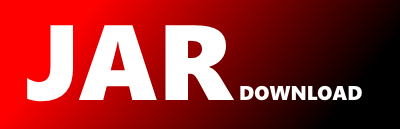
org.mortbay.jetty.runner.Runner Maven / Gradle / Ivy
// ========================================================================
// Copyright 2008 Mort Bay Consulting Pty. Ltd.
// ------------------------------------------------------------------------
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
// http://www.apache.org/licenses/LICENSE-2.0
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// ========================================================================
package org.mortbay.jetty.runner;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Properties;
import java.util.Random;
import javax.transaction.UserTransaction;
import org.eclipse.jetty.util.security.Constraint;
import org.eclipse.jetty.plus.jndi.Transaction;
import org.eclipse.jetty.server.Connector;
import org.eclipse.jetty.server.Handler;
import org.eclipse.jetty.server.NCSARequestLog;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.server.ShutdownMonitor;
import org.eclipse.jetty.server.handler.ContextHandler;
import org.eclipse.jetty.server.handler.ContextHandlerCollection;
import org.eclipse.jetty.server.handler.DefaultHandler;
import org.eclipse.jetty.server.handler.HandlerCollection;
import org.eclipse.jetty.server.handler.RequestLogHandler;
import org.eclipse.jetty.server.handler.StatisticsHandler;
import org.eclipse.jetty.server.nio.SelectChannelConnector;
import org.eclipse.jetty.server.session.SessionHandler;
import org.eclipse.jetty.security.ConstraintMapping;
import org.eclipse.jetty.security.ConstraintSecurityHandler;
import org.eclipse.jetty.security.HashLoginService;
import org.eclipse.jetty.security.authentication.BasicAuthenticator;
import org.eclipse.jetty.servlet.ServletContextHandler;
import org.eclipse.jetty.servlet.ServletHolder;
import org.eclipse.jetty.servlet.StatisticsServlet;
import org.eclipse.jetty.util.RolloverFileOutputStream;
import org.eclipse.jetty.util.log.Log;
import org.eclipse.jetty.util.log.Logger;
import org.eclipse.jetty.util.resource.Resource;
import org.eclipse.jetty.webapp.WebAppContext;
import org.eclipse.jetty.xml.XmlConfiguration;
public class Runner
{
private static final Logger LOG = Log.getLogger(Runner.class);
public static final String[] __plusConfigurationClasses = new String[] {
org.eclipse.jetty.webapp.WebInfConfiguration.class.getCanonicalName(),
org.eclipse.jetty.webapp.WebXmlConfiguration.class.getCanonicalName(),
org.eclipse.jetty.webapp.MetaInfConfiguration.class.getCanonicalName(),
org.eclipse.jetty.webapp.FragmentConfiguration.class.getCanonicalName(),
org.eclipse.jetty.plus.webapp.EnvConfiguration.class.getCanonicalName(),
org.eclipse.jetty.plus.webapp.PlusConfiguration.class.getCanonicalName(),
org.eclipse.jetty.annotations.AnnotationConfiguration.class.getCanonicalName(),
org.eclipse.jetty.webapp.JettyWebXmlConfiguration.class.getCanonicalName(),
org.eclipse.jetty.webapp.TagLibConfiguration.class.getCanonicalName()
};
public static final String __containerIncludeJarPattern = ".*/.*jsp-api-[^/]*\\.jar$|.*/.*jsp-[^/]*\\.jar$|.*/.*taglibs[^/]*\\.jar$|.*/.*jstl[^/]*\\.jar$|.*/.*jsf-impl-[^/]*\\.jar$|.*/.*javax.faces-[^/]*\\.jar$|.*/.*myfaces-impl-[^/]*\\.jar$|.*/.*jetty-runner-[^/]*\\.jar$";
protected Server _server;
protected Monitor _monitor;
protected URLClassLoader _classLoader;
protected List _classpath=new ArrayList();
protected ContextHandlerCollection _contexts;
protected RequestLogHandler _logHandler;
protected String _logFile;
protected String _configFile;
protected UserTransaction _ut;
protected String _utId;
protected String _txMgrPropertiesFile;
protected Random _random = new Random();
protected boolean _isTxServiceAvailable=false;
protected boolean _enableStatsGathering=false;
protected String _statsPropFile;
protected boolean _clusteredSessions=true;
public Runner()
{
}
public void usage(String error)
{
if (error!=null)
System.err.println("ERROR: "+error);
System.err.println("Usage: java [-DDEBUG] [-Djetty.home=dir] -jar jetty-runner.jar [--help|--version] [ server opts] [[ context opts] context ...] ");
System.err.println("Server Options:");
System.err.println(" --version - display version and exit");
System.err.println(" --log file - request log filename (with optional 'yyyy_mm_dd' wildcard");
System.err.println(" --out file - info/warn/debug log filename (with optional 'yyyy_mm_dd' wildcard");
System.err.println(" --port n - port to listen on (default 8080)");
System.err.println(" --stop-port n - port to listen for stop command");
System.err.println(" --stop-key n - security string for stop command (required if --stop-port is present)");
System.err.println(" --jar file - a jar to be added to the classloader");
System.err.println(" --jdbc classname properties jndiname - classname of XADataSource or driver; properties string; name to register in jndi");
System.err.println(" --lib dir - a directory of jars to be added to the classloader");
System.err.println(" --classes dir - a directory of classes to be added to the classloader");
System.err.println(" --txFile - override properties file for Atomikos");
System.err.println(" --stats [unsecure|realm.properties] - enable stats gathering servlet context");
System.err.println(" --config file - a jetty xml config file to use instead of command line options");
System.err.println("Context Options:");
System.err.println(" --path /path - context path (default /)");
System.err.println(" context - WAR file, web app dir or context.xml file");
System.exit(1);
}
public void configure(String[] args) throws Exception
{
// handle classpath bits first so we can initialize the log mechanism.
for (int i=0;i 0 ? 1 : 0) + (stopKey != null ? 2 : 0))
{
case 1:
usage("Must specify --stop-key when --stop-port is specified");
break;
case 2:
usage("Must specify --stop-port when --stop-key is specified");
break;
case 3:
ShutdownMonitor monitor = ShutdownMonitor.getInstance();
monitor.setPort(stopPort);
monitor.setKey(stopKey);
monitor.setExitVm(true);
break;
}
if (_logFile!=null)
{
NCSARequestLog requestLog = new NCSARequestLog(_logFile);
requestLog.setExtended(false);
_logHandler.setRequestLog(requestLog);
}
}
protected void prependHandler (Handler handler, HandlerCollection handlers)
{
if (handler == null || handlers == null)
return;
Handler[] existing = handlers.getChildHandlers();
Handler[] children = new Handler[existing.length + 1];
children[0] = handler;
System.arraycopy(existing, 0, children, 1, existing.length);
handlers.setHandlers(children);
}
protected int configJDBC(String[] args,int i) throws Exception
{
String jdbcClass=null;
String jdbcProperties=null;
String jdbcJndiName=null;
if (!_isTxServiceAvailable)
{
LOG.warn("JDBC TX support not found on classpath");
i+=3;
}
else
{
jdbcClass=args[++i];
jdbcProperties=args[++i];
jdbcJndiName=args[++i];
//check for jdbc resources to register
if (jdbcClass!=null)
{
if (isXADataSource(jdbcClass))
{
Class simpleDataSourceBeanClass = Thread.currentThread().getContextClassLoader().loadClass("com.atomikos.jdbc.SimpleDataSourceBean");
Object o = simpleDataSourceBeanClass.newInstance();
simpleDataSourceBeanClass.getMethod("setXaDataSourceClassName", new Class[] {String.class}).invoke(o, new Object[] {jdbcClass});
simpleDataSourceBeanClass.getMethod("setXaDataSourceProperties", new Class[] {String.class}).invoke(o, new Object[] {jdbcProperties});
simpleDataSourceBeanClass.getMethod("setUniqueResourceName", new Class[] {String.class}).invoke(o, new Object[] {jdbcJndiName});
org.eclipse.jetty.plus.jndi.Resource jdbcResource = new org.eclipse.jetty.plus.jndi.Resource(jdbcJndiName, o);
}
else
{
String[] props = jdbcProperties.split(";");
String user=null;
String password=null;
String url=null;
for (int j=0;props!=null && j0)
{
ClassLoader context=Thread.currentThread().getContextClassLoader();
if (context==null)
_classLoader=new URLClassLoader(_classpath.toArray(new URL[_classpath.size()]));
else
_classLoader=new URLClassLoader(_classpath.toArray(new URL[_classpath.size()]),context);
Thread.currentThread().setContextClassLoader(_classLoader);
}
}
protected boolean isXADataSource (String classname)
throws Exception
{
Class clazz = Thread.currentThread().getContextClassLoader().loadClass(classname);
boolean isXA=false;
while (!isXA && clazz!=null)
{
Class[] interfaces = clazz.getInterfaces();
for (int i=0;interfaces!=null &&!isXA && i0&&args[0].equalsIgnoreCase("--help"))
{
runner.usage(null);
}
else if (args.length>0&&args[0].equalsIgnoreCase("--version"))
{
System.err.println("org.mortbay.jetty.Runner: "+Server.getVersion());
System.exit(1);
}
runner.configure(args);
runner.run();
}
catch (Exception e)
{
e.printStackTrace();
runner.usage(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy