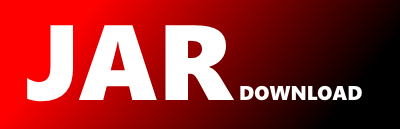
package.lib.index.js.map Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helpers Show documentation
Show all versions of helpers Show documentation
Collection of helper functions used by Babel transforms.
{"version":3,"names":["_traverse","require","_t","_helpers","assignmentExpression","cloneNode","expressionStatement","file","identifier","makePath","path","parts","parentPath","push","key","inList","listKey","reverse","join","FileClass","undefined","getHelperMetadata","globals","Set","localBindingNames","dependencies","Map","exportName","exportPath","exportBindingAssignments","importPaths","importBindingsReferences","dependencyVisitor","ImportDeclaration","child","name","node","source","value","helpers","buildCodeFrameError","get","length","isImportDefaultSpecifier","bindingIdentifier","specifiers","local","set","ExportDefaultDeclaration","decl","isFunctionDeclaration","id","ExportAllDeclaration","ExportNamedDeclaration","Statement","isImportDeclaration","isExportDeclaration","skip","referenceVisitor","Program","bindings","scope","getAllBindings","Object","keys","forEach","has","add","ReferencedIdentifier","binding","getBinding","AssignmentExpression","left","getBindingIdentifiers","isIdentifier","isProgram","traverse","ast","Error","Array","from","permuteHelperAST","metadata","localBindings","getDependency","dependenciesRefs","toRename","type","newName","exp","imps","map","p","impsBindingRefs","replaceWith","assignPath","assign","pushContainer","rename","remove","helperData","create","loadHelper","helper","ReferenceError","code","fn","fakeFile","stop","filename","inputMap","minVersion","build","nodes","program","body","getDependencies","values","ensure","newFileClass","list","exports","replace","_default","default"],"sources":["../src/index.ts"],"sourcesContent":["import type { File } from \"@babel/core\";\nimport type { NodePath, Visitor } from \"@babel/traverse\";\nimport traverse from \"@babel/traverse\";\nimport {\n assignmentExpression,\n cloneNode,\n expressionStatement,\n file,\n identifier,\n} from \"@babel/types\";\nimport type * as t from \"@babel/types\";\nimport helpers from \"./helpers.ts\";\n\nfunction makePath(path: NodePath) {\n const parts = [];\n\n for (; path.parentPath; path = path.parentPath) {\n parts.push(path.key);\n if (path.inList) parts.push(path.listKey);\n }\n\n return parts.reverse().join(\".\");\n}\n\nlet FileClass: typeof File | undefined = undefined;\n\ninterface HelperMetadata {\n globals: string[];\n localBindingNames: string[];\n dependencies: Map;\n exportBindingAssignments: string[];\n exportPath: string;\n exportName: string;\n importBindingsReferences: string[];\n importPaths: string[];\n}\n\n/**\n * Given a file AST for a given helper, get a bunch of metadata about it so that Babel can quickly render\n * the helper is whatever context it is needed in.\n */\nfunction getHelperMetadata(file: File): HelperMetadata {\n const globals = new Set();\n const localBindingNames = new Set();\n // Maps imported identifier -> helper name\n const dependencies = new Map();\n\n let exportName: string | undefined;\n let exportPath: string | undefined;\n const exportBindingAssignments: string[] = [];\n const importPaths: string[] = [];\n const importBindingsReferences: string[] = [];\n\n const dependencyVisitor: Visitor = {\n ImportDeclaration(child) {\n const name = child.node.source.value;\n if (!helpers[name]) {\n throw child.buildCodeFrameError(`Unknown helper ${name}`);\n }\n if (\n child.get(\"specifiers\").length !== 1 ||\n // @ts-expect-error isImportDefaultSpecifier does not work with NodePath union\n !child.get(\"specifiers.0\").isImportDefaultSpecifier()\n ) {\n throw child.buildCodeFrameError(\n \"Helpers can only import a default value\",\n );\n }\n const bindingIdentifier = child.node.specifiers[0].local;\n dependencies.set(bindingIdentifier, name);\n importPaths.push(makePath(child));\n },\n ExportDefaultDeclaration(child) {\n const decl = child.get(\"declaration\");\n\n if (!decl.isFunctionDeclaration() || !decl.node.id) {\n throw decl.buildCodeFrameError(\n \"Helpers can only export named function declarations\",\n );\n }\n\n exportName = decl.node.id.name;\n exportPath = makePath(child);\n },\n ExportAllDeclaration(child) {\n throw child.buildCodeFrameError(\"Helpers can only export default\");\n },\n ExportNamedDeclaration(child) {\n throw child.buildCodeFrameError(\"Helpers can only export default\");\n },\n Statement(child) {\n if (child.isImportDeclaration() || child.isExportDeclaration()) return;\n\n child.skip();\n },\n };\n\n const referenceVisitor: Visitor = {\n Program(path) {\n const bindings = path.scope.getAllBindings();\n\n Object.keys(bindings).forEach(name => {\n if (name === exportName) return;\n if (dependencies.has(bindings[name].identifier)) return;\n\n localBindingNames.add(name);\n });\n },\n ReferencedIdentifier(child) {\n const name = child.node.name;\n const binding = child.scope.getBinding(name);\n if (!binding) {\n globals.add(name);\n } else if (dependencies.has(binding.identifier)) {\n importBindingsReferences.push(makePath(child));\n }\n },\n AssignmentExpression(child) {\n const left = child.get(\"left\");\n\n if (!(exportName in left.getBindingIdentifiers())) return;\n\n if (!left.isIdentifier()) {\n throw left.buildCodeFrameError(\n \"Only simple assignments to exports are allowed in helpers\",\n );\n }\n\n const binding = child.scope.getBinding(exportName);\n\n if (binding?.scope.path.isProgram()) {\n exportBindingAssignments.push(makePath(child));\n }\n },\n };\n\n traverse(file.ast, dependencyVisitor, file.scope);\n traverse(file.ast, referenceVisitor, file.scope);\n\n if (!exportPath) throw new Error(\"Helpers must have a default export.\");\n\n // Process these in reverse so that mutating the references does not invalidate any later paths in\n // the list.\n exportBindingAssignments.reverse();\n\n return {\n globals: Array.from(globals),\n localBindingNames: Array.from(localBindingNames),\n dependencies,\n exportBindingAssignments,\n exportPath,\n exportName,\n importBindingsReferences,\n importPaths,\n };\n}\n\ntype GetDependency = (name: string) => t.Expression;\n\n/**\n * Given a helper AST and information about how it will be used, update the AST to match the usage.\n */\nfunction permuteHelperAST(\n file: File,\n metadata: HelperMetadata,\n id?: t.Identifier | t.MemberExpression,\n localBindings?: string[],\n getDependency?: GetDependency,\n) {\n if (localBindings && !id) {\n throw new Error(\"Unexpected local bindings for module-based helpers.\");\n }\n\n if (!id) return;\n\n const {\n localBindingNames,\n dependencies,\n exportBindingAssignments,\n exportPath,\n exportName,\n importBindingsReferences,\n importPaths,\n } = metadata;\n\n const dependenciesRefs: Record = {};\n dependencies.forEach((name, id) => {\n dependenciesRefs[id.name] =\n (typeof getDependency === \"function\" && getDependency(name)) || id;\n });\n\n const toRename: Record = {};\n const bindings = new Set(localBindings || []);\n if (id.type === \"Identifier\") bindings.add(id.name);\n localBindingNames.forEach(name => {\n let newName = name;\n while (bindings.has(newName)) newName = \"_\" + newName;\n\n if (newName !== name) toRename[name] = newName;\n });\n\n if (id.type === \"Identifier\" && exportName !== id.name) {\n toRename[exportName] = id.name;\n }\n\n const { path } = file;\n\n // We need to compute these in advance because removing nodes would\n // invalidate the paths.\n const exp: NodePath = path.get(exportPath);\n const imps: NodePath[] = importPaths.map(p =>\n path.get(p),\n );\n const impsBindingRefs: NodePath[] =\n importBindingsReferences.map(p => path.get(p));\n\n // We assert that this is a FunctionDeclaration in dependencyVisitor.\n const decl = exp.get(\"declaration\") as NodePath;\n\n if (id.type === \"Identifier\") {\n exp.replaceWith(decl);\n } else if (id.type === \"MemberExpression\") {\n exportBindingAssignments.forEach(assignPath => {\n const assign: NodePath = path.get(assignPath);\n assign.replaceWith(assignmentExpression(\"=\", id, assign.node));\n });\n exp.replaceWith(decl);\n path.pushContainer(\n \"body\",\n expressionStatement(\n assignmentExpression(\"=\", id, identifier(exportName)),\n ),\n );\n } else {\n throw new Error(\"Unexpected helper format.\");\n }\n\n Object.keys(toRename).forEach(name => {\n path.scope.rename(name, toRename[name]);\n });\n\n for (const path of imps) path.remove();\n for (const path of impsBindingRefs) {\n const node = cloneNode(dependenciesRefs[path.node.name]);\n path.replaceWith(node);\n }\n}\n\ninterface HelperData {\n build: (\n getDependency: GetDependency,\n id: t.Identifier | t.MemberExpression,\n localBindings: string[],\n ) => {\n nodes: t.Program[\"body\"];\n globals: string[];\n };\n minVersion: string;\n getDependencies: () => string[];\n}\n\nconst helperData: Record = Object.create(null);\nfunction loadHelper(name: string) {\n if (!helperData[name]) {\n const helper = helpers[name];\n if (!helper) {\n throw Object.assign(new ReferenceError(`Unknown helper ${name}`), {\n code: \"BABEL_HELPER_UNKNOWN\",\n helper: name,\n });\n }\n\n const fn = (): File => {\n if (!process.env.BABEL_8_BREAKING) {\n if (!FileClass) {\n const fakeFile = { ast: file(helper.ast()), path: null } as File;\n traverse(fakeFile.ast, {\n Program: path => (fakeFile.path = path).stop(),\n });\n return fakeFile;\n }\n }\n return new FileClass(\n { filename: `babel-helper://${name}` },\n {\n ast: file(helper.ast()),\n code: \"[internal Babel helper code]\",\n inputMap: null,\n },\n );\n };\n\n // We compute the helper metadata lazily, so that we skip that\n // work if we only need the `.minVersion` (for example because\n // of a call to `.availableHelper` when `@babel/runtime`).\n let metadata: HelperMetadata | null = null;\n\n helperData[name] = {\n minVersion: helper.minVersion,\n build(getDependency, id, localBindings) {\n const file = fn();\n metadata ||= getHelperMetadata(file);\n permuteHelperAST(file, metadata, id, localBindings, getDependency);\n\n return {\n nodes: file.ast.program.body,\n globals: metadata.globals,\n };\n },\n getDependencies() {\n metadata ||= getHelperMetadata(fn());\n return Array.from(metadata.dependencies.values());\n },\n };\n }\n\n return helperData[name];\n}\n\nexport function get(\n name: string,\n getDependency?: GetDependency,\n id?: t.Identifier | t.MemberExpression,\n localBindings?: string[],\n) {\n return loadHelper(name).build(getDependency, id, localBindings);\n}\n\nexport function minVersion(name: string) {\n return loadHelper(name).minVersion;\n}\n\nexport function getDependencies(name: string): ReadonlyArray {\n return loadHelper(name).getDependencies();\n}\n\nexport function ensure(name: string, newFileClass: typeof File) {\n // We inject the File class here rather than importing it to avoid\n // circular dependencies between @babel/core and @babel/helpers.\n FileClass ||= newFileClass;\n\n loadHelper(name);\n}\n\nexport const list = Object.keys(helpers).map(name => name.replace(/^_/, \"\"));\n\nexport default get;\n"],"mappings":";;;;;;;;;;;AAEA,IAAAA,SAAA,GAAAC,OAAA;AACA,IAAAC,EAAA,GAAAD,OAAA;AAQA,IAAAE,QAAA,GAAAF,OAAA;AAAmC;EAPjCG,oBAAoB;EACpBC,SAAS;EACTC,mBAAmB;EACnBC,IAAI;EACJC;AAAU,IAAAN,EAAA;AAKZ,SAASO,QAAQA,CAACC,IAAc,EAAE;EAChC,MAAMC,KAAK,GAAG,EAAE;EAEhB,OAAOD,IAAI,CAACE,UAAU,EAAEF,IAAI,GAAGA,IAAI,CAACE,UAAU,EAAE;IAC9CD,KAAK,CAACE,IAAI,CAACH,IAAI,CAACI,GAAG,CAAC;IACpB,IAAIJ,IAAI,CAACK,MAAM,EAAEJ,KAAK,CAACE,IAAI,CAACH,IAAI,CAACM,OAAO,CAAC;EAC3C;EAEA,OAAOL,KAAK,CAACM,OAAO,CAAC,CAAC,CAACC,IAAI,CAAC,GAAG,CAAC;AAClC;AAEA,IAAIC,SAAkC,GAAGC,SAAS;AAiBlD,SAASC,iBAAiBA,CAACd,IAAU,EAAkB;EACrD,MAAMe,OAAO,GAAG,IAAIC,GAAG,CAAS,CAAC;EACjC,MAAMC,iBAAiB,GAAG,IAAID,GAAG,CAAS,CAAC;EAE3C,MAAME,YAAY,GAAG,IAAIC,GAAG,CAAuB,CAAC;EAEpD,IAAIC,UAA8B;EAClC,IAAIC,UAA8B;EAClC,MAAMC,wBAAkC,GAAG,EAAE;EAC7C,MAAMC,WAAqB,GAAG,EAAE;EAChC,MAAMC,wBAAkC,GAAG,EAAE;EAE7C,MAAMC,iBAA0B,GAAG;IACjCC,iBAAiBA,CAACC,KAAK,EAAE;MACvB,MAAMC,IAAI,GAAGD,KAAK,CAACE,IAAI,CAACC,MAAM,CAACC,KAAK;MACpC,IAAI,CAACC,gBAAO,CAACJ,IAAI,CAAC,EAAE;QAClB,MAAMD,KAAK,CAACM,mBAAmB,CAAE,kBAAiBL,IAAK,EAAC,CAAC;MAC3D;MACA,IACED,KAAK,CAACO,GAAG,CAAC,YAAY,CAAC,CAACC,MAAM,KAAK,CAAC,IAEpC,CAACR,KAAK,CAACO,GAAG,CAAC,cAAc,CAAC,CAACE,wBAAwB,CAAC,CAAC,EACrD;QACA,MAAMT,KAAK,CAACM,mBAAmB,CAC7B,yCACF,CAAC;MACH;MACA,MAAMI,iBAAiB,GAAGV,KAAK,CAACE,IAAI,CAACS,UAAU,CAAC,CAAC,CAAC,CAACC,KAAK;MACxDrB,YAAY,CAACsB,GAAG,CAACH,iBAAiB,EAAET,IAAI,CAAC;MACzCL,WAAW,CAACjB,IAAI,CAACJ,QAAQ,CAACyB,KAAK,CAAC,CAAC;IACnC,CAAC;IACDc,wBAAwBA,CAACd,KAAK,EAAE;MAC9B,MAAMe,IAAI,GAAGf,KAAK,CAACO,GAAG,CAAC,aAAa,CAAC;MAErC,IAAI,CAACQ,IAAI,CAACC,qBAAqB,CAAC,CAAC,IAAI,CAACD,IAAI,CAACb,IAAI,CAACe,EAAE,EAAE;QAClD,MAAMF,IAAI,CAACT,mBAAmB,CAC5B,qDACF,CAAC;MACH;MAEAb,UAAU,GAAGsB,IAAI,CAACb,IAAI,CAACe,EAAE,CAAChB,IAAI;MAC9BP,UAAU,GAAGnB,QAAQ,CAACyB,KAAK,CAAC;IAC9B,CAAC;IACDkB,oBAAoBA,CAAClB,KAAK,EAAE;MAC1B,MAAMA,KAAK,CAACM,mBAAmB,CAAC,iCAAiC,CAAC;IACpE,CAAC;IACDa,sBAAsBA,CAACnB,KAAK,EAAE;MAC5B,MAAMA,KAAK,CAACM,mBAAmB,CAAC,iCAAiC,CAAC;IACpE,CAAC;IACDc,SAASA,CAACpB,KAAK,EAAE;MACf,IAAIA,KAAK,CAACqB,mBAAmB,CAAC,CAAC,IAAIrB,KAAK,CAACsB,mBAAmB,CAAC,CAAC,EAAE;MAEhEtB,KAAK,CAACuB,IAAI,CAAC,CAAC;IACd;EACF,CAAC;EAED,MAAMC,gBAAyB,GAAG;IAChCC,OAAOA,CAACjD,IAAI,EAAE;MACZ,MAAMkD,QAAQ,GAAGlD,IAAI,CAACmD,KAAK,CAACC,cAAc,CAAC,CAAC;MAE5CC,MAAM,CAACC,IAAI,CAACJ,QAAQ,CAAC,CAACK,OAAO,CAAC9B,IAAI,IAAI;QACpC,IAAIA,IAAI,KAAKR,UAAU,EAAE;QACzB,IAAIF,YAAY,CAACyC,GAAG,CAACN,QAAQ,CAACzB,IAAI,CAAC,CAAC3B,UAAU,CAAC,EAAE;QAEjDgB,iBAAiB,CAAC2C,GAAG,CAAChC,IAAI,CAAC;MAC7B,CAAC,CAAC;IACJ,CAAC;IACDiC,oBAAoBA,CAAClC,KAAK,EAAE;MAC1B,MAAMC,IAAI,GAAGD,KAAK,CAACE,IAAI,CAACD,IAAI;MAC5B,MAAMkC,OAAO,GAAGnC,KAAK,CAAC2B,KAAK,CAACS,UAAU,CAACnC,IAAI,CAAC;MAC5C,IAAI,CAACkC,OAAO,EAAE;QACZ/C,OAAO,CAAC6C,GAAG,CAAChC,IAAI,CAAC;MACnB,CAAC,MAAM,IAAIV,YAAY,CAACyC,GAAG,CAACG,OAAO,CAAC7D,UAAU,CAAC,EAAE;QAC/CuB,wBAAwB,CAAClB,IAAI,CAACJ,QAAQ,CAACyB,KAAK,CAAC,CAAC;MAChD;IACF,CAAC;IACDqC,oBAAoBA,CAACrC,KAAK,EAAE;MAC1B,MAAMsC,IAAI,GAAGtC,KAAK,CAACO,GAAG,CAAC,MAAM,CAAC;MAE9B,IAAI,EAAEd,UAAU,IAAI6C,IAAI,CAACC,qBAAqB,CAAC,CAAC,CAAC,EAAE;MAEnD,IAAI,CAACD,IAAI,CAACE,YAAY,CAAC,CAAC,EAAE;QACxB,MAAMF,IAAI,CAAChC,mBAAmB,CAC5B,2DACF,CAAC;MACH;MAEA,MAAM6B,OAAO,GAAGnC,KAAK,CAAC2B,KAAK,CAACS,UAAU,CAAC3C,UAAU,CAAC;MAElD,IAAI0C,OAAO,YAAPA,OAAO,CAAER,KAAK,CAACnD,IAAI,CAACiE,SAAS,CAAC,CAAC,EAAE;QACnC9C,wBAAwB,CAAChB,IAAI,CAACJ,QAAQ,CAACyB,KAAK,CAAC,CAAC;MAChD;IACF;EACF,CAAC;EAED,IAAA0C,iBAAQ,EAACrE,IAAI,CAACsE,GAAG,EAAE7C,iBAAiB,EAAEzB,IAAI,CAACsD,KAAK,CAAC;EACjD,IAAAe,iBAAQ,EAACrE,IAAI,CAACsE,GAAG,EAAEnB,gBAAgB,EAAEnD,IAAI,CAACsD,KAAK,CAAC;EAEhD,IAAI,CAACjC,UAAU,EAAE,MAAM,IAAIkD,KAAK,CAAC,qCAAqC,CAAC;EAIvEjD,wBAAwB,CAACZ,OAAO,CAAC,CAAC;EAElC,OAAO;IACLK,OAAO,EAAEyD,KAAK,CAACC,IAAI,CAAC1D,OAAO,CAAC;IAC5BE,iBAAiB,EAAEuD,KAAK,CAACC,IAAI,CAACxD,iBAAiB,CAAC;IAChDC,YAAY;IACZI,wBAAwB;IACxBD,UAAU;IACVD,UAAU;IACVI,wBAAwB;IACxBD;EACF,CAAC;AACH;AAOA,SAASmD,gBAAgBA,CACvB1E,IAAU,EACV2E,QAAwB,EACxB/B,EAAsC,EACtCgC,aAAwB,EACxBC,aAA6B,EAC7B;EACA,IAAID,aAAa,IAAI,CAAChC,EAAE,EAAE;IACxB,MAAM,IAAI2B,KAAK,CAAC,qDAAqD,CAAC;EACxE;EAEA,IAAI,CAAC3B,EAAE,EAAE;EAET,MAAM;IACJ3B,iBAAiB;IACjBC,YAAY;IACZI,wBAAwB;IACxBD,UAAU;IACVD,UAAU;IACVI,wBAAwB;IACxBD;EACF,CAAC,GAAGoD,QAAQ;EAEZ,MAAMG,gBAA8C,GAAG,CAAC,CAAC;EACzD5D,YAAY,CAACwC,OAAO,CAAC,CAAC9B,IAAI,EAAEgB,EAAE,KAAK;IACjCkC,gBAAgB,CAAClC,EAAE,CAAChB,IAAI,CAAC,GACtB,OAAOiD,aAAa,KAAK,UAAU,IAAIA,aAAa,CAACjD,IAAI,CAAC,IAAKgB,EAAE;EACtE,CAAC,CAAC;EAEF,MAAMmC,QAAgC,GAAG,CAAC,CAAC;EAC3C,MAAM1B,QAAQ,GAAG,IAAIrC,GAAG,CAAC4D,aAAa,IAAI,EAAE,CAAC;EAC7C,IAAIhC,EAAE,CAACoC,IAAI,KAAK,YAAY,EAAE3B,QAAQ,CAACO,GAAG,CAAChB,EAAE,CAAChB,IAAI,CAAC;EACnDX,iBAAiB,CAACyC,OAAO,CAAC9B,IAAI,IAAI;IAChC,IAAIqD,OAAO,GAAGrD,IAAI;IAClB,OAAOyB,QAAQ,CAACM,GAAG,CAACsB,OAAO,CAAC,EAAEA,OAAO,GAAG,GAAG,GAAGA,OAAO;IAErD,IAAIA,OAAO,KAAKrD,IAAI,EAAEmD,QAAQ,CAACnD,IAAI,CAAC,GAAGqD,OAAO;EAChD,CAAC,CAAC;EAEF,IAAIrC,EAAE,CAACoC,IAAI,KAAK,YAAY,IAAI5D,UAAU,KAAKwB,EAAE,CAAChB,IAAI,EAAE;IACtDmD,QAAQ,CAAC3D,UAAU,CAAC,GAAGwB,EAAE,CAAChB,IAAI;EAChC;EAEA,MAAM;IAAEzB;EAAK,CAAC,GAAGH,IAAI;EAIrB,MAAMkF,GAAyC,GAAG/E,IAAI,CAAC+B,GAAG,CAACb,UAAU,CAAC;EACtE,MAAM8D,IAAqC,GAAG5D,WAAW,CAAC6D,GAAG,CAACC,CAAC,IAC7DlF,IAAI,CAAC+B,GAAG,CAACmD,CAAC,CACZ,CAAC;EACD,MAAMC,eAAyC,GAC7C9D,wBAAwB,CAAC4D,GAAG,CAACC,CAAC,IAAIlF,IAAI,CAAC+B,GAAG,CAACmD,CAAC,CAAC,CAAC;EAGhD,MAAM3C,IAAI,GAAGwC,GAAG,CAAChD,GAAG,CAAC,aAAa,CAAoC;EAEtE,IAAIU,EAAE,CAACoC,IAAI,KAAK,YAAY,EAAE;IAC5BE,GAAG,CAACK,WAAW,CAAC7C,IAAI,CAAC;EACvB,CAAC,MAAM,IAAIE,EAAE,CAACoC,IAAI,KAAK,kBAAkB,EAAE;IACzC1D,wBAAwB,CAACoC,OAAO,CAAC8B,UAAU,IAAI;MAC7C,MAAMC,MAA8B,GAAGtF,IAAI,CAAC+B,GAAG,CAACsD,UAAU,CAAC;MAC3DC,MAAM,CAACF,WAAW,CAAC1F,oBAAoB,CAAC,GAAG,EAAE+C,EAAE,EAAE6C,MAAM,CAAC5D,IAAI,CAAC,CAAC;IAChE,CAAC,CAAC;IACFqD,GAAG,CAACK,WAAW,CAAC7C,IAAI,CAAC;IACrBvC,IAAI,CAACuF,aAAa,CAChB,MAAM,EACN3F,mBAAmB,CACjBF,oBAAoB,CAAC,GAAG,EAAE+C,EAAE,EAAE3C,UAAU,CAACmB,UAAU,CAAC,CACtD,CACF,CAAC;EACH,CAAC,MAAM;IACL,MAAM,IAAImD,KAAK,CAAC,2BAA2B,CAAC;EAC9C;EAEAf,MAAM,CAACC,IAAI,CAACsB,QAAQ,CAAC,CAACrB,OAAO,CAAC9B,IAAI,IAAI;IACpCzB,IAAI,CAACmD,KAAK,CAACqC,MAAM,CAAC/D,IAAI,EAAEmD,QAAQ,CAACnD,IAAI,CAAC,CAAC;EACzC,CAAC,CAAC;EAEF,KAAK,MAAMzB,IAAI,IAAIgF,IAAI,EAAEhF,IAAI,CAACyF,MAAM,CAAC,CAAC;EACtC,KAAK,MAAMzF,IAAI,IAAImF,eAAe,EAAE;IAClC,MAAMzD,IAAI,GAAG/B,SAAS,CAACgF,gBAAgB,CAAC3E,IAAI,CAAC0B,IAAI,CAACD,IAAI,CAAC,CAAC;IACxDzB,IAAI,CAACoF,WAAW,CAAC1D,IAAI,CAAC;EACxB;AACF;AAeA,MAAMgE,UAAsC,GAAGrC,MAAM,CAACsC,MAAM,CAAC,IAAI,CAAC;AAClE,SAASC,UAAUA,CAACnE,IAAY,EAAE;EAChC,IAAI,CAACiE,UAAU,CAACjE,IAAI,CAAC,EAAE;IACrB,MAAMoE,MAAM,GAAGhE,gBAAO,CAACJ,IAAI,CAAC;IAC5B,IAAI,CAACoE,MAAM,EAAE;MACX,MAAMxC,MAAM,CAACiC,MAAM,CAAC,IAAIQ,cAAc,CAAE,kBAAiBrE,IAAK,EAAC,CAAC,EAAE;QAChEsE,IAAI,EAAE,sBAAsB;QAC5BF,MAAM,EAAEpE;MACV,CAAC,CAAC;IACJ;IAEA,MAAMuE,EAAE,GAAGA,CAAA,KAAY;MACc;QACjC,IAAI,CAACvF,SAAS,EAAE;UACd,MAAMwF,QAAQ,GAAG;YAAE9B,GAAG,EAAEtE,IAAI,CAACgG,MAAM,CAAC1B,GAAG,CAAC,CAAC,CAAC;YAAEnE,IAAI,EAAE;UAAK,CAAS;UAChE,IAAAkE,iBAAQ,EAAC+B,QAAQ,CAAC9B,GAAG,EAAE;YACrBlB,OAAO,EAAEjD,IAAI,IAAI,CAACiG,QAAQ,CAACjG,IAAI,GAAGA,IAAI,EAAEkG,IAAI,CAAC;UAC/C,CAAC,CAAC;UACF,OAAOD,QAAQ;QACjB;MACF;MACA,OAAO,IAAIxF,SAAS,CAClB;QAAE0F,QAAQ,EAAG,kBAAiB1E,IAAK;MAAE,CAAC,EACtC;QACE0C,GAAG,EAAEtE,IAAI,CAACgG,MAAM,CAAC1B,GAAG,CAAC,CAAC,CAAC;QACvB4B,IAAI,EAAE,8BAA8B;QACpCK,QAAQ,EAAE;MACZ,CACF,CAAC;IACH,CAAC;IAKD,IAAI5B,QAA+B,GAAG,IAAI;IAE1CkB,UAAU,CAACjE,IAAI,CAAC,GAAG;MACjB4E,UAAU,EAAER,MAAM,CAACQ,UAAU;MAC7BC,KAAKA,CAAC5B,aAAa,EAAEjC,EAAE,EAAEgC,aAAa,EAAE;QACtC,MAAM5E,IAAI,GAAGmG,EAAE,CAAC,CAAC;QACjBxB,QAAQ,KAARA,QAAQ,GAAK7D,iBAAiB,CAACd,IAAI,CAAC;QACpC0E,gBAAgB,CAAC1E,IAAI,EAAE2E,QAAQ,EAAE/B,EAAE,EAAEgC,aAAa,EAAEC,aAAa,CAAC;QAElE,OAAO;UACL6B,KAAK,EAAE1G,IAAI,CAACsE,GAAG,CAACqC,OAAO,CAACC,IAAI;UAC5B7F,OAAO,EAAE4D,QAAQ,CAAC5D;QACpB,CAAC;MACH,CAAC;MACD8F,eAAeA,CAAA,EAAG;QAChBlC,QAAQ,KAARA,QAAQ,GAAK7D,iBAAiB,CAACqF,EAAE,CAAC,CAAC,CAAC;QACpC,OAAO3B,KAAK,CAACC,IAAI,CAACE,QAAQ,CAACzD,YAAY,CAAC4F,MAAM,CAAC,CAAC,CAAC;MACnD;IACF,CAAC;EACH;EAEA,OAAOjB,UAAU,CAACjE,IAAI,CAAC;AACzB;AAEO,SAASM,GAAGA,CACjBN,IAAY,EACZiD,aAA6B,EAC7BjC,EAAsC,EACtCgC,aAAwB,EACxB;EACA,OAAOmB,UAAU,CAACnE,IAAI,CAAC,CAAC6E,KAAK,CAAC5B,aAAa,EAAEjC,EAAE,EAAEgC,aAAa,CAAC;AACjE;AAEO,SAAS4B,UAAUA,CAAC5E,IAAY,EAAE;EACvC,OAAOmE,UAAU,CAACnE,IAAI,CAAC,CAAC4E,UAAU;AACpC;AAEO,SAASK,eAAeA,CAACjF,IAAY,EAAyB;EACnE,OAAOmE,UAAU,CAACnE,IAAI,CAAC,CAACiF,eAAe,CAAC,CAAC;AAC3C;AAEO,SAASE,MAAMA,CAACnF,IAAY,EAAEoF,YAAyB,EAAE;EAG9DpG,SAAS,KAATA,SAAS,GAAKoG,YAAY;EAE1BjB,UAAU,CAACnE,IAAI,CAAC;AAClB;AAEO,MAAMqF,IAAI,GAAAC,OAAA,CAAAD,IAAA,GAAGzD,MAAM,CAACC,IAAI,CAACzB,gBAAO,CAAC,CAACoD,GAAG,CAACxD,IAAI,IAAIA,IAAI,CAACuF,OAAO,CAAC,IAAI,EAAE,EAAE,CAAC,CAAC;AAAC,IAAAC,QAAA,GAAAF,OAAA,CAAAG,OAAA,GAE9DnF,GAAG"}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy