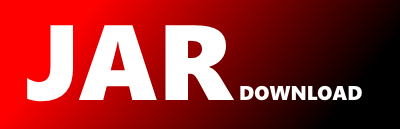
rhino1.7.7.testsrc.org.mozilla.javascript.tests.DoctestsTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rhino Show documentation
Show all versions of rhino Show documentation
Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically
embedded into Java applications to provide scripting to end users.
/* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
package org.mozilla.javascript.tests;
import java.io.File;
import java.io.FileFilter;
import java.io.FileReader;
import java.io.IOException;
import java.util.*;
import org.junit.*;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import org.junit.runners.Parameterized.Parameters;
import org.mozilla.javascript.Context;
import org.mozilla.javascript.ContextFactory;
import org.mozilla.javascript.drivers.TestUtils;
import org.mozilla.javascript.tools.shell.Global;
import static org.junit.Assert.*;
/**
* Run doctests in folder testsrc/doctests.
*
* A doctest is a test in the form of an interactive shell session; Rhino
* collects and runs the inputs to the shell prompt and compares them to the
* expected outputs.
*
* @author Norris Boyd
*/
@RunWith(Parameterized.class)
public class DoctestsTest {
static final String baseDirectory = "testsrc" + File.separator + "doctests";
static final String doctestsExtension = ".doctest";
String name;
String source;
int optimizationLevel;
public DoctestsTest(String name, String source, int optimizationLevel) {
this.name = name;
this.source = source;
this.optimizationLevel = optimizationLevel;
}
public static File[] getDoctestFiles() {
return TestUtils.recursiveListFiles(new File(baseDirectory),
new FileFilter() {
public boolean accept(File f) {
return f.getName().endsWith(doctestsExtension);
}
});
}
public static String loadFile(File f) throws IOException {
int length = (int) f.length(); // don't worry about very long files
char[] buf = new char[length];
new FileReader(f).read(buf, 0, length);
return new String(buf);
}
@Parameters
public static Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy