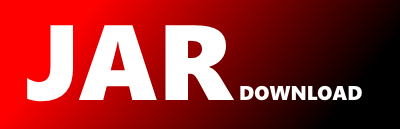
com.mongodb.reactivestreams.client.internal.FindPublisherImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongodb-driver-reactivestreams Show documentation
Show all versions of mongodb-driver-reactivestreams Show documentation
A Reactive Streams implementation of the MongoDB Java driver
/*
* Copyright 2008-present MongoDB, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mongodb.reactivestreams.client.internal;
import com.mongodb.CursorType;
import com.mongodb.ExplainVerbosity;
import com.mongodb.client.cursor.TimeoutMode;
import com.mongodb.client.model.Collation;
import com.mongodb.internal.TimeoutSettings;
import com.mongodb.internal.async.AsyncBatchCursor;
import com.mongodb.internal.client.model.FindOptions;
import com.mongodb.internal.operation.AsyncExplainableReadOperation;
import com.mongodb.internal.operation.AsyncOperations;
import com.mongodb.internal.operation.AsyncReadOperation;
import com.mongodb.lang.Nullable;
import com.mongodb.reactivestreams.client.ClientSession;
import com.mongodb.reactivestreams.client.FindPublisher;
import org.bson.BsonValue;
import org.bson.Document;
import org.bson.conversions.Bson;
import org.reactivestreams.Publisher;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
import static com.mongodb.assertions.Assertions.notNull;
final class FindPublisherImpl extends BatchCursorPublisher implements FindPublisher {
private final FindOptions findOptions;
private Bson filter;
FindPublisherImpl(@Nullable final ClientSession clientSession, final MongoOperationPublisher mongoOperationPublisher,
final Bson filter) {
super(clientSession, mongoOperationPublisher);
this.filter = notNull("filter", filter);
this.findOptions = new FindOptions();
}
@Override
public FindPublisher filter(@Nullable final Bson filter) {
this.filter = filter;
return this;
}
@Override
public FindPublisher limit(final int limit) {
findOptions.limit(limit);
return this;
}
@Override
public FindPublisher skip(final int skip) {
findOptions.skip(skip);
return this;
}
@Override
public FindPublisher maxTime(final long maxTime, final TimeUnit timeUnit) {
notNull("timeUnit", timeUnit);
findOptions.maxTime(maxTime, timeUnit);
return this;
}
@Override
public FindPublisher maxAwaitTime(final long maxAwaitTime, final TimeUnit timeUnit) {
validateMaxAwaitTime(maxAwaitTime, timeUnit);
findOptions.maxAwaitTime(maxAwaitTime, timeUnit);
return this;
}
@Override
public FindPublisher batchSize(final int batchSize) {
super.batchSize(batchSize);
findOptions.batchSize(batchSize);
return this;
}
@Override
public FindPublisher collation(@Nullable final Collation collation) {
findOptions.collation(collation);
return this;
}
@Override
public FindPublisher projection(@Nullable final Bson projection) {
findOptions.projection(projection);
return this;
}
@Override
public FindPublisher sort(@Nullable final Bson sort) {
findOptions.sort(sort);
return this;
}
@Override
public FindPublisher noCursorTimeout(final boolean noCursorTimeout) {
findOptions.noCursorTimeout(noCursorTimeout);
return this;
}
@Override
public FindPublisher partial(final boolean partial) {
findOptions.partial(partial);
return this;
}
@Override
public FindPublisher cursorType(final CursorType cursorType) {
findOptions.cursorType(cursorType);
return this;
}
@Override
public FindPublisher comment(@Nullable final String comment) {
findOptions.comment(comment);
return this;
}
@Override
public FindPublisher comment(@Nullable final BsonValue comment) {
findOptions.comment(comment);
return this;
}
@Override
public FindPublisher hint(@Nullable final Bson hint) {
findOptions.hint(hint);
return this;
}
@Override
public FindPublisher hintString(@Nullable final String hint) {
findOptions.hintString(hint);
return this;
}
@Override
public FindPublisher let(@Nullable final Bson variables) {
findOptions.let(variables);
return this;
}
@Override
public FindPublisher max(@Nullable final Bson max) {
findOptions.max(max);
return this;
}
@Override
public FindPublisher min(@Nullable final Bson min) {
findOptions.min(min);
return this;
}
@Override
public FindPublisher returnKey(final boolean returnKey) {
findOptions.returnKey(returnKey);
return this;
}
@Override
public FindPublisher showRecordId(final boolean showRecordId) {
findOptions.showRecordId(showRecordId);
return this;
}
@Override
public FindPublisher allowDiskUse(@Nullable final Boolean allowDiskUse) {
findOptions.allowDiskUse(allowDiskUse);
return this;
}
@Override
public FindPublisher timeoutMode(final TimeoutMode timeoutMode) {
super.timeoutMode(timeoutMode);
findOptions.timeoutMode(timeoutMode);
return this;
}
@Override
public Publisher explain() {
return publishExplain(Document.class, null);
}
@Override
public Publisher explain(final ExplainVerbosity verbosity) {
return publishExplain(Document.class, notNull("verbosity", verbosity));
}
@Override
public Publisher explain(final Class explainResultClass) {
return publishExplain(explainResultClass, null);
}
@Override
public Publisher explain(final Class explainResultClass, final ExplainVerbosity verbosity) {
return publishExplain(explainResultClass, notNull("verbosity", verbosity));
}
private Publisher publishExplain(final Class explainResultClass, @Nullable final ExplainVerbosity verbosity) {
notNull("explainDocumentClass", explainResultClass);
return getMongoOperationPublisher().createReadOperationMono(
getTimeoutSettings(),
() -> asAsyncReadOperation(0)
.asAsyncExplainableOperation(verbosity, getCodecRegistry().get(explainResultClass)), getClientSession());
}
@Override
AsyncExplainableReadOperation> asAsyncReadOperation(final int initialBatchSize) {
return getOperations().find(filter, getDocumentClass(), findOptions.withBatchSize(initialBatchSize));
}
@Override
Function, TimeoutSettings> getTimeoutSettings() {
return (asyncOperations -> asyncOperations.createTimeoutSettings(findOptions));
}
@Override
AsyncReadOperation> asAsyncFirstReadOperation() {
return getOperations().findFirst(filter, getDocumentClass(), findOptions);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy