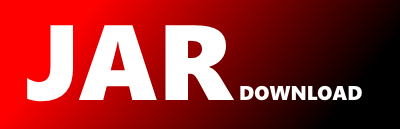
org.mule.routing.WireTap Maven / Gradle / Ivy
/*
* $Id: WireTap.java 20759 2010-12-15 23:30:56Z dfeist $
* --------------------------------------------------------------------------------------
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
*
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.routing;
import org.mule.RequestContext;
import org.mule.api.MuleEvent;
import org.mule.api.MuleException;
import org.mule.api.processor.MessageProcessor;
import org.mule.api.routing.filter.Filter;
import org.mule.processor.AbstractFilteringMessageProcessor;
import org.mule.processor.AbstractMessageProcessorOwner;
import org.mule.util.ObjectUtils;
import java.util.Collections;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* The WireTap
MessageProcessor allows inspection of messages in a flow.
*
* The incoming message is is sent to both the primary and wiretap outputs. The flow
* of the primary output will be unmodified and a copy of the message used for the
* wiretap output.
*
* An optional filter can be used to filter which message are sent to the wiretap
* output, this filter does not affect the flow to the primary output. If there is an
* error sending to the wiretap output no exception will be thrown but rather an
* error logged.
*
* EIP Reference: http://www.eaipatterns.com/WireTap.html
*/
public class WireTap extends AbstractMessageProcessorOwner implements MessageProcessor
{
protected final transient Log logger = LogFactory.getLog(getClass());
protected volatile MessageProcessor tap;
protected volatile Filter filter;
protected MessageProcessor filteredTap = new WireTapFilter();
public MuleEvent process(MuleEvent event) throws MuleException
{
if (tap == null)
{
return event;
}
try
{
// Do we need this?
RequestContext.setEvent(null);
filteredTap.process(RequestContext.setEvent(event));
}
catch (MuleException e)
{
logger.error("Exception sending to wiretap output " + tap, e);
}
return event;
}
public MessageProcessor getTap()
{
return tap;
}
public void setTap(MessageProcessor tap)
{
this.tap = tap;
}
@Deprecated
public void setMessageProcessor(MessageProcessor tap)
{
setTap(tap);
}
public Filter getFilter()
{
return filter;
}
public void setFilter(Filter filter)
{
this.filter = filter;
}
private class WireTapFilter extends AbstractFilteringMessageProcessor
{
@Override
protected boolean accept(MuleEvent event)
{
if (filter == null)
{
return true;
}
else
{
return filter.accept(event.getMessage());
}
}
@Override
protected MuleEvent processNext(MuleEvent event) throws MuleException
{
if (tap != null)
{
tap.process(event);
}
return null;
}
@Override
public String toString()
{
return ObjectUtils.toString(this);
}
}
@Override
public String toString()
{
return ObjectUtils.toString(this);
}
@Override
protected List getOwnedMessageProcessors()
{
return Collections.singletonList(tap);
}
}