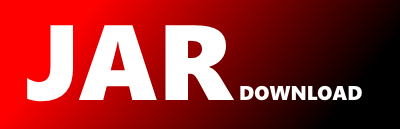
rhino1.7.6.examples.Matrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rhino Show documentation
Show all versions of rhino Show documentation
Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically
embedded into Java applications to provide scripting to end users.
/* -*- Mode: java; tab-width: 8; indent-tabs-mode: nil; c-basic-offset: 4 -*-
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import org.mozilla.javascript.*;
import java.util.List;
import java.util.ArrayList;
/**
* Matrix: An example host object class that implements the Scriptable interface.
*
* Built-in JavaScript arrays don't handle multiple dimensions gracefully: the
* script writer must create every array in an array of arrays. The Matrix class
* takes care of that by automatically allocating arrays for every index that
* is accessed. What's more, the Matrix constructor takes a integer argument
* that specifies the dimension of the Matrix. If m is a Matrix with dimension 3,
* then m[0] will be a Matrix with dimension 1, and m[0][0] will be an Array.
*
* Here's a shell session showing the Matrix object in action:
*
* js> defineClass("Matrix")
* js> var m = new Matrix(2); // A constructor call, see "Matrix(int dimension)"
* js> m // Object.toString will call "Matrix.getClassName()"
* [object Matrix]
* js> m[0][0] = 3;
* 3
* js> uneval(m[0]); // an array was created automatically!
* [3]
* js> uneval(m[1]); // array is created even if we don't set a value
* []
* js> m.dim; // we can access the "dim" property
* 2
* js> m.dim = 3;
* 3
* js> m.dim; // but not modify the "dim" property
* 2
*
*
* @see org.mozilla.javascript.Context
* @see org.mozilla.javascript.Scriptable
*
* @author Norris Boyd
*/
public class Matrix implements Scriptable {
/**
* The zero-parameter constructor.
*
* When ScriptableObject.defineClass is called with this class, it will
* construct Matrix.prototype using this constructor.
*/
public Matrix() {
}
/**
* The Java constructor, also used to define the JavaScript constructor.
*/
public Matrix(int dimension) {
if (dimension <= 0) {
throw Context.reportRuntimeError(
"Dimension of Matrix must be greater than zero");
}
dim = dimension;
list = new ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy