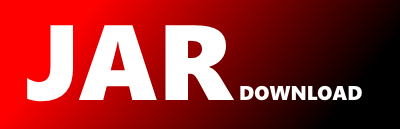
rhino1.7.7.testsrc.org.mozilla.javascript.tests.MozillaSuiteTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rhino Show documentation
Show all versions of rhino Show documentation
Rhino is an open-source implementation of JavaScript written entirely in Java. It is typically
embedded into Java applications to provide scripting to end users.
/* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
package org.mozilla.javascript.tests;
import java.io.File;
import java.io.FileFilter;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.PrintStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import junit.framework.Assert;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import org.junit.runners.Parameterized.Parameters;
import org.mozilla.javascript.drivers.JsTestsBase;
import org.mozilla.javascript.drivers.ShellTest;
import org.mozilla.javascript.drivers.TestUtils;
import org.mozilla.javascript.tools.shell.ShellContextFactory;
/**
* This JUnit suite runs the Mozilla test suite (in mozilla.org CVS
* at /mozilla/js/tests).
*
* Not all tests in the suite are run. Since the mozilla.org tests are
* designed and maintained for the SpiderMonkey engine, tests in the
* suite may not pass due to feature set differences and known bugs.
* To make sure that this unit test is stable in the midst of changes
* to the mozilla.org suite, we maintain a list of passing tests in
* files opt-1.tests, opt0.tests, and opt9.tests. This class also
* implements the ability to run skipped tests, see if any pass, and
* print out a script to modify the *.tests files.
* (This approach doesn't handle breaking changes to existing passing
* tests, but in practice that has been very rare.)
* @author Norris Boyd
* @author Attila Szegedi
*/
@RunWith(Parameterized.class)
public class MozillaSuiteTest {
private final File jsFile;
private final int optimizationLevel;
static final int[] OPT_LEVELS = { -1, 0, 9 };
public MozillaSuiteTest(File jsFile, int optimizationLevel) {
this.jsFile = jsFile;
this.optimizationLevel = optimizationLevel;
}
public static File getTestDir() throws IOException {
File testDir = null;
if (System.getProperty("mozilla.js.tests") != null) {
testDir = new File(System.getProperty("mozilla.js.tests"));
} else {
URL url = JsTestsBase.class.getResource(".");
String path = url.getFile();
int jsIndex = path.lastIndexOf("/js");
if (jsIndex == -1) {
throw new IllegalStateException("You aren't running the tests "+
"from within the standard mozilla/js directory structure");
}
path = path.substring(0, jsIndex + 3).replace('/', File.separatorChar);
path = path.replace("%20", " ");
testDir = new File(path, "tests");
}
if (!testDir.isDirectory()) {
throw new FileNotFoundException(testDir + " is not a directory");
}
return testDir;
}
public static String getTestFilename(int optimizationLevel) {
return "opt" + optimizationLevel + ".tests";
}
public static File[] getTestFiles(int optimizationLevel) throws IOException {
File testDir = getTestDir();
String[] tests = TestUtils.loadTestsFromResource(
"/" + getTestFilename(optimizationLevel), null);
Arrays.sort(tests);
File[] files = new File[tests.length];
for (int i=0; i < files.length; i++) {
files[i] = new File(testDir, tests[i]);
}
return files;
}
public static String loadFile(File f) throws IOException {
int length = (int) f.length(); // don't worry about very long files
char[] buf = new char[length];
new FileReader(f).read(buf, 0, length);
return new String(buf);
}
@Parameters(name = "{index}, js={0}, opt={1}")
public static Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy