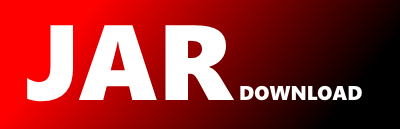
org.mustangproject.ZUGFeRD.DAPullProvider Maven / Gradle / Ivy
Show all versions of library Show documentation
/**
* *********************************************************************
*
* Copyright 2018 Jochen Staerk
*
* Use is subject to license terms.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at http://www.apache.org/licenses/LICENSE-2.0.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
*
* See the License for the specific language governing permissions and
* limitations under the License.
*
* **********************************************************************
*/
package org.mustangproject.ZUGFeRD;
import static org.mustangproject.ZUGFeRD.ZUGFeRDDateFormat.DATE;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import java.util.Optional;
import org.mustangproject.EStandard;
import org.mustangproject.FileAttachment;
import org.mustangproject.Invoice;
import org.mustangproject.XMLTools;
public class DAPullProvider extends ZUGFeRD2PullProvider {
protected IExportableTransaction trans;
protected Profile profile = Profiles.getByName(EStandard.despatchadvice,"pilot", 1);
@Override
public void generateXML(IExportableTransaction trans) {
this.trans = trans;
final String typecode = "220";
/*if (trans.getDocumentCode() != null) {
typecode = trans.getDocumentCode();
}*/
String testBooleanStr="true";
String xml = "\n"
// + "
// xsi:schemaLocation=\"urn:un:unece:uncefact:data:standard:CrossIndustryInvoice:100
// ../Schema/ZUGFeRD1p0.xsd\""
+ ""
// + "
+" "+testBooleanStr+" \n"
//
+ ""
+ "" + getProfile().getID() + " "
+ " "
+ " "
+ ""
+ "" + XMLTools.encodeXML(trans.getNumber()) + " "
// + " RECHNUNG "
// + "380 "
+ "" + typecode + " "
+ ""
+ DATE.udtFormat(trans.getIssueDate()) + " " // date
+ buildNotes(trans)
+ " "
+ "";
int lineID = 0;
for (final IZUGFeRDExportableItem currentItem : trans.getZFItems()) {
lineID++;
if (currentItem.getProduct().getTaxExemptionReason() != null) {
// exemptionReason = "" + XMLTools.encodeXML(currentItem.getProduct().getTaxExemptionReason()) + " ";
}
final LineCalculator lc = new LineCalculator(currentItem);
xml += "" +
""
+ "" + lineID + " "
+ buildItemNotes(currentItem)
+ " "
+ "";
// + " 4012345001235 "
if (currentItem.getProduct().getSellerAssignedID() != null) {
xml += ""
+ XMLTools.encodeXML(currentItem.getProduct().getSellerAssignedID()) + " ";
}
if (currentItem.getProduct().getBuyerAssignedID() != null) {
xml += ""
+ XMLTools.encodeXML(currentItem.getProduct().getBuyerAssignedID()) + " ";
}
String allowanceChargeStr = "";
if (currentItem.getItemAllowances() != null && currentItem.getItemAllowances().length > 0) {
for (final IZUGFeRDAllowanceCharge allowance : currentItem.getItemAllowances()) {
allowanceChargeStr += getAllowanceChargeStr(allowance, currentItem);
}
}
if (currentItem.getItemCharges() != null && currentItem.getItemCharges().length > 0) {
for (final IZUGFeRDAllowanceCharge charge : currentItem.getItemCharges()) {
allowanceChargeStr += getAllowanceChargeStr(charge, currentItem);
}
}
xml += "" + XMLTools.encodeXML(currentItem.getProduct().getName()) + " "
+ "" + XMLTools.encodeXML(currentItem.getProduct().getDescription())
+ " "
+ " "
+ ""
+ ""
+ quantityFormat(currentItem.getQuantity()) + " "
+ " "
+ "";
if ((currentItem.getDetailedDeliveryPeriodFrom() != null) || (currentItem.getDetailedDeliveryPeriodTo() != null)) {
xml += "";
if (currentItem.getDetailedDeliveryPeriodFrom() != null) {
xml += "" + DATE.udtFormat(currentItem.getDetailedDeliveryPeriodFrom()) + " ";
}
if (currentItem.getDetailedDeliveryPeriodTo() != null) {
xml += "" + DATE.udtFormat(currentItem.getDetailedDeliveryPeriodTo()) + " ";
}
xml += " ";
}
xml += ""
+ "" + currencyFormat(lc.getItemTotalNetAmount())
+ " " // currencyID=\"EUR\"
+ " ";
/* if (currentItem.getAdditionalReferencedDocumentID() != null) {
xml += "" + currentItem.getAdditionalReferencedDocumentID() + " 130 ";
}*/
xml += " "
+ " ";
}
xml += "";
if (trans.getReferenceNumber() != null) {
xml += "" + XMLTools.encodeXML(trans.getReferenceNumber()) + " ";
}
xml += ""
+ getTradePartyAsXML(trans.getSender(), true, false)
+ " "
+ "";
// + " GE2020211 "
// + " 4000001987658 "
xml += getTradePartyAsXML(trans.getRecipient(), false, false);
xml += " ";
if (trans.getSellerOrderReferencedDocumentID() != null) {
xml += ""
+ ""
+ XMLTools.encodeXML(trans.getSellerOrderReferencedDocumentID()) + " "
+ " ";
}
if (trans.getBuyerOrderReferencedDocumentID() != null) {
xml += ""
+ ""
+ XMLTools.encodeXML(trans.getBuyerOrderReferencedDocumentID()) + " "
+ " ";
}
if (trans.getContractReferencedDocument() != null) {
xml += ""
+ ""
+ XMLTools.encodeXML(trans.getContractReferencedDocument()) + " "
+ " ";
}
// Additional Documents of XRechnung (Rechnungsbegruendende Unterlagen - BG-24 XRechnung)
if (trans.getAdditionalReferencedDocuments() != null) {
for (final FileAttachment f : trans.getAdditionalReferencedDocuments()) {
final String documentContent = new String(Base64.getEncoder().encodeToString(f.getData()));
xml += ""
+ "" + f.getFilename() + " "
+ "916 "
+ "" + f.getDescription() + " "
+ "" + documentContent + " "
+ " ";
}
}
if (trans.getSpecifiedProcuringProjectID() != null) {
xml += ""
+ ""
+ XMLTools.encodeXML(trans.getSpecifiedProcuringProjectID()) + " ";
if (trans.getSpecifiedProcuringProjectName() != null) {
xml += "" + XMLTools.encodeXML(trans.getSpecifiedProcuringProjectName()) + " ";
}
xml += " ";
}
xml += " "
+ "";
if (this.trans.getDeliveryAddress() != null) {
xml += "" +
getTradePartyAsXML(this.trans.getDeliveryAddress(), false, true) +
" ";
}
xml += " \n" +
" \n" +
" "+ DATE.udtFormat(trans.getDeliveryDate() )+" \n" +
" \n" +
" ";
/*
xml += ""
+ "";
if (trans.getDeliveryDate() != null) {
xml += DATE.udtFormat(trans.getDeliveryDate());
} else {
throw new IllegalStateException("No delivery date provided");
}
xml += " \n";
xml += " \n"
*/
/*
* + "\n" +
* "20130603 \n" +
* "2013-51112 \n" +
* " \n"
*/
xml+= " \n";
// + " "
// + " "
// + " "
// + " Wir erlauben uns Ihnen folgende Positionen aus der Lieferung Nr.
// 2013-51112 in Rechnung zu stellen: \n"
// + " \n"
// + " \n"
// + " \n";
xml += " "
+ " ";
final byte[] zugferdRaw;
zugferdRaw = xml.getBytes(StandardCharsets.UTF_8);
zugferdData = XMLTools.removeBOM(zugferdRaw);
}
@Override
public void setProfile(Profile p) {
profile = p;
}
@Override
public Profile getProfile() {
return profile;
}
@Override
protected String buildNotes(IExportableTransaction exportableTransaction) {
Invoice copyWithoutRebateInfo = new Invoice()
.setOwnOrganisationFullPlaintextInfo(exportableTransaction.getOwnOrganisationFullPlaintextInfo())
.addNotes(exportableTransaction.getNotesWithSubjectCode());
if(exportableTransaction.getNotes() != null) {
for (String note : exportableTransaction.getNotes()) {
copyWithoutRebateInfo.addNote(note);
}
}
Optional.ofNullable(exportableTransaction.getSubjectNote()).ifPresent(copyWithoutRebateInfo::addNote);
return super.buildNotes(copyWithoutRebateInfo);
}
}