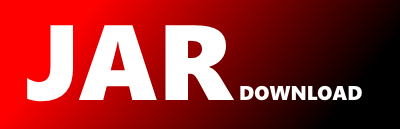
org.mule.maven.client.internal.util.FileUtils Maven / Gradle / Ivy
/*
* Copyright (c) MuleSoft, Inc. All rights reserved. http://www.mulesoft.com
* The software in this package is published under the terms of the CPAL v1.0
* license, a copy of which has been included with this distribution in the
* LICENSE.txt file.
*/
package org.mule.maven.client.internal.util;
import static java.util.Optional.empty;
import static java.util.Optional.of;
import static org.apache.commons.io.IOUtils.toByteArray;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.net.JarURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Optional;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
public class FileUtils {
/**
* Loads the content of a file within a jar into a byte array.
*
* @param jarFile the jar file
* @return the content of the file as byte array or empty if the file does not exists within the jar file.
* @throws IOException if there was a problem reading from the jar file.
*/
public static Optional loadFileContentFrom(URL jarFile) throws IOException {
/* A specific implementation of JarURLConnection is required to read jar content because not all implementations
* support ways to disable connection caching. Disabling connection caching is necessary to avoid file descriptor leaks.
* See MULE-13139.
*/
JarURLConnection jarConnection =
new sun.net.www.protocol.jar.JarURLConnection(jarFile, new sun.net.www.protocol.jar.Handler());
jarConnection.setUseCaches(false);
try (InputStream stream = jarConnection.getInputStream()) {
return of(toByteArray(stream));
} catch (FileNotFoundException e) {
return empty();
}
}
/**
* Creates an URL to a path within a jar file.
*
* @param jarFile the jar file
* @param filePath the path within the jar file
* @return an URL to the {@code filePath} within the {@code jarFile}
* @throws MalformedURLException if the provided {@code filePath} is malformed
*/
public static URL getUrlWithinJar(File jarFile, String filePath) throws MalformedURLException {
return new URL("jar:" + jarFile.toURI().toString() + "!/" + filePath);
}
/**
* Gets all the URL of files within a directory
*
* @param file the jar file
* @param directory the directory within the jar file
* @return a collection of URLs to files within the directory {@code directory}. Empty collection if the directory does not
* exists or is empty.
* @throws IOException
*/
public static List getUrlsWithinJar(File file, String directory) throws IOException {
List urls = new ArrayList<>();
try (JarFile jarFile = new JarFile(file)) {
Enumeration entries = jarFile.entries();
while (entries.hasMoreElements()) {
JarEntry jarEntry = entries.nextElement();
if (!jarEntry.isDirectory() && jarEntry.getName().startsWith(directory + "/")) {
urls.add(getUrlWithinJar(file, jarEntry.getName()));
}
}
}
return urls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy