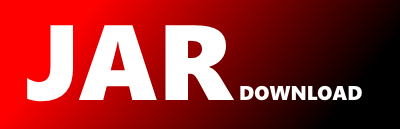
com.mongodb.reactivestreams.client.internal.ChangeStreamPublisherImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongodb-driver-reactivestreams Show documentation
Show all versions of mongodb-driver-reactivestreams Show documentation
A Reactive Streams implementation of the MongoDB Java driver
/*
* Copyright 2008-present MongoDB, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mongodb.reactivestreams.client.internal;
import com.mongodb.client.model.Collation;
import com.mongodb.client.model.changestream.ChangeStreamDocument;
import com.mongodb.client.model.changestream.FullDocument;
import com.mongodb.client.model.changestream.FullDocumentBeforeChange;
import com.mongodb.internal.async.AsyncBatchCursor;
import com.mongodb.internal.client.model.changestream.ChangeStreamLevel;
import com.mongodb.internal.operation.AsyncReadOperation;
import com.mongodb.lang.Nullable;
import com.mongodb.reactivestreams.client.ChangeStreamPublisher;
import com.mongodb.reactivestreams.client.ClientSession;
import org.bson.BsonDocument;
import org.bson.BsonString;
import org.bson.BsonTimestamp;
import org.bson.BsonValue;
import org.bson.codecs.Codec;
import org.bson.conversions.Bson;
import org.reactivestreams.Publisher;
import java.util.List;
import java.util.concurrent.TimeUnit;
import static com.mongodb.assertions.Assertions.notNull;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
final class ChangeStreamPublisherImpl extends BatchCursorPublisher>
implements ChangeStreamPublisher {
private final List extends Bson> pipeline;
private final Codec> codec;
private final ChangeStreamLevel changeStreamLevel;
private FullDocument fullDocument = FullDocument.DEFAULT;
private FullDocumentBeforeChange fullDocumentBeforeChange = FullDocumentBeforeChange.DEFAULT;
private BsonDocument resumeToken;
private BsonDocument startAfter;
private long maxAwaitTimeMS;
private Collation collation;
private BsonValue comment;
private BsonTimestamp startAtOperationTime;
private boolean showExpandedEvents;
ChangeStreamPublisherImpl(
@Nullable final ClientSession clientSession,
final MongoOperationPublisher> mongoOperationPublisher,
final Class innerResultClass,
final List extends Bson> pipeline,
final ChangeStreamLevel changeStreamLevel) {
this(clientSession, mongoOperationPublisher,
ChangeStreamDocument.createCodec(notNull("innerResultClass", innerResultClass),
mongoOperationPublisher.getCodecRegistry()),
notNull("pipeline", pipeline), notNull("changeStreamLevel", changeStreamLevel));
}
private ChangeStreamPublisherImpl(
@Nullable final ClientSession clientSession,
final MongoOperationPublisher> mongoOperationPublisher,
final Codec> codec,
final List extends Bson> pipeline,
final ChangeStreamLevel changeStreamLevel) {
super(clientSession, mongoOperationPublisher.withDocumentClass(codec.getEncoderClass()));
this.pipeline = pipeline;
this.codec = codec;
this.changeStreamLevel = changeStreamLevel;
}
@Override
public ChangeStreamPublisher fullDocument(final FullDocument fullDocument) {
this.fullDocument = notNull("fullDocument", fullDocument);
return this;
}
@Override
public ChangeStreamPublisher fullDocumentBeforeChange(final FullDocumentBeforeChange fullDocumentBeforeChange) {
this.fullDocumentBeforeChange = notNull("fullDocumentBeforeChange", fullDocumentBeforeChange);
return this;
}
@Override
public ChangeStreamPublisher resumeAfter(final BsonDocument resumeAfter) {
this.resumeToken = notNull("resumeAfter", resumeAfter);
return this;
}
@Override
public ChangeStreamPublisher batchSize(final int batchSize) {
super.batchSize(batchSize);
return this;
}
@Override
public ChangeStreamPublisher comment(@Nullable final String comment) {
this.comment = comment == null ? null : new BsonString(comment);
return this;
}
@Override
public ChangeStreamPublisher comment(@Nullable final BsonValue comment) {
this.comment = comment;
return this;
}
@Override
public ChangeStreamPublisher maxAwaitTime(final long maxAwaitTime, final TimeUnit timeUnit) {
notNull("timeUnit", timeUnit);
this.maxAwaitTimeMS = MILLISECONDS.convert(maxAwaitTime, timeUnit);
return this;
}
@Override
public ChangeStreamPublisher collation(@Nullable final Collation collation) {
this.collation = notNull("collation", collation);
return this;
}
@Override
public Publisher withDocumentClass(final Class clazz) {
return new BatchCursorPublisher(getClientSession(), getMongoOperationPublisher().withDocumentClass(clazz),
getBatchSize()) {
@Override
AsyncReadOperation> asAsyncReadOperation(final int initialBatchSize) {
return createChangeStreamOperation(getMongoOperationPublisher().getCodecRegistry().get(clazz), initialBatchSize);
}
};
}
@Override
public ChangeStreamPublisher showExpandedEvents(final boolean showExpandedEvents) {
this.showExpandedEvents = showExpandedEvents;
return this;
}
@Override
public ChangeStreamPublisher startAtOperationTime(final BsonTimestamp startAtOperationTime) {
this.startAtOperationTime = notNull("startAtOperationTime", startAtOperationTime);
return this;
}
@Override
public ChangeStreamPublisherImpl startAfter(final BsonDocument startAfter) {
this.startAfter = notNull("startAfter", startAfter);
return this;
}
@Override
AsyncReadOperation>> asAsyncReadOperation(final int initialBatchSize) {
return createChangeStreamOperation(codec, initialBatchSize);
}
private AsyncReadOperation> createChangeStreamOperation(final Codec codec, final int initialBatchSize) {
return getOperations().changeStream(fullDocument, fullDocumentBeforeChange, pipeline, codec, changeStreamLevel, initialBatchSize,
collation, comment, maxAwaitTimeMS, resumeToken, startAtOperationTime, startAfter, showExpandedEvents);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy