {
this.dbName = dbName;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getIncludeCount}
* @param includeCount Flag that indicates whether the response returns the total number of items (totalCount) in the response.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder includeCount(java.lang.Boolean includeCount) {
this.includeCount = includeCount;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getItemsPerPage}
* @param itemsPerPage Number of items that the response returns per page.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder itemsPerPage(java.lang.Number itemsPerPage) {
this.itemsPerPage = itemsPerPage;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getPageNum}
* @param pageNum Number of the page that displays the current set of the total objects that the response returns.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder pageNum(java.lang.Number pageNum) {
this.pageNum = pageNum;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getPartitionFields}
* @param partitionFields List that contains document parameters to use to logically divide data within a collection.
* Partitions provide a coarse level of filtering of the underlying collection data. To divide your data, specify up to two parameters that you frequently query. Any queries that don't use these parameters result in a full collection scan of all archived documents. This takes more time and increase your costs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder partitionFields(java.util.List extends org.mongodb.awscdk.resources.mongodbatlas.PartitionFieldView> partitionFields) {
this.partitionFields = (java.util.List)partitionFields;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getProfile}
* @param profile The profile is defined in AWS Secret manager.
* See Secret Manager Profile setup.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getProjectId}
* @param projectId Unique 24-hexadecimal digit string that identifies your project.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the value of {@link CfnOnlineArchiveProps#getSchedule}
* @param schedule Regular frequency and duration when archiving process occurs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder schedule(org.mongodb.awscdk.resources.mongodbatlas.ScheduleView schedule) {
this.schedule = schedule;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnOnlineArchiveProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnOnlineArchiveProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnOnlineArchiveProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnOnlineArchiveProps {
private final java.lang.String clusterName;
private final org.mongodb.awscdk.resources.mongodbatlas.CriteriaView criteria;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType collectionType;
private final java.lang.String collName;
private final java.lang.String dbName;
private final java.lang.Boolean includeCount;
private final java.lang.Number itemsPerPage;
private final java.lang.Number pageNum;
private final java.util.List partitionFields;
private final java.lang.String profile;
private final java.lang.String projectId;
private final org.mongodb.awscdk.resources.mongodbatlas.ScheduleView schedule;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.clusterName = software.amazon.jsii.Kernel.get(this, "clusterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.criteria = software.amazon.jsii.Kernel.get(this, "criteria", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CriteriaView.class));
this.collectionType = software.amazon.jsii.Kernel.get(this, "collectionType", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType.class));
this.collName = software.amazon.jsii.Kernel.get(this, "collName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.dbName = software.amazon.jsii.Kernel.get(this, "dbName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.includeCount = software.amazon.jsii.Kernel.get(this, "includeCount", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.itemsPerPage = software.amazon.jsii.Kernel.get(this, "itemsPerPage", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.pageNum = software.amazon.jsii.Kernel.get(this, "pageNum", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.partitionFields = software.amazon.jsii.Kernel.get(this, "partitionFields", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.PartitionFieldView.class)));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.projectId = software.amazon.jsii.Kernel.get(this, "projectId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.schedule = software.amazon.jsii.Kernel.get(this, "schedule", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.ScheduleView.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.clusterName = java.util.Objects.requireNonNull(builder.clusterName, "clusterName is required");
this.criteria = java.util.Objects.requireNonNull(builder.criteria, "criteria is required");
this.collectionType = builder.collectionType;
this.collName = builder.collName;
this.dbName = builder.dbName;
this.includeCount = builder.includeCount;
this.itemsPerPage = builder.itemsPerPage;
this.pageNum = builder.pageNum;
this.partitionFields = (java.util.List)builder.partitionFields;
this.profile = builder.profile;
this.projectId = builder.projectId;
this.schedule = builder.schedule;
}
@Override
public final java.lang.String getClusterName() {
return this.clusterName;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CriteriaView getCriteria() {
return this.criteria;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CfnOnlineArchivePropsCollectionType getCollectionType() {
return this.collectionType;
}
@Override
public final java.lang.String getCollName() {
return this.collName;
}
@Override
public final java.lang.String getDbName() {
return this.dbName;
}
@Override
public final java.lang.Boolean getIncludeCount() {
return this.includeCount;
}
@Override
public final java.lang.Number getItemsPerPage() {
return this.itemsPerPage;
}
@Override
public final java.lang.Number getPageNum() {
return this.pageNum;
}
@Override
public final java.util.List getPartitionFields() {
return this.partitionFields;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final java.lang.String getProjectId() {
return this.projectId;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.ScheduleView getSchedule() {
return this.schedule;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("clusterName", om.valueToTree(this.getClusterName()));
data.set("criteria", om.valueToTree(this.getCriteria()));
if (this.getCollectionType() != null) {
data.set("collectionType", om.valueToTree(this.getCollectionType()));
}
if (this.getCollName() != null) {
data.set("collName", om.valueToTree(this.getCollName()));
}
if (this.getDbName() != null) {
data.set("dbName", om.valueToTree(this.getDbName()));
}
if (this.getIncludeCount() != null) {
data.set("includeCount", om.valueToTree(this.getIncludeCount()));
}
if (this.getItemsPerPage() != null) {
data.set("itemsPerPage", om.valueToTree(this.getItemsPerPage()));
}
if (this.getPageNum() != null) {
data.set("pageNum", om.valueToTree(this.getPageNum()));
}
if (this.getPartitionFields() != null) {
data.set("partitionFields", om.valueToTree(this.getPartitionFields()));
}
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getProjectId() != null) {
data.set("projectId", om.valueToTree(this.getProjectId()));
}
if (this.getSchedule() != null) {
data.set("schedule", om.valueToTree(this.getSchedule()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CfnOnlineArchiveProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnOnlineArchiveProps.Jsii$Proxy that = (CfnOnlineArchiveProps.Jsii$Proxy) o;
if (!clusterName.equals(that.clusterName)) return false;
if (!criteria.equals(that.criteria)) return false;
if (this.collectionType != null ? !this.collectionType.equals(that.collectionType) : that.collectionType != null) return false;
if (this.collName != null ? !this.collName.equals(that.collName) : that.collName != null) return false;
if (this.dbName != null ? !this.dbName.equals(that.dbName) : that.dbName != null) return false;
if (this.includeCount != null ? !this.includeCount.equals(that.includeCount) : that.includeCount != null) return false;
if (this.itemsPerPage != null ? !this.itemsPerPage.equals(that.itemsPerPage) : that.itemsPerPage != null) return false;
if (this.pageNum != null ? !this.pageNum.equals(that.pageNum) : that.pageNum != null) return false;
if (this.partitionFields != null ? !this.partitionFields.equals(that.partitionFields) : that.partitionFields != null) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
if (this.projectId != null ? !this.projectId.equals(that.projectId) : that.projectId != null) return false;
return this.schedule != null ? this.schedule.equals(that.schedule) : that.schedule == null;
}
@Override
public final int hashCode() {
int result = this.clusterName.hashCode();
result = 31 * result + (this.criteria.hashCode());
result = 31 * result + (this.collectionType != null ? this.collectionType.hashCode() : 0);
result = 31 * result + (this.collName != null ? this.collName.hashCode() : 0);
result = 31 * result + (this.dbName != null ? this.dbName.hashCode() : 0);
result = 31 * result + (this.includeCount != null ? this.includeCount.hashCode() : 0);
result = 31 * result + (this.itemsPerPage != null ? this.itemsPerPage.hashCode() : 0);
result = 31 * result + (this.pageNum != null ? this.pageNum.hashCode() : 0);
result = 31 * result + (this.partitionFields != null ? this.partitionFields.hashCode() : 0);
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.projectId != null ? this.projectId.hashCode() : 0);
result = 31 * result + (this.schedule != null ? this.schedule.hashCode() : 0);
return result;
}
}
}