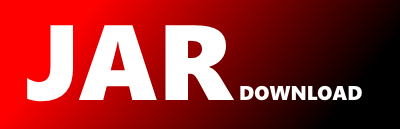
org.moskito.central.HashMapAdapter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moskito-central-core Show documentation
Show all versions of moskito-central-core Show documentation
shared stuff between all projects.
package org.moskito.central;
import org.moskito.central.HashMapAdapter.MyMapType;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.adapters.XmlAdapter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map.Entry;
/**
*
* @author dagafonov
*
* @param
* @param
*/
public class HashMapAdapter extends XmlAdapter, HashMap> {
/**
* Default constructor.
*/
public HashMapAdapter() {
}
@Override
public MyMapType marshal(HashMap arg0) {
MyMapType myMapType = new MyMapType();
for (Entry entry : arg0.entrySet()) {
MyMapEntryType myMapEntryType = new MyMapEntryType();
myMapEntryType.key = entry.getKey();
myMapEntryType.value = entry.getValue();
myMapType.entries.add(myMapEntryType);
}
return myMapType;
}
@Override
public HashMap unmarshal(MyMapType arg0) {
HashMap hashMap = new HashMap();
for (MyMapEntryType myEntryType : arg0.entries) {
hashMap.put(myEntryType.key, myEntryType.value);
}
return hashMap;
}
/**
* Wrapper for all entries that stored in hash map.
*
* @author dagafonov
*
* @param
* @param
*/
@XmlRootElement
public static class MyMapType {
/**
* All keys and values will be transformed into {@link MyMapEntryType}.
*/
private List> entries = new ArrayList>();
public List> getEntries() {
return entries;
}
public void setEntries(List> entries) {
this.entries = entries;
}
@Override
public String toString() {
return "MyMapType [entries=" + entries + "]";
}
}
/**
* Wrapper for key - value pair.
*
* @author dagafonov
*
* @param
* @param
*/
@XmlRootElement
public static class MyMapEntryType {
/**
* Entry key.
*/
private K key;
/**
* Entry value.
*/
private V value;
public K getKey() {
return key;
}
public void setKey(K key) {
this.key = key;
}
public V getValue() {
return value;
}
public void setValue(V value) {
this.value = value;
}
@Override
public String toString() {
return "MyMapEntryType [key=" + key + ", value=" + value + "]";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy