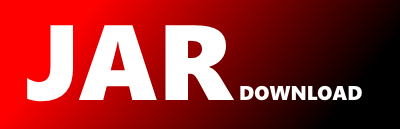
org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionProps Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* Returns, adds, edits, and removes one connection for a stream instance in the specified project.
*
* To use this resource, the requesting API Key must have the Project Owner roles.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.984Z")
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnStreamConnectionProps")
@software.amazon.jsii.Jsii.Proxy(CfnStreamConnectionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnStreamConnectionProps extends software.amazon.jsii.JsiiSerializable {
/**
* Human-readable label that identifies the stream connection.
*
* In the case of the Sample type, this is the name of the sample source.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getConnectionName();
/**
* Human-readable label that identifies the stream instance.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getInstanceName();
/**
* Unique 24-hexadecimal digit string that identifies your project.
*
* Use the /groups endpoint to retrieve all projects to which the authenticated user has access.
*
* NOTE: Groups and projects are synonymous terms. Your group id is the same as your project id. For existing groups, your group/project id remains the same. The resource and corresponding endpoints use the term groups.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getProjectId();
/**
* Type of the connection.
*
* Can be either Cluster, Kafka, or Sample.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType getType();
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication getAuthentication() {
return null;
}
/**
* Comma separated list of server addresses.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getBootstrapServers() {
return null;
}
/**
* Name of the cluster configured for this connection.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getClusterName() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getConfig() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute getDbRoleToExecute() {
return null;
}
/**
* Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getProfile() {
return null;
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity getSecurity() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnStreamConnectionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnStreamConnectionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String connectionName;
java.lang.String instanceName;
java.lang.String projectId;
org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType type;
org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication authentication;
java.lang.String bootstrapServers;
java.lang.String clusterName;
java.lang.Object config;
org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute dbRoleToExecute;
java.lang.String profile;
org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity security;
/**
* Sets the value of {@link CfnStreamConnectionProps#getConnectionName}
* @param connectionName Human-readable label that identifies the stream connection. This parameter is required.
* In the case of the Sample type, this is the name of the sample source.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder connectionName(java.lang.String connectionName) {
this.connectionName = connectionName;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getInstanceName}
* @param instanceName Human-readable label that identifies the stream instance. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceName(java.lang.String instanceName) {
this.instanceName = instanceName;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getProjectId}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
* Use the /groups endpoint to retrieve all projects to which the authenticated user has access.
*
* NOTE: Groups and projects are synonymous terms. Your group id is the same as your project id. For existing groups, your group/project id remains the same. The resource and corresponding endpoints use the term groups.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getType}
* @param type Type of the connection. This parameter is required.
* Can be either Cluster, Kafka, or Sample.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getAuthentication}
* @param authentication the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authentication(org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication authentication) {
this.authentication = authentication;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getBootstrapServers}
* @param bootstrapServers Comma separated list of server addresses.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bootstrapServers(java.lang.String bootstrapServers) {
this.bootstrapServers = bootstrapServers;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getClusterName}
* @param clusterName Name of the cluster configured for this connection.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder clusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getConfig}
* @param config the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder config(java.lang.Object config) {
this.config = config;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getDbRoleToExecute}
* @param dbRoleToExecute the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dbRoleToExecute(org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute dbRoleToExecute) {
this.dbRoleToExecute = dbRoleToExecute;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getProfile}
* @param profile Profile used to provide credentials information, (a secret with the cfn/atlas/profile/{Profile}, is required), if not provided default is used.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(java.lang.String profile) {
this.profile = profile;
return this;
}
/**
* Sets the value of {@link CfnStreamConnectionProps#getSecurity}
* @param security the value to be set.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder security(org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity security) {
this.security = security;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnStreamConnectionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnStreamConnectionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnStreamConnectionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnStreamConnectionProps {
private final java.lang.String connectionName;
private final java.lang.String instanceName;
private final java.lang.String projectId;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType type;
private final org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication authentication;
private final java.lang.String bootstrapServers;
private final java.lang.String clusterName;
private final java.lang.Object config;
private final org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute dbRoleToExecute;
private final java.lang.String profile;
private final org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity security;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.connectionName = software.amazon.jsii.Kernel.get(this, "connectionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.instanceName = software.amazon.jsii.Kernel.get(this, "instanceName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.projectId = software.amazon.jsii.Kernel.get(this, "projectId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType.class));
this.authentication = software.amazon.jsii.Kernel.get(this, "authentication", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication.class));
this.bootstrapServers = software.amazon.jsii.Kernel.get(this, "bootstrapServers", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.clusterName = software.amazon.jsii.Kernel.get(this, "clusterName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.config = software.amazon.jsii.Kernel.get(this, "config", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.dbRoleToExecute = software.amazon.jsii.Kernel.get(this, "dbRoleToExecute", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute.class));
this.profile = software.amazon.jsii.Kernel.get(this, "profile", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.security = software.amazon.jsii.Kernel.get(this, "security", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.connectionName = java.util.Objects.requireNonNull(builder.connectionName, "connectionName is required");
this.instanceName = java.util.Objects.requireNonNull(builder.instanceName, "instanceName is required");
this.projectId = java.util.Objects.requireNonNull(builder.projectId, "projectId is required");
this.type = java.util.Objects.requireNonNull(builder.type, "type is required");
this.authentication = builder.authentication;
this.bootstrapServers = builder.bootstrapServers;
this.clusterName = builder.clusterName;
this.config = builder.config;
this.dbRoleToExecute = builder.dbRoleToExecute;
this.profile = builder.profile;
this.security = builder.security;
}
@Override
public final java.lang.String getConnectionName() {
return this.connectionName;
}
@Override
public final java.lang.String getInstanceName() {
return this.instanceName;
}
@Override
public final java.lang.String getProjectId() {
return this.projectId;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.CfnStreamConnectionPropsType getType() {
return this.type;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaAuthentication getAuthentication() {
return this.authentication;
}
@Override
public final java.lang.String getBootstrapServers() {
return this.bootstrapServers;
}
@Override
public final java.lang.String getClusterName() {
return this.clusterName;
}
@Override
public final java.lang.Object getConfig() {
return this.config;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.DbRoleToExecute getDbRoleToExecute() {
return this.dbRoleToExecute;
}
@Override
public final java.lang.String getProfile() {
return this.profile;
}
@Override
public final org.mongodb.awscdk.resources.mongodbatlas.StreamsKafkaSecurity getSecurity() {
return this.security;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("connectionName", om.valueToTree(this.getConnectionName()));
data.set("instanceName", om.valueToTree(this.getInstanceName()));
data.set("projectId", om.valueToTree(this.getProjectId()));
data.set("type", om.valueToTree(this.getType()));
if (this.getAuthentication() != null) {
data.set("authentication", om.valueToTree(this.getAuthentication()));
}
if (this.getBootstrapServers() != null) {
data.set("bootstrapServers", om.valueToTree(this.getBootstrapServers()));
}
if (this.getClusterName() != null) {
data.set("clusterName", om.valueToTree(this.getClusterName()));
}
if (this.getConfig() != null) {
data.set("config", om.valueToTree(this.getConfig()));
}
if (this.getDbRoleToExecute() != null) {
data.set("dbRoleToExecute", om.valueToTree(this.getDbRoleToExecute()));
}
if (this.getProfile() != null) {
data.set("profile", om.valueToTree(this.getProfile()));
}
if (this.getSecurity() != null) {
data.set("security", om.valueToTree(this.getSecurity()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("awscdk-resources-mongodbatlas.CfnStreamConnectionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnStreamConnectionProps.Jsii$Proxy that = (CfnStreamConnectionProps.Jsii$Proxy) o;
if (!connectionName.equals(that.connectionName)) return false;
if (!instanceName.equals(that.instanceName)) return false;
if (!projectId.equals(that.projectId)) return false;
if (!type.equals(that.type)) return false;
if (this.authentication != null ? !this.authentication.equals(that.authentication) : that.authentication != null) return false;
if (this.bootstrapServers != null ? !this.bootstrapServers.equals(that.bootstrapServers) : that.bootstrapServers != null) return false;
if (this.clusterName != null ? !this.clusterName.equals(that.clusterName) : that.clusterName != null) return false;
if (this.config != null ? !this.config.equals(that.config) : that.config != null) return false;
if (this.dbRoleToExecute != null ? !this.dbRoleToExecute.equals(that.dbRoleToExecute) : that.dbRoleToExecute != null) return false;
if (this.profile != null ? !this.profile.equals(that.profile) : that.profile != null) return false;
return this.security != null ? this.security.equals(that.security) : that.security == null;
}
@Override
public final int hashCode() {
int result = this.connectionName.hashCode();
result = 31 * result + (this.instanceName.hashCode());
result = 31 * result + (this.projectId.hashCode());
result = 31 * result + (this.type.hashCode());
result = 31 * result + (this.authentication != null ? this.authentication.hashCode() : 0);
result = 31 * result + (this.bootstrapServers != null ? this.bootstrapServers.hashCode() : 0);
result = 31 * result + (this.clusterName != null ? this.clusterName.hashCode() : 0);
result = 31 * result + (this.config != null ? this.config.hashCode() : 0);
result = 31 * result + (this.dbRoleToExecute != null ? this.dbRoleToExecute.hashCode() : 0);
result = 31 * result + (this.profile != null ? this.profile.hashCode() : 0);
result = 31 * result + (this.security != null ? this.security.hashCode() : 0);
return result;
}
}
}