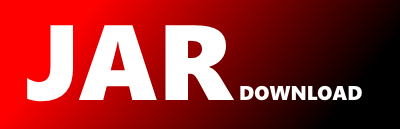
org.mule.runtime.api.metadata.MetadataCache Maven / Gradle / Ivy
/*
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.runtime.api.metadata;
import org.mule.runtime.api.connection.ConnectionException;
import org.mule.runtime.api.metadata.resolving.InputTypeResolver;
import org.mule.runtime.api.metadata.resolving.OutputTypeResolver;
import org.mule.runtime.api.metadata.resolving.TypeKeysResolver;
import java.io.Serializable;
import java.util.Map;
import java.util.Optional;
/**
* This component provides the capability to store data so future requests
* for that data can be served faster and accessed from every {@link InputTypeResolver},
* {@link TypeKeysResolver} and {@link OutputTypeResolver}
*
* @since 1.0
*/
public interface MetadataCache {
/**
* Associates the specified value with the specified key in the cache.
* if the cache previously contained a mapping for the specified key, the old value gets replaced
*
* @param key a key to associate the specified value
* @param value the value to persist in the cache
*/
void put(Serializable key, Serializable value);
/**
* Copies all of the entries from the specified map to the cache.
*
* @param values values to be stored in the cache
*/
void putAll(Map extends Serializable, ? extends Serializable> values);
/**
* @param key the key whose associated value is to be returned
* @return the value to which the specified key is mapped,
* or {@code Option.empty()} if this map contains no value for the specified key.
*/
Optional get(Serializable key);
/**
* If the specified key is not already associated with a value,
* attempts to compute its value using the given mapping function
* and enters it into this map unless {@code null}.
*
* @param key the key whose associated value is to be returned
* @return the current (existing or computed) value associated with
* the specified key, or null if the computed value is null
*/
T computeIfAbsent(Serializable key, MetadataCacheValueResolver mappingFunction)
throws MetadataResolvingException, ConnectionException;
@FunctionalInterface
interface MetadataCacheValueResolver {
Serializable compute(Serializable key) throws MetadataResolvingException, ConnectionException;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy