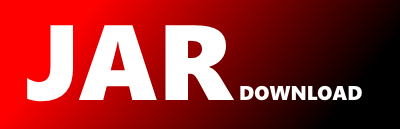
org.mortbay.jetty.plugin.HelpMojo Maven / Gradle / Ivy
package org.mortbay.jetty.plugin;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
/**
* Display help information on jetty-maven-plugin.
Call mvn jetty:help -Ddetail=true -Dgoal=<goal-name>
to display parameter details.
*
* @version generated on Fri Jul 26 14:56:37 CDT 2013
* @author org.apache.maven.tools.plugin.generator.PluginHelpGenerator (version 2.9)
* @goal help
* @requiresProject false
* @threadSafe
*/
public class HelpMojo
extends AbstractMojo
{
/**
* If true
, display all settable properties for each goal.
*
* @parameter expression="${detail}" default-value="false"
*/
private boolean detail;
/**
* The name of the goal for which to show help. If unspecified, all goals will be displayed.
*
* @parameter expression="${goal}"
*/
private java.lang.String goal;
/**
* The maximum length of a display line, should be positive.
*
* @parameter expression="${lineLength}" default-value="80"
*/
private int lineLength;
/**
* The number of spaces per indentation level, should be positive.
*
* @parameter expression="${indentSize}" default-value="2"
*/
private int indentSize;
/** {@inheritDoc} */
public void execute()
throws MojoExecutionException
{
if ( lineLength <= 0 )
{
getLog().warn( "The parameter 'lineLength' should be positive, using '80' as default." );
lineLength = 80;
}
if ( indentSize <= 0 )
{
getLog().warn( "The parameter 'indentSize' should be positive, using '2' as default." );
indentSize = 2;
}
StringBuffer sb = new StringBuffer();
append( sb, "org.mortbay.jetty:jetty-maven-plugin:8.1.12.v20130726", 0 );
append( sb, "", 0 );
append( sb, "Jetty :: Jetty Maven Plugin", 0 );
append( sb, "Jetty integrations and distributions", 1 );
append( sb, "", 0 );
if ( goal == null || goal.length() <= 0 )
{
append( sb, "This plugin has 8 goals:", 0 );
append( sb, "", 0 );
}
if ( goal == null || goal.length() <= 0 || "deploy-war".equals( goal ) )
{
append( sb, "jetty:deploy-war", 0 );
append( sb, "This goal is used to run Jetty with a pre-assembled war.\n\nIt accepts exactly the same options as the run-war goal. However, it doesn\'t assume that the current artifact is a webapp and doesn\'t try to assemble it into a war before its execution. So using it makes sense only when used in conjunction with the webApp configuration parameter pointing to a pre-built WAR.\n\nThis goal is useful e.g. for launching a web app in Jetty as a target for unit-tested HTTP client components.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "connectors", 2 );
append( sb, "List of connectors to use. If none are configured then the default is a single SelectChannelConnector at port 8080. You can override this default port number by using the system property jetty.port on the command line, eg: mvn -Djetty.port=9999 jetty:run. Consider using instead the element to specify external jetty xml config file.", 3 );
append( sb, "", 0 );
append( sb, "contextHandlers", 2 );
append( sb, "List of other contexts to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "daemon (Default: false)", 2 );
append( sb, "Determines whether or not the server blocks when started. The default behavior (daemon = false) will cause the server to pause other processes while it continues to handle web requests. This is useful when starting the server with the intent to work with it interactively.\n\nOften, it is desirable to let the server start and continue running subsequent processes in an automated build environment. This can be facilitated by setting daemon to true.\n", 3 );
append( sb, "Expression: ${jetty.daemon}", 3 );
append( sb, "", 0 );
append( sb, "excludedGoals", 2 );
append( sb, "List of goals that are NOT to be used", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Comma separated list of a jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "loginServices", 2 );
append( sb, "List of security realms to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "reload (Default: automatic)", 2 );
append( sb, "reload can be set to either \'automatic\' or \'manual\' if \'manual\' then the context can be reloaded by a linefeed in the console if \'automatic\' then traditional reloading on changed files is enabled.", 3 );
append( sb, "Expression: ${jetty.reload}", 3 );
append( sb, "", 0 );
append( sb, "requestLog", 2 );
append( sb, "A RequestLog implementation to use for the webapp at runtime. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanIntervalSeconds (Default: 0)", 2 );
append( sb, "The interval in seconds to scan the webapp for changes and restart the context if necessary. Ignored if reload is enabled. Disabled by default.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${jetty.scanIntervalSeconds}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip this mojo execution.", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "systemProperties", 2 );
append( sb, "System properties to set before execution. Note that these properties will NOT override System properties that have been set on the command line or by the JVM. They WILL override System properties that have been set via systemPropertiesFile. Optional.", 3 );
append( sb, "", 0 );
append( sb, "systemPropertiesFile", 2 );
append( sb, "File containing system properties to be set before execution Note that these properties will NOT override System properties that have been set on the command line, by the JVM, or directly in the POM via systemProperties. Optional.", 3 );
append( sb, "Expression: ${jetty.systemPropertiesFile}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "war", 2 );
append( sb, "The location of the war file.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}.war", 3 );
append( sb, "", 0 );
append( sb, "webApp", 2 );
append( sb, "An instance of org.eclipse.jetty.webapp.WebAppContext that represents the webapp. Use any of its setters to configure the webapp. This is the preferred and most flexible method of configuration, rather than using the (deprecated) individual parameters like \'tmpDirectory\', \'contextPath\' etc.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "help".equals( goal ) )
{
append( sb, "jetty:help", 0 );
append( sb, "Display help information on jetty-maven-plugin.\nCall\n\u00a0\u00a0mvn\u00a0jetty:help\u00a0-Ddetail=true\u00a0-Dgoal=\nto display parameter details.", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "detail (Default: false)", 2 );
append( sb, "If true, display all settable properties for each goal.", 3 );
append( sb, "Expression: ${detail}", 3 );
append( sb, "", 0 );
append( sb, "goal", 2 );
append( sb, "The name of the goal for which to show help. If unspecified, all goals will be displayed.", 3 );
append( sb, "Expression: ${goal}", 3 );
append( sb, "", 0 );
append( sb, "indentSize (Default: 2)", 2 );
append( sb, "The number of spaces per indentation level, should be positive.", 3 );
append( sb, "Expression: ${indentSize}", 3 );
append( sb, "", 0 );
append( sb, "lineLength (Default: 80)", 2 );
append( sb, "The maximum length of a display line, should be positive.", 3 );
append( sb, "Expression: ${lineLength}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "run".equals( goal ) )
{
append( sb, "jetty:run", 0 );
append( sb, "This goal is used in-situ on a Maven project without first requiring that the project is assembled into a war, saving time during the development cycle. The plugin forks a parallel lifecycle to ensure that the \'compile\' phase has been completed before invoking Jetty. This means that you do not need to explicity execute a \'mvn compile\' first. It also means that a \'mvn clean jetty:run\' will ensure that a full fresh compile is done before invoking Jetty.\n\nOnce invoked, the plugin can be configured to run continuously, scanning for changes in the project and automatically performing a hot redeploy when necessary. This allows the developer to concentrate on coding changes to the project using their IDE of choice and have those changes immediately and transparently reflected in the running web container, eliminating development time that is wasted on rebuilding, reassembling and redeploying.\n\nYou may also specify the location of a jetty.xml file whose contents will be applied before any plugin configuration. This can be used, for example, to deploy a static webapp that is not part of your maven build.\n\nThere is a reference guide to the configuration parameters for this plugin, and more detailed information with examples in the Configuration Guide.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "classesDirectory", 2 );
append( sb, "The directory containing generated classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.outputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "connectors", 2 );
append( sb, "List of connectors to use. If none are configured then the default is a single SelectChannelConnector at port 8080. You can override this default port number by using the system property jetty.port on the command line, eg: mvn -Djetty.port=9999 jetty:run. Consider using instead the element to specify external jetty xml config file.", 3 );
append( sb, "", 0 );
append( sb, "contextHandlers", 2 );
append( sb, "List of other contexts to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "daemon (Default: false)", 2 );
append( sb, "Determines whether or not the server blocks when started. The default behavior (daemon = false) will cause the server to pause other processes while it continues to handle web requests. This is useful when starting the server with the intent to work with it interactively.\n\nOften, it is desirable to let the server start and continue running subsequent processes in an automated build environment. This can be facilitated by setting daemon to true.\n", 3 );
append( sb, "Expression: ${jetty.daemon}", 3 );
append( sb, "", 0 );
append( sb, "excludedGoals", 2 );
append( sb, "List of goals that are NOT to be used", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Comma separated list of a jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "loginServices", 2 );
append( sb, "List of security realms to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "reload (Default: automatic)", 2 );
append( sb, "reload can be set to either \'automatic\' or \'manual\' if \'manual\' then the context can be reloaded by a linefeed in the console if \'automatic\' then traditional reloading on changed files is enabled.", 3 );
append( sb, "Expression: ${jetty.reload}", 3 );
append( sb, "", 0 );
append( sb, "requestLog", 2 );
append( sb, "A RequestLog implementation to use for the webapp at runtime. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanIntervalSeconds (Default: 0)", 2 );
append( sb, "The interval in seconds to scan the webapp for changes and restart the context if necessary. Ignored if reload is enabled. Disabled by default.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${jetty.scanIntervalSeconds}", 3 );
append( sb, "", 0 );
append( sb, "scanTargetPatterns", 2 );
append( sb, "List of directories with ant-style and patterns for extra targets to periodically scan for changes. Can be used instead of, or in conjunction with .Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanTargets", 2 );
append( sb, "List of files or directories to additionally periodically scan for changes. Optional.", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip this mojo execution.", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "systemProperties", 2 );
append( sb, "System properties to set before execution. Note that these properties will NOT override System properties that have been set on the command line or by the JVM. They WILL override System properties that have been set via systemPropertiesFile. Optional.", 3 );
append( sb, "", 0 );
append( sb, "systemPropertiesFile", 2 );
append( sb, "File containing system properties to be set before execution Note that these properties will NOT override System properties that have been set on the command line, by the JVM, or directly in the POM via systemProperties. Optional.", 3 );
append( sb, "Expression: ${jetty.systemPropertiesFile}", 3 );
append( sb, "", 0 );
append( sb, "testClassesDirectory", 2 );
append( sb, "The directory containing generated test classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.testOutputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "useTestScope (Default: false)", 2 );
append( sb, "If true, the and the dependencies of test will be put first on the runtime classpath.", 3 );
append( sb, "", 0 );
append( sb, "webApp", 2 );
append( sb, "An instance of org.eclipse.jetty.webapp.WebAppContext that represents the webapp. Use any of its setters to configure the webapp. This is the preferred and most flexible method of configuration, rather than using the (deprecated) individual parameters like \'tmpDirectory\', \'contextPath\' etc.", 3 );
append( sb, "", 0 );
append( sb, "webAppSourceDirectory", 2 );
append( sb, "Root directory for all html/jsp etc files", 3 );
append( sb, "Expression: ${maven.war.src}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "run-exploded".equals( goal ) )
{
append( sb, "jetty:run-exploded", 0 );
append( sb, "This goal is used to assemble your webapp into an exploded war and automatically deploy it to Jetty.\n\nOnce invoked, the plugin can be configured to run continuously, scanning for changes in the pom.xml and to WEB-INF/web.xml, WEB-INF/classes or WEB-INF/lib and hot redeploy when a change is detected.\n\nYou may also specify the location of a jetty.xml file whose contents will be applied before any plugin configuration. This can be used, for example, to deploy a static webapp that is not part of your maven build.\n\nThere is a reference guide to the configuration parameters for this plugin, and more detailed information with examples in the Configuration Guide.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "connectors", 2 );
append( sb, "List of connectors to use. If none are configured then the default is a single SelectChannelConnector at port 8080. You can override this default port number by using the system property jetty.port on the command line, eg: mvn -Djetty.port=9999 jetty:run. Consider using instead the element to specify external jetty xml config file.", 3 );
append( sb, "", 0 );
append( sb, "contextHandlers", 2 );
append( sb, "List of other contexts to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "daemon (Default: false)", 2 );
append( sb, "Determines whether or not the server blocks when started. The default behavior (daemon = false) will cause the server to pause other processes while it continues to handle web requests. This is useful when starting the server with the intent to work with it interactively.\n\nOften, it is desirable to let the server start and continue running subsequent processes in an automated build environment. This can be facilitated by setting daemon to true.\n", 3 );
append( sb, "Expression: ${jetty.daemon}", 3 );
append( sb, "", 0 );
append( sb, "excludedGoals", 2 );
append( sb, "List of goals that are NOT to be used", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Comma separated list of a jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "loginServices", 2 );
append( sb, "List of security realms to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "reload (Default: automatic)", 2 );
append( sb, "reload can be set to either \'automatic\' or \'manual\' if \'manual\' then the context can be reloaded by a linefeed in the console if \'automatic\' then traditional reloading on changed files is enabled.", 3 );
append( sb, "Expression: ${jetty.reload}", 3 );
append( sb, "", 0 );
append( sb, "requestLog", 2 );
append( sb, "A RequestLog implementation to use for the webapp at runtime. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanIntervalSeconds (Default: 0)", 2 );
append( sb, "The interval in seconds to scan the webapp for changes and restart the context if necessary. Ignored if reload is enabled. Disabled by default.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${jetty.scanIntervalSeconds}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip this mojo execution.", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "systemProperties", 2 );
append( sb, "System properties to set before execution. Note that these properties will NOT override System properties that have been set on the command line or by the JVM. They WILL override System properties that have been set via systemPropertiesFile. Optional.", 3 );
append( sb, "", 0 );
append( sb, "systemPropertiesFile", 2 );
append( sb, "File containing system properties to be set before execution Note that these properties will NOT override System properties that have been set on the command line, by the JVM, or directly in the POM via systemProperties. Optional.", 3 );
append( sb, "Expression: ${jetty.systemPropertiesFile}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "war", 2 );
append( sb, "The location of the war file.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}", 3 );
append( sb, "", 0 );
append( sb, "webApp", 2 );
append( sb, "An instance of org.eclipse.jetty.webapp.WebAppContext that represents the webapp. Use any of its setters to configure the webapp. This is the preferred and most flexible method of configuration, rather than using the (deprecated) individual parameters like \'tmpDirectory\', \'contextPath\' etc.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "run-forked".equals( goal ) )
{
append( sb, "jetty:run-forked", 0 );
append( sb, "This goal is used to assemble your webapp into a war and automatically deploy it to Jetty in a forked JVM.\n\nYou need to define a jetty.xml file to configure connectors etc and a context xml file that sets up anything special about your webapp. This plugin will fill in the:\n\n-\tcontext path\n-\tclasses\n-\tweb.xml\n-\troot of the webapp\nBased on a combination of information that you supply and the location of files in your unassembled webapp.\n\n\nThere is a reference guide to the configuration parameters for this plugin, and more detailed information with examples in the Configuration Guide.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "baseAppFirst (Default: true)", 2 );
append( sb, "If true, the webAppSourceDirectory will be first on the list of resources that form the resource base for the webapp. If false, it will be last.", 3 );
append( sb, "", 0 );
append( sb, "classesDirectory", 2 );
append( sb, "The directory containing generated classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.outputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Location of jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "jvmArgs", 2 );
append( sb, "Arbitrary jvm args to pass to the forked process", 3 );
append( sb, "", 0 );
append( sb, "maxStartupLines (Default: 50)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "", 0 );
append( sb, "resourceBases", 2 );
append( sb, "Directories that contain static resources for the webapp. Optional.", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "testClassesDirectory", 2 );
append( sb, "The directory containing generated test classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.testOutputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "useTestScope (Default: false)", 2 );
append( sb, "If true, the and the dependencies of test will be put first on the runtime classpath.", 3 );
append( sb, "", 0 );
append( sb, "waitForChild (Default: true)", 2 );
append( sb, "(no description available)", 3 );
append( sb, "Expression: true", 3 );
append( sb, "", 0 );
append( sb, "webAppSourceDirectory", 2 );
append( sb, "Root directory for all html/jsp etc files", 3 );
append( sb, "Expression: ${basedir}/src/main/webapp", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "run-war".equals( goal ) )
{
append( sb, "jetty:run-war", 0 );
append( sb, "This goal is used to assemble your webapp into a war and automatically deploy it to Jetty.\n\nOnce invoked, the plugin can be configured to run continuously, scanning for changes in the project and to the war file and automatically performing a hot redeploy when necessary.\n\nYou may also specify the location of a jetty.xml file whose contents will be applied before any plugin configuration. This can be used, for example, to deploy a static webapp that is not part of your maven build.\n\nThere is a reference guide to the configuration parameters for this plugin, and more detailed information with examples in the Configuration Guide.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "connectors", 2 );
append( sb, "List of connectors to use. If none are configured then the default is a single SelectChannelConnector at port 8080. You can override this default port number by using the system property jetty.port on the command line, eg: mvn -Djetty.port=9999 jetty:run. Consider using instead the element to specify external jetty xml config file.", 3 );
append( sb, "", 0 );
append( sb, "contextHandlers", 2 );
append( sb, "List of other contexts to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "daemon (Default: false)", 2 );
append( sb, "Determines whether or not the server blocks when started. The default behavior (daemon = false) will cause the server to pause other processes while it continues to handle web requests. This is useful when starting the server with the intent to work with it interactively.\n\nOften, it is desirable to let the server start and continue running subsequent processes in an automated build environment. This can be facilitated by setting daemon to true.\n", 3 );
append( sb, "Expression: ${jetty.daemon}", 3 );
append( sb, "", 0 );
append( sb, "excludedGoals", 2 );
append( sb, "List of goals that are NOT to be used", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Comma separated list of a jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "loginServices", 2 );
append( sb, "List of security realms to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "reload (Default: automatic)", 2 );
append( sb, "reload can be set to either \'automatic\' or \'manual\' if \'manual\' then the context can be reloaded by a linefeed in the console if \'automatic\' then traditional reloading on changed files is enabled.", 3 );
append( sb, "Expression: ${jetty.reload}", 3 );
append( sb, "", 0 );
append( sb, "requestLog", 2 );
append( sb, "A RequestLog implementation to use for the webapp at runtime. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanIntervalSeconds (Default: 0)", 2 );
append( sb, "The interval in seconds to scan the webapp for changes and restart the context if necessary. Ignored if reload is enabled. Disabled by default.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${jetty.scanIntervalSeconds}", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip this mojo execution.", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "systemProperties", 2 );
append( sb, "System properties to set before execution. Note that these properties will NOT override System properties that have been set on the command line or by the JVM. They WILL override System properties that have been set via systemPropertiesFile. Optional.", 3 );
append( sb, "", 0 );
append( sb, "systemPropertiesFile", 2 );
append( sb, "File containing system properties to be set before execution Note that these properties will NOT override System properties that have been set on the command line, by the JVM, or directly in the POM via systemProperties. Optional.", 3 );
append( sb, "Expression: ${jetty.systemPropertiesFile}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "war", 2 );
append( sb, "The location of the war file.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.directory}/${project.build.finalName}.war", 3 );
append( sb, "", 0 );
append( sb, "webApp", 2 );
append( sb, "An instance of org.eclipse.jetty.webapp.WebAppContext that represents the webapp. Use any of its setters to configure the webapp. This is the preferred and most flexible method of configuration, rather than using the (deprecated) individual parameters like \'tmpDirectory\', \'contextPath\' etc.", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "start".equals( goal ) )
{
append( sb, "jetty:start", 0 );
append( sb, "This goal is similar to the jetty:run goal, EXCEPT that it is designed to be bound to an execution inside your pom, rather than being run from the command line.\n\nWhen using it, be careful to ensure that you bind it to a phase in which all necessary generated files and classes for the webapp will have been created. If you run it from the command line, then also ensure that all necessary generated files and classes for the webapp already exist.\n", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "classesDirectory", 2 );
append( sb, "The directory containing generated classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.outputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "connectors", 2 );
append( sb, "List of connectors to use. If none are configured then the default is a single SelectChannelConnector at port 8080. You can override this default port number by using the system property jetty.port on the command line, eg: mvn -Djetty.port=9999 jetty:run. Consider using instead the element to specify external jetty xml config file.", 3 );
append( sb, "", 0 );
append( sb, "contextHandlers", 2 );
append( sb, "List of other contexts to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "contextXml", 2 );
append( sb, "Location of a context xml configuration file whose contents will be applied to the webapp AFTER anything in .Optional.", 3 );
append( sb, "", 0 );
append( sb, "daemon (Default: false)", 2 );
append( sb, "Determines whether or not the server blocks when started. The default behavior (daemon = false) will cause the server to pause other processes while it continues to handle web requests. This is useful when starting the server with the intent to work with it interactively.\n\nOften, it is desirable to let the server start and continue running subsequent processes in an automated build environment. This can be facilitated by setting daemon to true.\n", 3 );
append( sb, "Expression: ${jetty.daemon}", 3 );
append( sb, "", 0 );
append( sb, "excludedGoals", 2 );
append( sb, "List of goals that are NOT to be used", 3 );
append( sb, "", 0 );
append( sb, "jettyXml", 2 );
append( sb, "Comma separated list of a jetty xml configuration files whose contents will be applied before any plugin configuration. Optional.", 3 );
append( sb, "", 0 );
append( sb, "loginServices", 2 );
append( sb, "List of security realms to set up. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "reload (Default: automatic)", 2 );
append( sb, "reload can be set to either \'automatic\' or \'manual\' if \'manual\' then the context can be reloaded by a linefeed in the console if \'automatic\' then traditional reloading on changed files is enabled.", 3 );
append( sb, "Expression: ${jetty.reload}", 3 );
append( sb, "", 0 );
append( sb, "requestLog", 2 );
append( sb, "A RequestLog implementation to use for the webapp at runtime. Consider using instead the element to specify external jetty xml config file. Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanIntervalSeconds (Default: 0)", 2 );
append( sb, "The interval in seconds to scan the webapp for changes and restart the context if necessary. Ignored if reload is enabled. Disabled by default.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${jetty.scanIntervalSeconds}", 3 );
append( sb, "", 0 );
append( sb, "scanTargetPatterns", 2 );
append( sb, "List of directories with ant-style and patterns for extra targets to periodically scan for changes. Can be used instead of, or in conjunction with .Optional.", 3 );
append( sb, "", 0 );
append( sb, "scanTargets", 2 );
append( sb, "List of files or directories to additionally periodically scan for changes. Optional.", 3 );
append( sb, "", 0 );
append( sb, "skip (Default: false)", 2 );
append( sb, "Skip this mojo execution.", 3 );
append( sb, "Expression: ${jetty.skip}", 3 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on executing -DSTOP.PORT= -DSTOP.KEY= -jar start.jar --stop", 3 );
append( sb, "", 0 );
append( sb, "systemProperties", 2 );
append( sb, "System properties to set before execution. Note that these properties will NOT override System properties that have been set on the command line or by the JVM. They WILL override System properties that have been set via systemPropertiesFile. Optional.", 3 );
append( sb, "", 0 );
append( sb, "systemPropertiesFile", 2 );
append( sb, "File containing system properties to be set before execution Note that these properties will NOT override System properties that have been set on the command line, by the JVM, or directly in the POM via systemProperties. Optional.", 3 );
append( sb, "Expression: ${jetty.systemPropertiesFile}", 3 );
append( sb, "", 0 );
append( sb, "testClassesDirectory", 2 );
append( sb, "The directory containing generated test classes.", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "Expression: ${project.build.testOutputDirectory}", 3 );
append( sb, "", 0 );
append( sb, "useProvidedScope (Default: false)", 2 );
append( sb, "Whether or not to include dependencies on the plugin\'s classpath with provided Use WITH CAUTION as you may wind up with duplicate jars/classes.", 3 );
append( sb, "", 0 );
append( sb, "useTestScope (Default: false)", 2 );
append( sb, "If true, the and the dependencies of test will be put first on the runtime classpath.", 3 );
append( sb, "", 0 );
append( sb, "webApp", 2 );
append( sb, "An instance of org.eclipse.jetty.webapp.WebAppContext that represents the webapp. Use any of its setters to configure the webapp. This is the preferred and most flexible method of configuration, rather than using the (deprecated) individual parameters like \'tmpDirectory\', \'contextPath\' etc.", 3 );
append( sb, "", 0 );
append( sb, "webAppSourceDirectory", 2 );
append( sb, "Root directory for all html/jsp etc files", 3 );
append( sb, "Expression: ${maven.war.src}", 3 );
append( sb, "", 0 );
}
}
if ( goal == null || goal.length() <= 0 || "stop".equals( goal ) )
{
append( sb, "jetty:stop", 0 );
append( sb, "JettyStopMojo - stops a running instance of jetty. The ff are required: -DstopKey=someKey -DstopPort=somePort", 1 );
append( sb, "", 0 );
if ( detail )
{
append( sb, "Available parameters:", 1 );
append( sb, "", 0 );
append( sb, "stopKey", 2 );
append( sb, "Key to provide when stopping jetty on executing java -DSTOP.KEY= -DSTOP.PORT= -jar start.jar --stop", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
append( sb, "stopPort", 2 );
append( sb, "Port to listen to stop jetty on sending stop command", 3 );
append( sb, "Required: Yes", 3 );
append( sb, "", 0 );
}
}
if ( getLog().isInfoEnabled() )
{
getLog().info( sb.toString() );
}
}
/**
* Repeat a String n
times to form a new string.
*
* @param str String to repeat
* @param repeat number of times to repeat str
* @return String with repeated String
* @throws NegativeArraySizeException if repeat < 0
* @throws NullPointerException if str is null
*/
private static String repeat( String str, int repeat )
{
StringBuffer buffer = new StringBuffer( repeat * str.length() );
for ( int i = 0; i < repeat; i++ )
{
buffer.append( str );
}
return buffer.toString();
}
/**
* Append a description to the buffer by respecting the indentSize and lineLength parameters.
* Note: The last character is always a new line.
*
* @param sb The buffer to append the description, not null
.
* @param description The description, not null
.
* @param indent The base indentation level of each line, must not be negative.
*/
private void append( StringBuffer sb, String description, int indent )
{
for ( Iterator it = toLines( description, indent, indentSize, lineLength ).iterator(); it.hasNext(); )
{
sb.append( it.next().toString() ).append( '\n' );
}
}
/**
* Splits the specified text into lines of convenient display length.
*
* @param text The text to split into lines, must not be null
.
* @param indent The base indentation level of each line, must not be negative.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
* @return The sequence of display lines, never null
.
* @throws NegativeArraySizeException if indent < 0
*/
private static List toLines( String text, int indent, int indentSize, int lineLength )
{
List lines = new ArrayList();
String ind = repeat( "\t", indent );
String[] plainLines = text.split( "(\r\n)|(\r)|(\n)" );
for ( int i = 0; i < plainLines.length; i++ )
{
toLines( lines, ind + plainLines[i], indentSize, lineLength );
}
return lines;
}
/**
* Adds the specified line to the output sequence, performing line wrapping if necessary.
*
* @param lines The sequence of display lines, must not be null
.
* @param line The line to add, must not be null
.
* @param indentSize The size of each indentation, must not be negative.
* @param lineLength The length of the line, must not be negative.
*/
private static void toLines( List lines, String line, int indentSize, int lineLength )
{
int lineIndent = getIndentLevel( line );
StringBuffer buf = new StringBuffer( 256 );
String[] tokens = line.split( " +" );
for ( int i = 0; i < tokens.length; i++ )
{
String token = tokens[i];
if ( i > 0 )
{
if ( buf.length() + token.length() >= lineLength )
{
lines.add( buf.toString() );
buf.setLength( 0 );
buf.append( repeat( " ", lineIndent * indentSize ) );
}
else
{
buf.append( ' ' );
}
}
for ( int j = 0; j < token.length(); j++ )
{
char c = token.charAt( j );
if ( c == '\t' )
{
buf.append( repeat( " ", indentSize - buf.length() % indentSize ) );
}
else if ( c == '\u00A0' )
{
buf.append( ' ' );
}
else
{
buf.append( c );
}
}
}
lines.add( buf.toString() );
}
/**
* Gets the indentation level of the specified line.
*
* @param line The line whose indentation level should be retrieved, must not be null
.
* @return The indentation level of the line.
*/
private static int getIndentLevel( String line )
{
int level = 0;
for ( int i = 0; i < line.length() && line.charAt( i ) == '\t'; i++ )
{
level++;
}
for ( int i = level + 1; i <= level + 4 && i < line.length(); i++ )
{
if ( line.charAt( i ) == '\t' )
{
level++;
break;
}
}
return level;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy