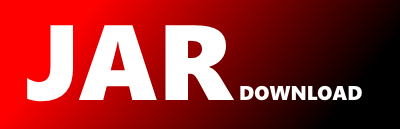
org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::Organization
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.952Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnOrganization")
public class CfnOrganization extends software.amazon.awscdk.CfnResource {
protected CfnOrganization(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnOrganization(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::Organization
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnOrganization(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnOrganizationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Attribute MongoDB::Atlas::Organization.OrgId
.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAttrOrgId() {
return software.amazon.jsii.Kernel.get(this, "attrOrgId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnOrganizationProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnOrganizationProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnOrganizationProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnOrganizationProps.Builder();
}
/**
* AwsSecretName used to set newly created Org credentials information.
*
* @return {@code this}
* @param awsSecretName AwsSecretName used to set newly created Org credentials information. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder awsSecretName(final java.lang.String awsSecretName) {
this.props.awsSecretName(awsSecretName);
return this;
}
/**
* Human-readable label that identifies the organization.
*
* @return {@code this}
* @param name Human-readable label that identifies the organization. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(final java.lang.String name) {
this.props.name(name);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies the MongoDB Cloud user that you want to assign the Organization Owner role.
*
* This user must be a member of the same organization as the calling API key. If you provide federationSettingsId, this user must instead have the Organization Owner role on an organization in the specified federation. This parameter is required only when you authenticate with Programmatic API Keys.
*
* @return {@code this}
* @param orgOwnerId Unique 24-hexadecimal digit string that identifies the MongoDB Cloud user that you want to assign the Organization Owner role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder orgOwnerId(final java.lang.String orgOwnerId) {
this.props.orgOwnerId(orgOwnerId);
return this;
}
/**
* Flag that indicates whether to require API operations to originate from an IP Address added to the API access list for the specified organization.
*
* @return {@code this}
* @param apiAccessListRequired Flag that indicates whether to require API operations to originate from an IP Address added to the API access list for the specified organization. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder apiAccessListRequired(final java.lang.Boolean apiAccessListRequired) {
this.props.apiAccessListRequired(apiAccessListRequired);
return this;
}
/**
* @return {@code this}
* @param apiKey This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder apiKey(final org.mongodb.awscdk.resources.mongodbatlas.ApiKey apiKey) {
this.props.apiKey(apiKey);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies the federation to link the newly created organization to.
*
* If specified, the proposed Organization Owner of the new organization must have the Organization Owner role in an organization associated with the federation.
*
* @return {@code this}
* @param federatedSettingsId Unique 24-hexadecimal digit string that identifies the federation to link the newly created organization to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder federatedSettingsId(final java.lang.String federatedSettingsId) {
this.props.federatedSettingsId(federatedSettingsId);
return this;
}
/**
* Flag that indicates whether this organization has been deleted.
*
* @return {@code this}
* @param isDeleted Flag that indicates whether this organization has been deleted. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder isDeleted(final java.lang.Boolean isDeleted) {
this.props.isDeleted(isDeleted);
return this;
}
/**
* Flag that indicates whether to require users to set up Multi-Factor Authentication (MFA) before accessing the specified organization.
*
* To learn more, see: https://www.mongodb.com/docs/atlas/security-multi-factor-authentication/.
*
* @return {@code this}
* @param multiFactorAuthRequired Flag that indicates whether to require users to set up Multi-Factor Authentication (MFA) before accessing the specified organization. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder multiFactorAuthRequired(final java.lang.Boolean multiFactorAuthRequired) {
this.props.multiFactorAuthRequired(multiFactorAuthRequired);
return this;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*
* @return {@code this}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* Flag that indicates whether to block MongoDB Support from accessing Atlas infrastructure for any deployment in the specified organization without explicit permission.
*
* Once this setting is turned on, you can grant MongoDB Support a 24-hour bypass access to the Atlas deployment to resolve support issues. To learn more, see: https://www.mongodb.com/docs/atlas/security-restrict-support-access/.
*
* @return {@code this}
* @param restrictEmployeeAccess Flag that indicates whether to block MongoDB Support from accessing Atlas infrastructure for any deployment in the specified organization without explicit permission. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder restrictEmployeeAccess(final java.lang.Boolean restrictEmployeeAccess) {
this.props.restrictEmployeeAccess(restrictEmployeeAccess);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnOrganization(
this.scope,
this.id,
this.props.build()
);
}
}
}