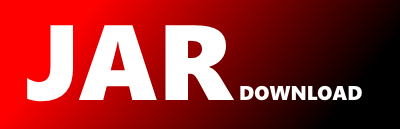
rhino1.7.7.examples.RunScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rhino Show documentation
Show all versions of rhino Show documentation
Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically
embedded into Java applications to provide scripting to end users.
/* -*- Mode: java; tab-width: 8; indent-tabs-mode: nil; c-basic-offset: 4 -*-
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import org.mozilla.javascript.*;
/**
* RunScript: simplest example of controlling execution of Rhino.
*
* Collects its arguments from the command line, executes the
* script, and prints the result.
*
* @author Norris Boyd
*/
public class RunScript {
public static void main(String args[])
{
// Creates and enters a Context. The Context stores information
// about the execution environment of a script.
Context cx = Context.enter();
try {
// Initialize the standard objects (Object, Function, etc.)
// This must be done before scripts can be executed. Returns
// a scope object that we use in later calls.
Scriptable scope = cx.initStandardObjects();
// Collect the arguments into a single string.
String s = "";
for (int i=0; i < args.length; i++) {
s += args[i];
}
// Now evaluate the string we've colected.
Object result = cx.evaluateString(scope, s, "", 1, null);
// Convert the result to a string and print it.
System.err.println(Context.toString(result));
} finally {
// Exit from the context.
Context.exit();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy