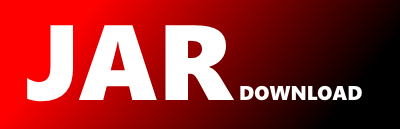
com.mongodb.FindIterableImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mongodb-driver Show documentation
Show all versions of mongodb-driver Show documentation
The MongoDB Driver uber-artifact that combines mongodb-driver-sync and the legacy driver
/*
* Copyright (c) 2008-2014 MongoDB, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mongodb;
import com.mongodb.client.FindIterable;
import com.mongodb.client.MongoCursor;
import com.mongodb.client.MongoIterable;
import com.mongodb.client.model.Collation;
import com.mongodb.client.model.FindOptions;
import com.mongodb.operation.BatchCursor;
import com.mongodb.operation.FindOperation;
import com.mongodb.operation.OperationExecutor;
import org.bson.BsonDocument;
import org.bson.codecs.configuration.CodecRegistry;
import org.bson.conversions.Bson;
import java.util.Collection;
import java.util.concurrent.TimeUnit;
import static com.mongodb.assertions.Assertions.notNull;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
final class FindIterableImpl implements FindIterable {
private final MongoNamespace namespace;
private final Class documentClass;
private final Class resultClass;
private final ReadPreference readPreference;
private final ReadConcern readConcern;
private final CodecRegistry codecRegistry;
private final OperationExecutor executor;
private final FindOptions findOptions;
private Bson filter;
FindIterableImpl(final MongoNamespace namespace, final Class documentClass, final Class resultClass,
final CodecRegistry codecRegistry, final ReadPreference readPreference, final ReadConcern readConcern,
final OperationExecutor executor, final Bson filter, final FindOptions findOptions) {
this.namespace = notNull("namespace", namespace);
this.documentClass = notNull("documentClass", documentClass);
this.resultClass = notNull("resultClass", resultClass);
this.codecRegistry = notNull("codecRegistry", codecRegistry);
this.readPreference = notNull("readPreference", readPreference);
this.readConcern = notNull("readConcern", readConcern);
this.executor = notNull("executor", executor);
this.filter = notNull("filter", filter);
this.findOptions = notNull("findOptions", findOptions);
}
@Override
public FindIterable filter(final Bson filter) {
this.filter = filter;
return this;
}
@Override
public FindIterable limit(final int limit) {
findOptions.limit(limit);
return this;
}
@Override
public FindIterable skip(final int skip) {
findOptions.skip(skip);
return this;
}
@Override
public FindIterable maxTime(final long maxTime, final TimeUnit timeUnit) {
notNull("timeUnit", timeUnit);
findOptions.maxTime(maxTime, timeUnit);
return this;
}
@Override
public FindIterable maxAwaitTime(final long maxAwaitTime, final TimeUnit timeUnit) {
notNull("timeUnit", timeUnit);
findOptions.maxAwaitTime(maxAwaitTime, timeUnit);
return this;
}
@Override
public FindIterable batchSize(final int batchSize) {
findOptions.batchSize(batchSize);
return this;
}
@Override
public FindIterable collation(final Collation collation) {
findOptions.collation(collation);
return this;
}
@Override
public FindIterable modifiers(final Bson modifiers) {
findOptions.modifiers(modifiers);
return this;
}
@Override
public FindIterable projection(final Bson projection) {
findOptions.projection(projection);
return this;
}
@Override
public FindIterable sort(final Bson sort) {
findOptions.sort(sort);
return this;
}
@Override
public FindIterable noCursorTimeout(final boolean noCursorTimeout) {
findOptions.noCursorTimeout(noCursorTimeout);
return this;
}
@Override
public FindIterable oplogReplay(final boolean oplogReplay) {
findOptions.oplogReplay(oplogReplay);
return this;
}
@Override
public FindIterable partial(final boolean partial) {
findOptions.partial(partial);
return this;
}
@Override
public FindIterable cursorType(final CursorType cursorType) {
findOptions.cursorType(cursorType);
return this;
}
@Override
public MongoCursor iterator() {
return execute().iterator();
}
@Override
public TResult first() {
return execute().first();
}
@Override
public MongoIterable map(final Function mapper) {
return new MappingIterable(this, mapper);
}
@Override
public void forEach(final Block super TResult> block) {
execute().forEach(block);
}
@Override
public > A into(final A target) {
return execute().into(target);
}
private MongoIterable execute() {
return new FindOperationIterable(createQueryOperation(), readPreference, executor);
}
private FindOperation createQueryOperation() {
return new FindOperation(namespace, codecRegistry.get(resultClass))
.filter(filter.toBsonDocument(documentClass, codecRegistry))
.batchSize(findOptions.getBatchSize())
.skip(findOptions.getSkip())
.limit(findOptions.getLimit())
.maxTime(findOptions.getMaxTime(MILLISECONDS), MILLISECONDS)
.maxAwaitTime(findOptions.getMaxAwaitTime(MILLISECONDS), MILLISECONDS)
.modifiers(toBsonDocument(findOptions.getModifiers()))
.projection(toBsonDocument(findOptions.getProjection()))
.sort(toBsonDocument(findOptions.getSort()))
.cursorType(findOptions.getCursorType())
.noCursorTimeout(findOptions.isNoCursorTimeout())
.oplogReplay(findOptions.isOplogReplay())
.partial(findOptions.isPartial())
.slaveOk(readPreference.isSlaveOk())
.readConcern(readConcern)
.collation(findOptions.getCollation());
}
private BsonDocument toBsonDocument(final Bson document) {
return document == null ? null : document.toBsonDocument(documentClass, codecRegistry);
}
private final class FindOperationIterable extends OperationIterable {
private final ReadPreference readPreference;
private final OperationExecutor executor;
FindOperationIterable(final FindOperation operation, final ReadPreference readPreference,
final OperationExecutor executor) {
super(operation, readPreference, executor);
this.readPreference = readPreference;
this.executor = executor;
}
@Override
public TResult first() {
FindOperation findFirstOperation = createQueryOperation().batchSize(0).limit(-1);
BatchCursor batchCursor = executor.execute(findFirstOperation, readPreference);
return batchCursor.hasNext() ? batchCursor.next().iterator().next() : null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy