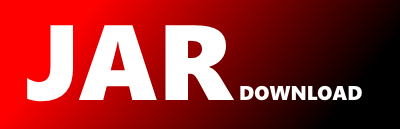
package.index.d.ts Maven / Gradle / Ivy
/**
* @license Angular v18.2.1
* (c) 2010-2024 Google LLC. https://angular.io/
* License: MIT
*/
import { ApplicationConfig as ApplicationConfig_2 } from '@angular/core';
import { ApplicationRef } from '@angular/core';
import { ComponentRef } from '@angular/core';
import { DebugElement } from '@angular/core';
import { DebugNode } from '@angular/core';
import { EnvironmentProviders } from '@angular/core';
import { GetTestability } from '@angular/core';
import { HttpTransferCacheOptions } from '@angular/common/http';
import * as i0 from '@angular/core';
import * as i1 from '@angular/common';
import { InjectionToken } from '@angular/core';
import { ModuleWithProviders } from '@angular/core';
import { NgZone } from '@angular/core';
import { OnDestroy } from '@angular/core';
import { PlatformRef } from '@angular/core';
import { Predicate } from '@angular/core';
import { Provider } from '@angular/core';
import { Renderer2 } from '@angular/core';
import { RendererFactory2 } from '@angular/core';
import { RendererType2 } from '@angular/core';
import { Sanitizer } from '@angular/core';
import { SecurityContext } from '@angular/core';
import { StaticProvider } from '@angular/core';
import { Testability } from '@angular/core';
import { TestabilityRegistry } from '@angular/core';
import { Type } from '@angular/core';
import { Version } from '@angular/core';
import { ɵConsole } from '@angular/core';
import { ɵDomAdapter } from '@angular/common';
import { ɵgetDOM } from '@angular/common';
/**
* Set of config options available during the application bootstrap operation.
*
* @publicApi
*
* @deprecated
* `ApplicationConfig` has moved, please import `ApplicationConfig` from `@angular/core` instead.
*/
export declare type ApplicationConfig = ApplicationConfig_2;
/**
* Bootstraps an instance of an Angular application and renders a standalone component as the
* application's root component. More information about standalone components can be found in [this
* guide](guide/components/importing).
*
* @usageNotes
* The root component passed into this function *must* be a standalone one (should have the
* `standalone: true` flag in the `@Component` decorator config).
*
* ```typescript
* @Component({
* standalone: true,
* template: 'Hello world!'
* })
* class RootComponent {}
*
* const appRef: ApplicationRef = await bootstrapApplication(RootComponent);
* ```
*
* You can add the list of providers that should be available in the application injector by
* specifying the `providers` field in an object passed as the second argument:
*
* ```typescript
* await bootstrapApplication(RootComponent, {
* providers: [
* {provide: BACKEND_URL, useValue: 'https://yourdomain.com/api'}
* ]
* });
* ```
*
* The `importProvidersFrom` helper method can be used to collect all providers from any
* existing NgModule (and transitively from all NgModules that it imports):
*
* ```typescript
* await bootstrapApplication(RootComponent, {
* providers: [
* importProvidersFrom(SomeNgModule)
* ]
* });
* ```
*
* Note: the `bootstrapApplication` method doesn't include [Testability](api/core/Testability) by
* default. You can add [Testability](api/core/Testability) by getting the list of necessary
* providers using `provideProtractorTestingSupport()` function and adding them into the `providers`
* array, for example:
*
* ```typescript
* import {provideProtractorTestingSupport} from '@angular/platform-browser';
*
* await bootstrapApplication(RootComponent, {providers: [provideProtractorTestingSupport()]});
* ```
*
* @param rootComponent A reference to a standalone component that should be rendered.
* @param options Extra configuration for the bootstrap operation, see `ApplicationConfig` for
* additional info.
* @returns A promise that returns an `ApplicationRef` instance once resolved.
*
* @publicApi
*/
export declare function bootstrapApplication(rootComponent: Type, options?: ApplicationConfig): Promise;
/**
* Exports required infrastructure for all Angular apps.
* Included by default in all Angular apps created with the CLI
* `new` command.
* Re-exports `CommonModule` and `ApplicationModule`, making their
* exports and providers available to all apps.
*
* @publicApi
*/
export declare class BrowserModule {
constructor(providersAlreadyPresent: boolean | null);
/**
* Configures a browser-based app to transition from a server-rendered app, if
* one is present on the page.
*
* @param params An object containing an identifier for the app to transition.
* The ID must match between the client and server versions of the app.
* @returns The reconfigured `BrowserModule` to import into the app's root `AppModule`.
*
* @deprecated Use {@link APP_ID} instead to set the application ID.
*/
static withServerTransition(params: {
appId: string;
}): ModuleWithProviders;
static ɵfac: i0.ɵɵFactoryDeclaration;
static ɵmod: i0.ɵɵNgModuleDeclaration;
static ɵinj: i0.ɵɵInjectorDeclaration;
}
/**
* Predicates for use with {@link DebugElement}'s query functions.
*
* @publicApi
*/
export declare class By {
/**
* Match all nodes.
*
* @usageNotes
* ### Example
*
* {@example platform-browser/dom/debug/ts/by/by.ts region='by_all'}
*/
static all(): Predicate;
/**
* Match elements by the given CSS selector.
*
* @usageNotes
* ### Example
*
* {@example platform-browser/dom/debug/ts/by/by.ts region='by_css'}
*/
static css(selector: string): Predicate;
/**
* Match nodes that have the given directive present.
*
* @usageNotes
* ### Example
*
* {@example platform-browser/dom/debug/ts/by/by.ts region='by_directive'}
*/
static directive(type: Type): Predicate;
}
/**
* Create an instance of an Angular application without bootstrapping any components. This is useful
* for the situation where one wants to decouple application environment creation (a platform and
* associated injectors) from rendering components on a screen. Components can be subsequently
* bootstrapped on the returned `ApplicationRef`.
*
* @param options Extra configuration for the application environment, see `ApplicationConfig` for
* additional info.
* @returns A promise that returns an `ApplicationRef` instance once resolved.
*
* @publicApi
*/
export declare function createApplication(options?: ApplicationConfig): Promise;
/**
* Disables Angular tools.
*
* @publicApi
*/
export declare function disableDebugTools(): void;
/**
* DomSanitizer helps preventing Cross Site Scripting Security bugs (XSS) by sanitizing
* values to be safe to use in the different DOM contexts.
*
* For example, when binding a URL in an `` hyperlink, `someValue` will be
* sanitized so that an attacker cannot inject e.g. a `javascript:` URL that would execute code on
* the website.
*
* In specific situations, it might be necessary to disable sanitization, for example if the
* application genuinely needs to produce a `javascript:` style link with a dynamic value in it.
* Users can bypass security by constructing a value with one of the `bypassSecurityTrust...`
* methods, and then binding to that value from the template.
*
* These situations should be very rare, and extraordinary care must be taken to avoid creating a
* Cross Site Scripting (XSS) security bug!
*
* When using `bypassSecurityTrust...`, make sure to call the method as early as possible and as
* close as possible to the source of the value, to make it easy to verify no security bug is
* created by its use.
*
* It is not required (and not recommended) to bypass security if the value is safe, e.g. a URL that
* does not start with a suspicious protocol, or an HTML snippet that does not contain dangerous
* code. The sanitizer leaves safe values intact.
*
* @security Calling any of the `bypassSecurityTrust...` APIs disables Angular's built-in
* sanitization for the value passed in. Carefully check and audit all values and code paths going
* into this call. Make sure any user data is appropriately escaped for this security context.
* For more detail, see the [Security Guide](https://g.co/ng/security).
*
* @publicApi
*/
export declare abstract class DomSanitizer implements Sanitizer {
/**
* Gets a safe value from either a known safe value or a value with unknown safety.
*
* If the given value is already a `SafeValue`, this method returns the unwrapped value.
* If the security context is HTML and the given value is a plain string, this method
* sanitizes the string, removing any potentially unsafe content.
* For any other security context, this method throws an error if provided
* with a plain string.
*/
abstract sanitize(context: SecurityContext, value: SafeValue | string | null): string | null;
/**
* Bypass security and trust the given value to be safe HTML. Only use this when the bound HTML
* is unsafe (e.g. contains `