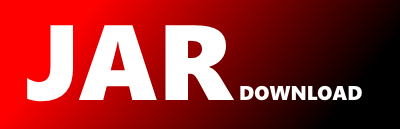
package.dist.components.checkbox.use-checkbox-group.cjs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
A collection of unstyled, accessible UI components for React, utilizing state machines for seamless interaction.
The newest version!
'use client';
'use strict';
Object.defineProperty(exports, Symbol.toStringTag, { value: 'Module' });
const useControllableState = require('../../utils/use-controllable-state.cjs');
const useEvent = require('../../utils/use-event.cjs');
function useCheckboxGroup(props = {}) {
const {
defaultValue,
value: controlledValue,
onValueChange,
disabled,
readOnly,
name,
invalid
} = props;
const interative = !(disabled || readOnly);
const onChangeProp = useEvent.useEvent(onValueChange, { sync: true });
const [value, setValue] = useControllableState.useControllableState({
value: controlledValue,
defaultValue: defaultValue || [],
onChange: onChangeProp
});
const isChecked = (val) => {
return value.some((v) => String(v) === String(val));
};
const toggleValue = (val) => {
isChecked(val) ? removeValue(val) : addValue(val);
};
const addValue = (val) => {
if (!interative) return;
if (isChecked(val)) return;
setValue(value.concat(val));
};
const removeValue = (val) => {
if (!interative) return;
setValue(value.filter((v) => String(v) !== String(val)));
};
const getItemProps = (props2) => {
return {
checked: props2.value != null ? isChecked(props2.value) : void 0,
onCheckedChange() {
if (props2.value != null) {
toggleValue(props2.value);
}
},
name,
disabled,
readOnly,
invalid
};
};
return {
isChecked,
value,
name,
disabled: !!disabled,
readOnly: !!readOnly,
invalid: !!invalid,
setValue,
addValue,
toggleValue,
getItemProps
};
}
exports.useCheckboxGroup = useCheckboxGroup;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy