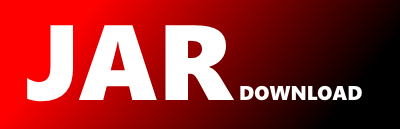
package.dist.components.fieldset.use-fieldset.cjs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
A collection of unstyled, accessible UI components for React, utilizing state machines for seamless interaction.
The newest version!
'use client';
'use strict';
Object.defineProperty(exports, Symbol.toStringTag, { value: 'Module' });
const domQuery = require('@zag-js/dom-query');
const react = require('react');
const fieldset_anatomy = require('./fieldset.anatomy.cjs');
const useSafeLayoutEffect = require('../../utils/use-safe-layout-effect.cjs');
const useFieldset = (props) => {
const { disabled = false, invalid = false } = props;
const hasErrorText = react.useRef(false);
const hasHelperText = react.useRef(false);
const id = props.id ?? react.useId();
const rootRef = react.useRef(null);
const errorTextId = `fieldset::${id}::error-text`;
const helperTextId = `fieldset::${id}::helper-text`;
useSafeLayoutEffect.useSafeLayoutEffect(() => {
const rootNode = rootRef.current;
if (!rootNode) return;
const win = domQuery.getWindow(rootNode);
const doc = win.document;
const checkTextElements = () => {
hasErrorText.current = !!doc.getElementById(errorTextId);
hasHelperText.current = !!doc.getElementById(helperTextId);
};
checkTextElements();
const observer = new win.MutationObserver(checkTextElements);
observer.observe(rootNode, { childList: true, subtree: true });
return () => observer.disconnect();
}, [errorTextId, helperTextId]);
const labelIds = react.useMemo(() => {
const ids = [];
if (hasErrorText.current && invalid) ids.push(errorTextId);
if (hasHelperText.current) ids.push(helperTextId);
return ids.join(" ") || void 0;
}, [invalid, errorTextId, helperTextId]);
const getRootProps = react.useMemo(
() => () => ({
...fieldset_anatomy.parts.root.attrs,
ref: rootRef,
disabled,
"data-disabled": disabled ? "true" : void 0,
"data-invalid": invalid ? "true" : void 0,
"aria-describedby": labelIds
}),
[disabled, invalid, labelIds]
);
const getLegendProps = react.useMemo(
() => () => ({
...fieldset_anatomy.parts.legend.attrs,
"data-disabled": disabled ? "true" : void 0,
"data-invalid": invalid ? "true" : void 0
}),
[disabled, invalid]
);
const getHelperTextProps = react.useMemo(
() => () => ({
id: helperTextId,
...fieldset_anatomy.parts.helperText.attrs
}),
[helperTextId]
);
const getErrorTextProps = react.useMemo(
() => () => ({
id: errorTextId,
...fieldset_anatomy.parts.errorText.attrs,
"aria-live": "polite"
}),
[errorTextId]
);
return {
refs: {
rootRef
},
disabled,
invalid,
getRootProps,
getLegendProps,
getHelperTextProps,
getErrorTextProps
};
};
exports.useFieldset = useFieldset;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy