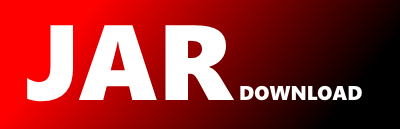
org.mule.config.spring.CompositeOptionalObjectsController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mule-module-spring-config Show documentation
Show all versions of mule-module-spring-config Show documentation
Mule Builder for use with Spring 2.X Namespace based XML
configuration.
/*
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.config.spring;
import static org.mule.util.Preconditions.checkArgument;
import org.mule.util.ArrayUtils;
import com.google.common.collect.ImmutableList;
import java.util.Collection;
import java.util.List;
/**
* Implementation of {@link OptionalObjectsController} which groups
* a list of controllers and executes every operation on all of them.
*
* @since 3.7.0
*/
public class CompositeOptionalObjectsController implements OptionalObjectsController
{
private final List controllers;
public CompositeOptionalObjectsController(OptionalObjectsController... controllers)
{
checkArgument(!ArrayUtils.isEmpty(controllers), "cannot compose empty controllers list");
this.controllers = ImmutableList.copyOf(controllers);
}
@Override
public void registerOptionalKey(String key)
{
for (OptionalObjectsController controller : controllers)
{
controller.registerOptionalKey(key);
}
}
@Override
public void discardOptionalObject(String key)
{
for (OptionalObjectsController controller : controllers)
{
controller.discardOptionalObject(key);
}
}
@Override
public boolean isOptional(String key)
{
for (OptionalObjectsController controller : controllers)
{
if (controller.isOptional(key))
{
return true;
}
}
return false;
}
@Override
public boolean isDiscarded(String key)
{
for (OptionalObjectsController controller : controllers)
{
if (controller.isDiscarded(key))
{
return true;
}
}
return false;
}
@Override
public Object getDiscardedObjectPlaceholder()
{
for (OptionalObjectsController controller : controllers)
{
Object placeHolder = controller.getDiscardedObjectPlaceholder();
if (placeHolder != null)
{
return placeHolder;
}
}
return null;
}
@Override
public Collection getAllOptionalKeys()
{
ImmutableList.Builder builder = ImmutableList.builder();
for (OptionalObjectsController controller : controllers)
{
builder.addAll(controller.getAllOptionalKeys());
}
return builder.build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy