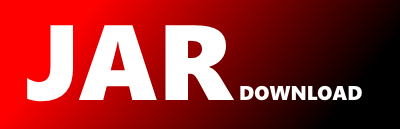
org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration Maven / Gradle / Ivy
Show all versions of awscdk-resources-mongodbatlas Show documentation
package org.mongodb.awscdk.resources.mongodbatlas;
/**
* A CloudFormation MongoDB::Atlas::ThirdPartyIntegration
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.101.0 (build b95fe5d)", date = "2024-07-30T09:54:28.989Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.mongodb.awscdk.resources.mongodbatlas.$Module.class, fqn = "awscdk-resources-mongodbatlas.CfnThirdPartyIntegration")
public class CfnThirdPartyIntegration extends software.amazon.awscdk.CfnResource {
protected CfnThirdPartyIntegration(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnThirdPartyIntegration(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new MongoDB::Atlas::ThirdPartyIntegration
.
*
* @param scope
- scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
* @param props - resource properties.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnThirdPartyIntegration(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
* Resource props.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationProps getProps() {
return software.amazon.jsii.Kernel.get(this, "props", software.amazon.jsii.NativeType.forClass(org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationProps.class));
}
/**
* A fluent builder for {@link org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined.
This parameter is required.
* @param id - scoped id of the resource.
This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationProps.Builder();
}
/**
* Key that allows MongoDB Cloud to access your Opsgenie/Datadog account.
*
* @return {@code this}
* @param apiKey Key that allows MongoDB Cloud to access your Opsgenie/Datadog account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder apiKey(final java.lang.String apiKey) {
this.props.apiKey(apiKey);
return this;
}
/**
* Key that allows MongoDB Cloud to access your Slack account.
*
* @return {@code this}
* @param apiToken Key that allows MongoDB Cloud to access your Slack account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder apiToken(final java.lang.String apiToken) {
this.props.apiToken(apiToken);
return this;
}
/**
* Name of the Slack channel to which MongoDB Cloud sends alert notifications.
*
* @return {@code this}
* @param channelName Name of the Slack channel to which MongoDB Cloud sends alert notifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder channelName(final java.lang.String channelName) {
this.props.channelName(channelName);
return this;
}
/**
* Flag that indicates whether someone has activated the Prometheus integration.
*
* @return {@code this}
* @param enabled Flag that indicates whether someone has activated the Prometheus integration. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(final java.lang.Boolean enabled) {
this.props.enabled(enabled);
return this;
}
/**
* Combination of IPv4 address and Internet Assigned Numbers Authority (IANA) port or the IANA port alone to which Prometheus binds to ingest MongoDB metrics.
*
* @return {@code this}
* @param listenAddress Combination of IPv4 address and Internet Assigned Numbers Authority (IANA) port or the IANA port alone to which Prometheus binds to ingest MongoDB metrics. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder listenAddress(final java.lang.String listenAddress) {
this.props.listenAddress(listenAddress);
return this;
}
/**
* Endpoint web address of the Microsoft Teams webhook to which MongoDB Cloud sends notifications.
*
* @return {@code this}
* @param microsoftTeamsWebhookUrl Endpoint web address of the Microsoft Teams webhook to which MongoDB Cloud sends notifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder microsoftTeamsWebhookUrl(final java.lang.String microsoftTeamsWebhookUrl) {
this.props.microsoftTeamsWebhookUrl(microsoftTeamsWebhookUrl);
return this;
}
/**
* Password required for your integration with Prometheus.
*
* @return {@code this}
* @param password Password required for your integration with Prometheus. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder password(final java.lang.String password) {
this.props.password(password);
return this;
}
/**
* The profile is defined in AWS Secret manager.
*
* See Secret Manager Profile setup.
*
* @return {@code this}
* @param profile The profile is defined in AWS Secret manager. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder profile(final java.lang.String profile) {
this.props.profile(profile);
return this;
}
/**
* Unique 24-hexadecimal digit string that identifies your project.
*
* @return {@code this}
* @param projectId Unique 24-hexadecimal digit string that identifies your project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder projectId(final java.lang.String projectId) {
this.props.projectId(projectId);
return this;
}
/**
* Two-letter code that indicates which regional URL MongoDB uses to access the Opsgenie/Datadog API.
*
* @return {@code this}
* @param region Two-letter code that indicates which regional URL MongoDB uses to access the Opsgenie/Datadog API. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(final java.lang.String region) {
this.props.region(region);
return this;
}
/**
* Routing key associated with your Splunk On-Call account.
*
* @return {@code this}
* @param routingKey Routing key associated with your Splunk On-Call account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder routingKey(final java.lang.String routingKey) {
this.props.routingKey(routingKey);
return this;
}
/**
* Security Scheme to apply to HyperText Transfer Protocol (HTTP) traffic between Prometheus and MongoDB Cloud.
*
* @return {@code this}
* @param scheme Security Scheme to apply to HyperText Transfer Protocol (HTTP) traffic between Prometheus and MongoDB Cloud. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder scheme(final org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationPropsScheme scheme) {
this.props.scheme(scheme);
return this;
}
/**
* Parameter returned if someone configure this webhook with a secret.
*
* @return {@code this}
* @param secret Parameter returned if someone configure this webhook with a secret. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secret(final java.lang.String secret) {
this.props.secret(secret);
return this;
}
/**
* Desired method to discover the Prometheus service.
*
* @return {@code this}
* @param serviceDiscovery Desired method to discover the Prometheus service. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceDiscovery(final org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationPropsServiceDiscovery serviceDiscovery) {
this.props.serviceDiscovery(serviceDiscovery);
return this;
}
/**
* Service key associated with your PagerDuty account.
*
* @return {@code this}
* @param serviceKey Service key associated with your PagerDuty account. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceKey(final java.lang.String serviceKey) {
this.props.serviceKey(serviceKey);
return this;
}
/**
* Human-readable label that identifies your Slack team.
*
* Set this parameter when you configure a legacy Slack integration.
*
* @return {@code this}
* @param teamName Human-readable label that identifies your Slack team. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder teamName(final java.lang.String teamName) {
this.props.teamName(teamName);
return this;
}
/**
* Root-relative path to the Transport Layer Security (TLS) Privacy Enhanced Mail (PEM) key and certificate file on the host.
*
* @return {@code this}
* @param tlsPemPath Root-relative path to the Transport Layer Security (TLS) Privacy Enhanced Mail (PEM) key and certificate file on the host. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder tlsPemPath(final java.lang.String tlsPemPath) {
this.props.tlsPemPath(tlsPemPath);
return this;
}
/**
* Human-readable label that identifies the service to which you want to integrate with MongoDB Cloud.
*
* The value must match the third-party service integration type.
*
* @return {@code this}
* @param type Human-readable label that identifies the service to which you want to integrate with MongoDB Cloud. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(final org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegrationPropsType type) {
this.props.type(type);
return this;
}
/**
* Endpoint web address to which MongoDB Cloud sends notifications.
*
* @return {@code this}
* @param url Endpoint web address to which MongoDB Cloud sends notifications. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder url(final java.lang.String url) {
this.props.url(url);
return this;
}
/**
* Human-readable label that identifies your Prometheus incoming webhook.
*
* @return {@code this}
* @param userName Human-readable label that identifies your Prometheus incoming webhook. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userName(final java.lang.String userName) {
this.props.userName(userName);
return this;
}
/**
* @return a newly built instance of {@link org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration build() {
return new org.mongodb.awscdk.resources.mongodbatlas.CfnThirdPartyIntegration(
this.scope,
this.id,
this.props.build()
);
}
}
}