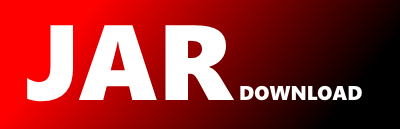
org.mule.exception.RedeliveryExceeded Maven / Gradle / Ivy
/*
* $Id: RedeliveryExceeded.java 23825 2012-02-06 19:30:31Z pablo.lagreca $
* --------------------------------------------------------------------------------------
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
*
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.exception;
import org.mule.api.MuleEvent;
import org.mule.api.MuleException;
import org.mule.api.construct.FlowConstruct;
import org.mule.api.construct.FlowConstructAware;
import org.mule.api.lifecycle.Initialisable;
import org.mule.api.lifecycle.InitialisationException;
import org.mule.api.processor.MessageProcessor;
import org.mule.api.processor.MessageProcessorChain;
import org.mule.processor.AbstractMessageProcessorOwner;
import org.mule.processor.chain.DefaultMessageProcessorChainBuilder;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
public class RedeliveryExceeded implements MessageProcessor, FlowConstructAware, Initialisable
{
private List messageProcessors = new CopyOnWriteArrayList();
private MessageProcessorChain configuredMessageProcessors;
private FlowConstruct flowConstruct;
@Override
public void initialise() throws InitialisationException
{
DefaultMessageProcessorChainBuilder defaultMessageProcessorChainBuilder = new DefaultMessageProcessorChainBuilder(this.flowConstruct);
try
{
configuredMessageProcessors = defaultMessageProcessorChainBuilder.chain(messageProcessors).build();
}
catch (MuleException e)
{
throw new InitialisationException(e, this);
}
}
public List getMessageProcessors()
{
return Collections.unmodifiableList(messageProcessors);
}
public void setMessageProcessors(List processors)
{
if (processors != null)
{
this.messageProcessors.clear();
this.messageProcessors.addAll(processors);
}
else
{
throw new IllegalArgumentException("List of targets = null");
}
}
@Override
public MuleEvent process(MuleEvent event) throws MuleException
{
MuleEvent result = event;
if (!messageProcessors.isEmpty()) {
result = configuredMessageProcessors.process(event);
}
if (result != null)
{
result.getMessage().setExceptionPayload(null);
}
return result;
}
@Override
public void setFlowConstruct(FlowConstruct flowConstruct)
{
this.flowConstruct = flowConstruct;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy