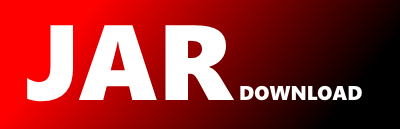
org.mule.config.spring.parsers.specific.RetryPolicyDefinitionParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mule-module-spring-config Show documentation
Show all versions of mule-module-spring-config Show documentation
Mule Builder for use with Spring 2.X Namespace based XML
configuration.
The newest version!
/*
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.config.spring.parsers.specific;
import static org.mule.api.config.MuleProperties.OBJECT_DEFAULT_RETRY_POLICY_TEMPLATE;
import static org.mule.api.config.MuleProperties.OBJECT_MULE_CONFIGURATION;
import org.mule.config.spring.parsers.generic.OptionalChildDefinitionParser;
import org.mule.retry.async.AsynchronousRetryTemplate;
import org.apache.commons.lang.StringUtils;
import org.springframework.beans.factory.config.BeanDefinition;
import org.springframework.beans.factory.config.BeanDefinitionHolder;
import org.springframework.beans.factory.config.RuntimeBeanReference;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.xml.ParserContext;
import org.w3c.dom.Element;
/**
* Allows retry policies to be children of connector elements or the element.
*/
public class RetryPolicyDefinitionParser extends OptionalChildDefinitionParser
{
boolean asynchronous = false;
public RetryPolicyDefinitionParser()
{
super("retryPolicyTemplate");
}
public RetryPolicyDefinitionParser(Class clazz)
{
super("retryPolicyTemplate", clazz);
}
@Override
protected boolean isChild(Element element, ParserContext parserContext, BeanDefinitionBuilder builder)
{
if (isConfigElement(element))
{
element.setAttribute(ATTRIBUTE_ID, OBJECT_DEFAULT_RETRY_POLICY_TEMPLATE);
return false;
}
else
{
return true;
}
}
protected boolean isConfigElement(Element element)
{
return getParentBeanName(element).equals(OBJECT_MULE_CONFIGURATION);
}
@Override
protected void preProcess(Element element)
{
super.preProcess(element);
// Is this an asynchronous retry policy?
asynchronous = !Boolean.parseBoolean(element.getAttribute("blocking"));
element.removeAttribute("blocking");
// Deprecated attribute from 2.x kept for backwards-compatibility. Remove for the next major release.
if (StringUtils.isNotEmpty(element.getAttribute("asynchronous")))
{
asynchronous = Boolean.parseBoolean(element.getAttribute("asynchronous"));
element.removeAttribute("asynchronous");
return;
}
}
/**
* The BDP magic inside this method will transform this simple config:
*
*
*
*
*
* into this equivalent config, because of the attribute asynchronous="true":
*
*
*
*
*
*
*
*
*
*
*
*
*
*/
@Override
protected void parseChild(Element element, ParserContext parserContext, BeanDefinitionBuilder builder)
{
super.parseChild(element, parserContext, builder);
if (asynchronous)
{
// Create the AsynchronousRetryTemplate as a wrapper bean
BeanDefinitionBuilder bdb = BeanDefinitionBuilder.genericBeanDefinition(AsynchronousRetryTemplate.class);
if (isConfigElement(element))
{
// rename the delegate policy
element.removeAttribute(ATTRIBUTE_ID);
element.setAttribute(ATTRIBUTE_ID, getBeanName(element));
wrapDelegateRetryPolicy(element, parserContext, bdb, OBJECT_DEFAULT_RETRY_POLICY_TEMPLATE);
}
else
{
// Generate a bean name
String asynchWrapperName = parserContext.getReaderContext().generateBeanName(bdb.getBeanDefinition());
wrapDelegateRetryPolicy(element, parserContext, bdb, asynchWrapperName);
// Set the AsynchronousRetryTemplate wrapper bean on the retry policy's parent instead of the retry
// policy itself
BeanDefinition parent = parserContext.getRegistry().getBeanDefinition(getParentBeanName(element));
parent.getPropertyValues().addPropertyValue(getPropertyName(element), new RuntimeBeanReference(asynchWrapperName));
}
}
}
protected void wrapDelegateRetryPolicy(Element element, ParserContext parserContext, BeanDefinitionBuilder bdb, String asynchWrapperName)
{
// Pass in the retry policy as a constructor argument
bdb.addConstructorArgReference(getBeanName(element));
// Register the new bean
BeanDefinitionHolder holder = new BeanDefinitionHolder(bdb.getBeanDefinition(), asynchWrapperName);
registerBeanDefinition(holder, parserContext.getRegistry());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy