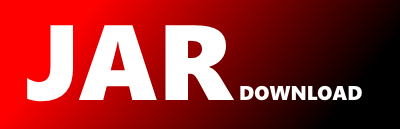
org.mortbay.jetty.plugin.AbstractJettyRunMojo Maven / Gradle / Ivy
//========================================================================
//$Id: AbstractJettyRunMojo.java 5224 2009-05-29 07:56:29Z dyu $
//Copyright 2000-2004 Mort Bay Consulting Pty. Ltd.
//------------------------------------------------------------------------
//Licensed under the Apache License, Version 2.0 (the "License");
//you may not use this file except in compliance with the License.
//You may obtain a copy of the License at
//http://www.apache.org/licenses/LICENSE-2.0
//Unless required by applicable law or agreed to in writing, software
//distributed under the License is distributed on an "AS IS" BASIS,
//WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
//See the License for the specific language governing permissions and
//limitations under the License.
//========================================================================
package org.mortbay.jetty.plugin;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.codehaus.plexus.util.FileUtils;
import org.mortbay.jetty.plugin.util.ScanTargetPattern;
import org.mortbay.resource.Resource;
import org.mortbay.resource.ResourceCollection;
import org.mortbay.util.Scanner;
/**
* AbstractJettyRunMojo
*
*
* Base class for all jetty versions for the "run" mojo.
*
*/
public abstract class AbstractJettyRunMojo extends AbstractJettyMojo
{
/**
* If true, the <testOutputDirectory>
* and the dependencies of <scope>test<scope>
* will be put first on the runtime classpath.
* @parameter default-value="false"
*/
private boolean useTestClasspath;
/**
* The location of a jetty-env.xml file. Optional.
* @parameter
*/
private File jettyEnvXml;
/**
* The location of the web.xml file. If not
* set then it is assumed it is in ${basedir}/src/main/webapp/WEB-INF
*
* @parameter expression="${maven.war.webxml}"
*/
private File webXml;
/**
* The directory containing generated classes.
*
* @parameter expression="${project.build.outputDirectory}"
* @required
*
*/
private File classesDirectory;
/**
* The directory containing generated test classes.
*
* @parameter expression="${project.build.testOutputDirectory}"
* @required
*/
private File testClassesDirectory;
/**
* Root directory for all html/jsp etc files
*
* @parameter expression="${basedir}/src/main/webapp"
* @required
*/
private File webAppSourceDirectory;
/**
* @parameter expression="${plugin.artifacts}"
* @readonly
*/
private List pluginArtifacts;
/**
* List of files or directories to additionally periodically scan for changes. Optional.
* @parameter
*/
private File[] scanTargets;
/**
* List of directories with ant-style <include> and <exclude> patterns
* for extra targets to periodically scan for changes. Can be used instead of,
* or in conjunction with <scanTargets>.Optional.
* @parameter
*/
private ScanTargetPattern[] scanTargetPatterns;
/**
* web.xml as a File
*/
private File webXmlFile;
/**
* jetty-env.xml as a File
*/
private File jettyEnvXmlFile;
/**
* List of files on the classpath for the webapp
*/
private List classPathFiles;
/**
* Extra scan targets as a list
*/
private List extraScanTargets;
/**
* overlays (resources)
*/
private List _overlays;
public File getWebXml()
{
return this.webXml;
}
public File getJettyEnvXml ()
{
return this.jettyEnvXml;
}
public File getClassesDirectory()
{
return this.classesDirectory;
}
public File getWebAppSourceDirectory()
{
return this.webAppSourceDirectory;
}
public void setWebXmlFile (File f)
{
this.webXmlFile = f;
}
public File getWebXmlFile ()
{
return this.webXmlFile;
}
public File getJettyEnvXmlFile ()
{
return this.jettyEnvXmlFile;
}
public void setJettyEnvXmlFile (File f)
{
this.jettyEnvXmlFile = f;
}
public void setClassPathFiles (List list)
{
this.classPathFiles = new ArrayList(list);
}
public List getClassPathFiles ()
{
return this.classPathFiles;
}
public List getExtraScanTargets ()
{
return this.extraScanTargets;
}
public void setExtraScanTargets(List list)
{
this.extraScanTargets = list;
}
/**
* Run the mojo
* @see org.apache.maven.plugin.Mojo#execute()
*/
public void execute() throws MojoExecutionException, MojoFailureException
{
super.execute();
}
/**
* Verify the configuration given in the pom.
*
* @see org.mortbay.jetty.plugin.AbstractJettyMojo#checkPomConfiguration()
*/
public void checkPomConfiguration () throws MojoExecutionException
{
File buildDir = new File(getProject().getBuild().getDirectory());
if(!buildDir.exists())
buildDir.mkdir();
// check the location of the static content/jsps etc
try
{
if ((getWebAppSourceDirectory() == null) || !getWebAppSourceDirectory().exists())
throw new MojoExecutionException("Webapp source directory "
+ (getWebAppSourceDirectory() == null ? "null" : getWebAppSourceDirectory().getCanonicalPath())
+ " does not exist");
else
getLog().info( "Webapp source directory = "
+ getWebAppSourceDirectory().getCanonicalPath());
}
catch (IOException e)
{
throw new MojoExecutionException("Webapp source directory does not exist", e);
}
// check reload mechanic
if ( !"automatic".equalsIgnoreCase( reload ) && !"manual".equalsIgnoreCase( reload ) )
{
throw new MojoExecutionException( "invalid reload mechanic specified, must be 'automatic' or 'manual'" );
}
else
{
getLog().info("Reload Mechanic: " + reload );
}
//check if a jetty-env.xml location has been provided, if so, it must exist
if (getJettyEnvXml() != null)
{
setJettyEnvXmlFile(jettyEnvXml);
try
{
if (!getJettyEnvXmlFile().exists())
throw new MojoExecutionException("jetty-env.xml file does not exist at location "+jettyEnvXml);
else
getLog().info(" jetty-env.xml = "+getJettyEnvXmlFile().getCanonicalPath());
}
catch (IOException e)
{
throw new MojoExecutionException("jetty-env.xml does not exist");
}
}
// check the classes to form a classpath with
try
{
//allow a webapp with no classes in it (just jsps/html)
if (getClassesDirectory() != null)
{
if (!getClassesDirectory().exists())
getLog().info( "Classes directory "+ getClassesDirectory().getCanonicalPath()+ " does not exist");
else
getLog().info("Classes = " + getClassesDirectory().getCanonicalPath());
}
else
getLog().info("Classes directory not set");
}
catch (IOException e)
{
throw new MojoExecutionException("Location of classesDirectory does not exist");
}
setExtraScanTargets(new ArrayList());
if (scanTargets != null)
{
for (int i=0; i< scanTargets.length; i++)
{
getLog().info("Added extra scan target:"+ scanTargets[i]);
getExtraScanTargets().add(scanTargets[i]);
}
}
if (scanTargetPatterns!=null)
{
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy