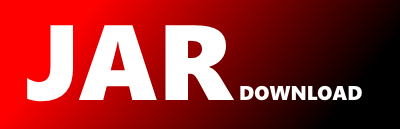
package.dist.index.mjs.map Maven / Gradle / Ivy
{"version":3,"file":"index.mjs","sources":["../../../node_modules/lodash-es/_castSlice.js","../../../node_modules/lodash-es/_hasUnicode.js","../../../node_modules/lodash-es/_asciiToArray.js","../../../node_modules/lodash-es/_unicodeToArray.js","../../../node_modules/lodash-es/_stringToArray.js","../../../node_modules/lodash-es/_createCaseFirst.js","../../../node_modules/lodash-es/upperFirst.js","../../../node_modules/lodash-es/capitalize.js","../../../node_modules/lodash-es/camelCase.js","../src/chart.ts","../src/axis-chart.ts","../src/charts/alluvial.ts","../src/charts/area.ts","../src/charts/boxplot.ts","../src/charts/bubble.ts","../src/charts/bullet.ts","../src/charts/choropleth.ts","../src/charts/circle-pack.ts","../src/charts/combo.ts","../src/charts/pie.ts","../src/charts/donut.ts","../src/charts/gauge.ts","../src/charts/bar-grouped.ts","../src/charts/heatmap.ts","../src/charts/histogram.ts","../src/charts/line.ts","../src/charts/lollipop.ts","../src/charts/meter.ts","../src/charts/radar.ts","../src/charts/scatter.ts","../src/charts/tree.ts","../src/charts/treemap.ts","../src/charts/bar-simple.ts","../src/charts/area-stacked.ts","../src/charts/bar-stacked.ts","../src/charts/wordcloud.ts"],"sourcesContent":["import baseSlice from './_baseSlice.js';\n\n/**\n * Casts `array` to a slice if it's needed.\n *\n * @private\n * @param {Array} array The array to inspect.\n * @param {number} start The start position.\n * @param {number} [end=array.length] The end position.\n * @returns {Array} Returns the cast slice.\n */\nfunction castSlice(array, start, end) {\n var length = array.length;\n end = end === undefined ? length : end;\n return (!start && end >= length) ? array : baseSlice(array, start, end);\n}\n\nexport default castSlice;\n","/** Used to compose unicode character classes. */\nvar rsAstralRange = '\\\\ud800-\\\\udfff',\n rsComboMarksRange = '\\\\u0300-\\\\u036f',\n reComboHalfMarksRange = '\\\\ufe20-\\\\ufe2f',\n rsComboSymbolsRange = '\\\\u20d0-\\\\u20ff',\n rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,\n rsVarRange = '\\\\ufe0e\\\\ufe0f';\n\n/** Used to compose unicode capture groups. */\nvar rsZWJ = '\\\\u200d';\n\n/** Used to detect strings with [zero-width joiners or code points from the astral planes](http://eev.ee/blog/2015/09/12/dark-corners-of-unicode/). */\nvar reHasUnicode = RegExp('[' + rsZWJ + rsAstralRange + rsComboRange + rsVarRange + ']');\n\n/**\n * Checks if `string` contains Unicode symbols.\n *\n * @private\n * @param {string} string The string to inspect.\n * @returns {boolean} Returns `true` if a symbol is found, else `false`.\n */\nfunction hasUnicode(string) {\n return reHasUnicode.test(string);\n}\n\nexport default hasUnicode;\n","/**\n * Converts an ASCII `string` to an array.\n *\n * @private\n * @param {string} string The string to convert.\n * @returns {Array} Returns the converted array.\n */\nfunction asciiToArray(string) {\n return string.split('');\n}\n\nexport default asciiToArray;\n","/** Used to compose unicode character classes. */\nvar rsAstralRange = '\\\\ud800-\\\\udfff',\n rsComboMarksRange = '\\\\u0300-\\\\u036f',\n reComboHalfMarksRange = '\\\\ufe20-\\\\ufe2f',\n rsComboSymbolsRange = '\\\\u20d0-\\\\u20ff',\n rsComboRange = rsComboMarksRange + reComboHalfMarksRange + rsComboSymbolsRange,\n rsVarRange = '\\\\ufe0e\\\\ufe0f';\n\n/** Used to compose unicode capture groups. */\nvar rsAstral = '[' + rsAstralRange + ']',\n rsCombo = '[' + rsComboRange + ']',\n rsFitz = '\\\\ud83c[\\\\udffb-\\\\udfff]',\n rsModifier = '(?:' + rsCombo + '|' + rsFitz + ')',\n rsNonAstral = '[^' + rsAstralRange + ']',\n rsRegional = '(?:\\\\ud83c[\\\\udde6-\\\\uddff]){2}',\n rsSurrPair = '[\\\\ud800-\\\\udbff][\\\\udc00-\\\\udfff]',\n rsZWJ = '\\\\u200d';\n\n/** Used to compose unicode regexes. */\nvar reOptMod = rsModifier + '?',\n rsOptVar = '[' + rsVarRange + ']?',\n rsOptJoin = '(?:' + rsZWJ + '(?:' + [rsNonAstral, rsRegional, rsSurrPair].join('|') + ')' + rsOptVar + reOptMod + ')*',\n rsSeq = rsOptVar + reOptMod + rsOptJoin,\n rsSymbol = '(?:' + [rsNonAstral + rsCombo + '?', rsCombo, rsRegional, rsSurrPair, rsAstral].join('|') + ')';\n\n/** Used to match [string symbols](https://mathiasbynens.be/notes/javascript-unicode). */\nvar reUnicode = RegExp(rsFitz + '(?=' + rsFitz + ')|' + rsSymbol + rsSeq, 'g');\n\n/**\n * Converts a Unicode `string` to an array.\n *\n * @private\n * @param {string} string The string to convert.\n * @returns {Array} Returns the converted array.\n */\nfunction unicodeToArray(string) {\n return string.match(reUnicode) || [];\n}\n\nexport default unicodeToArray;\n","import asciiToArray from './_asciiToArray.js';\nimport hasUnicode from './_hasUnicode.js';\nimport unicodeToArray from './_unicodeToArray.js';\n\n/**\n * Converts `string` to an array.\n *\n * @private\n * @param {string} string The string to convert.\n * @returns {Array} Returns the converted array.\n */\nfunction stringToArray(string) {\n return hasUnicode(string)\n ? unicodeToArray(string)\n : asciiToArray(string);\n}\n\nexport default stringToArray;\n","import castSlice from './_castSlice.js';\nimport hasUnicode from './_hasUnicode.js';\nimport stringToArray from './_stringToArray.js';\nimport toString from './toString.js';\n\n/**\n * Creates a function like `_.lowerFirst`.\n *\n * @private\n * @param {string} methodName The name of the `String` case method to use.\n * @returns {Function} Returns the new case function.\n */\nfunction createCaseFirst(methodName) {\n return function(string) {\n string = toString(string);\n\n var strSymbols = hasUnicode(string)\n ? stringToArray(string)\n : undefined;\n\n var chr = strSymbols\n ? strSymbols[0]\n : string.charAt(0);\n\n var trailing = strSymbols\n ? castSlice(strSymbols, 1).join('')\n : string.slice(1);\n\n return chr[methodName]() + trailing;\n };\n}\n\nexport default createCaseFirst;\n","import createCaseFirst from './_createCaseFirst.js';\n\n/**\n * Converts the first character of `string` to upper case.\n *\n * @static\n * @memberOf _\n * @since 4.0.0\n * @category String\n * @param {string} [string=''] The string to convert.\n * @returns {string} Returns the converted string.\n * @example\n *\n * _.upperFirst('fred');\n * // => 'Fred'\n *\n * _.upperFirst('FRED');\n * // => 'FRED'\n */\nvar upperFirst = createCaseFirst('toUpperCase');\n\nexport default upperFirst;\n","import toString from './toString.js';\nimport upperFirst from './upperFirst.js';\n\n/**\n * Converts the first character of `string` to upper case and the remaining\n * to lower case.\n *\n * @static\n * @memberOf _\n * @since 3.0.0\n * @category String\n * @param {string} [string=''] The string to capitalize.\n * @returns {string} Returns the capitalized string.\n * @example\n *\n * _.capitalize('FRED');\n * // => 'Fred'\n */\nfunction capitalize(string) {\n return upperFirst(toString(string).toLowerCase());\n}\n\nexport default capitalize;\n","import capitalize from './capitalize.js';\nimport createCompounder from './_createCompounder.js';\n\n/**\n * Converts `string` to [camel case](https://en.wikipedia.org/wiki/CamelCase).\n *\n * @static\n * @memberOf _\n * @since 3.0.0\n * @category String\n * @param {string} [string=''] The string to convert.\n * @returns {string} Returns the camel cased string.\n * @example\n *\n * _.camelCase('Foo Bar');\n * // => 'fooBar'\n *\n * _.camelCase('--foo-bar--');\n * // => 'fooBar'\n *\n * _.camelCase('__FOO_BAR__');\n * // => 'fooBar'\n */\nvar camelCase = createCompounder(function(result, word, index) {\n word = word.toLowerCase();\n return result + (index ? capitalize(word) : word);\n});\n\nexport default camelCase;\n","import { getProperty } from '@/tools'\nimport { ChartModel } from '@/model/model'\nimport { ChartConfig } from '@/interfaces/model'\nimport { BaseChartOptions } from '@/interfaces/charts'\nimport {\n\tLayoutGrowth,\n\tLayoutAlignItems,\n\tLayoutDirection,\n\tLegendOrientations,\n\tEvents as ChartEvents,\n\tRenderTypes\n} from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Toolbar } from '@/components/axes/toolbar'\nimport { LayoutComponent } from '@/components/layout'\nimport { Spacer } from '@/components/layout/spacer'\nimport { Modal } from '@/components/essentials/modal'\nimport { Title } from '@/components/essentials/title'\nimport { Legend } from '@/components/essentials/legend'\nimport { CanvasChartClip } from '@/components/essentials/canvas-chart-clip'\nimport { Tooltip } from '@/components/essentials/tooltip'\nimport { CanvasZoom } from '@/services/canvas-zoom'\nimport { DOMUtils } from '@/services/essentials/dom-utils'\nimport { Events } from '@/services/essentials/events'\nimport { Files } from '@/services/essentials/files'\nimport { GradientUtils } from '@/services/essentials/gradient-utils'\nimport { Transitions } from '@/services/essentials/transitions'\n\nexport class Chart {\n\tcomponents: Component[] = []\n\tservices: any = {\n\t\tcanvasZoom: CanvasZoom,\n\t\tdomUtils: DOMUtils,\n\t\tevents: Events,\n\t\tfiles: Files,\n\t\tgradientUtils: GradientUtils,\n\t\ttransitions: Transitions\n\t}\n\tmodel: ChartModel = new ChartModel(this.services)\n\n\t// eslint-disable-next-line @typescript-eslint/ban-ts-comment\n\t// @ts-ignore\n\t// eslint-disable-next-line @typescript-eslint/no-unused-vars\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\t// Allow for subclasses to override the constructor with additional parameters or initialization logic without breaking the API contract of the Chart class\n\t}\n\n\t// Contains the code that uses properties that are overridable by the super-class\n\tinit(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\t// Store the holder in the model\n\t\tthis.model.set({ holder }, { skipUpdate: true })\n\n\t\t// Initialize all services\n\t\tObject.keys(this.services).forEach(serviceName => {\n\t\t\tconst serviceObj = this.services[serviceName]\n\t\t\tthis.services[serviceName] = new serviceObj(this.model, this.services)\n\t\t})\n\n\t\t// Call update() when model has been updated\n\t\tthis.services.events.addEventListener(ChartEvents.Model.UPDATE, (e: CustomEvent) => {\n\t\t\tconst animate = !!getProperty(e, 'detail', 'animate')\n\t\t\tthis.update(animate)\n\t\t})\n\n\t\t// Set model data & options\n\t\tthis.model.setData(chartConfigs.data)\n\n\t\t// Set chart resize event listener\n\t\tthis.services.events.addEventListener(ChartEvents.Chart.RESIZE, () => {\n\t\t\tthis.update(false)\n\t\t})\n\n\t\tthis.components = this.getComponents()\n\n\t\tthis.update()\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents(): Component[] {\n\t\tconsole.error('getComponents() method is not implemented')\n\n\t\treturn []\n\t}\n\n\tupdate(animate = true) {\n\t\t// Called 4 times whenever a chart is displayed\n\t\tif (!this.components) {\n\t\t\treturn\n\t\t}\n\n\t\t// Update all services\n\t\tObject.keys(this.services).forEach((serviceName: string) => {\n\t\t\tconst serviceObj = this.services[serviceName]\n\t\t\tserviceObj.update()\n\t\t})\n\n\t\t// Render all components\n\t\tthis.components.forEach(component => component.render(animate))\n\n\t\t// Asynchronously dispatch a \"render-finished\" event\n\t\t// This is needed because of d3-transitions\n\t\t// Since at the start of the transition\n\t\t// Elements do not hold their final size or position\n\t\tconst pendingTransitions = this.services.transitions.getPendingTransitions()\n\t\tconst promises = Object.keys(pendingTransitions).map(transitionID => {\n\t\t\tconst transition = pendingTransitions[transitionID]\n\t\t\treturn transition.end().catch((e: any) => e) // Skip rejects since we don't care about those;\n\t\t})\n\n\t\tPromise.all(promises).then(() =>\n\t\t\tthis.services.events.dispatchEvent(ChartEvents.Chart.RENDER_FINISHED)\n\t\t)\n\t}\n\n\tdestroy() {\n\t\t// Call the destroy() method on all components\n\t\tthis.components.forEach(component => component.destroy())\n\n\t\t// Remove the chart holder\n\t\tthis.services.domUtils.getHolder().remove()\n\n\t\tthis.model.set({ destroyed: true }, { skipUpdate: true })\n\t}\n\n\tprotected getChartComponents(graphFrameComponents: any[], configs?: object) {\n\t\tconst options = this.model.getOptions()\n\n\t\tconst toolbarEnabled = getProperty(options, 'toolbar', 'enabled')\n\n\t\tconst legendComponent = {\n\t\t\tid: 'legend',\n\t\t\tcomponents: [new Legend(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\t// if canvas zoom is enabled\n\t\tconst isZoomEnabled = getProperty(options, 'canvasZoom', 'enabled')\n\n\t\tif (isZoomEnabled && isZoomEnabled === true) {\n\t\t\tgraphFrameComponents.push(new CanvasChartClip(this.model, this.services))\n\t\t}\n\n\t\tconst titleAvailable = !!this.model.getOptions().title\n\t\tconst titleComponent = {\n\t\t\tid: 'title',\n\t\t\tcomponents: [new Title(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst toolbarComponent = {\n\t\t\tid: 'toolbar',\n\t\t\tcomponents: [new Toolbar(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst headerComponent = {\n\t\t\tid: 'header',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t// always add title to keep layout correct\n\t\t\t\t\t\ttitleComponent,\n\t\t\t\t\t\t...(toolbarEnabled ? [toolbarComponent] : [])\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: LayoutDirection.ROW,\n\t\t\t\t\t\talignItems: LayoutAlignItems.CENTER\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst graphFrameComponent = {\n\t\t\tid: 'graph-frame',\n\t\t\tcomponents: graphFrameComponents,\n\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\trenderType: getProperty(configs, 'graphFrameRenderType') || RenderTypes.SVG\n\t\t}\n\n\t\tconst isLegendEnabled =\n\t\t\tgetProperty(configs, 'excludeLegend') !== true && options.legend.enabled !== false\n\t\t// TODO: REUSE BETWEEN AXISCHART & CHART\n\t\t// Decide the position of the legend in reference to the chart\n\t\tlet fullFrameComponentDirection = LayoutDirection.COLUMN\n\t\tif (isLegendEnabled) {\n\t\t\tconst legendPosition = getProperty(options, 'legend', 'position')\n\t\t\tif (legendPosition === 'left') {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.ROW\n\n\t\t\t\tif (!options.legend.orientation) {\n\t\t\t\t\toptions.legend.orientation = LegendOrientations.VERTICAL\n\t\t\t\t}\n\t\t\t} else if (legendPosition === 'right') {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.ROW_REVERSE\n\n\t\t\t\tif (!options.legend.orientation) {\n\t\t\t\t\toptions.legend.orientation = LegendOrientations.VERTICAL\n\t\t\t\t}\n\t\t\t} else if (legendPosition === 'bottom') {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.COLUMN_REVERSE\n\t\t\t}\n\t\t}\n\n\t\tconst legendSpacerComponent = {\n\t\t\tid: 'spacer',\n\t\t\tcomponents: [new Spacer(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst fullFrameComponent = {\n\t\t\tid: 'full-frame',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t...(isLegendEnabled ? [legendComponent] : []),\n\t\t\t\t\t\t...(isLegendEnabled ? [legendSpacerComponent] : []),\n\t\t\t\t\t\tgraphFrameComponent\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: fullFrameComponentDirection\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\t// Add chart title if it exists\n\t\tconst topLevelLayoutComponents: any[] = []\n\n\t\tif (titleAvailable || toolbarEnabled) {\n\t\t\ttopLevelLayoutComponents.push(headerComponent)\n\n\t\t\tconst titleSpacerComponent = {\n\t\t\t\tid: 'spacer',\n\t\t\t\tcomponents: [\n\t\t\t\t\tnew Spacer(this.model, this.services, toolbarEnabled ? { size: 15 } : undefined)\n\t\t\t\t],\n\t\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t\t}\n\n\t\t\ttopLevelLayoutComponents.push(titleSpacerComponent)\n\t\t}\n\t\ttopLevelLayoutComponents.push(fullFrameComponent)\n\n\t\treturn [\n\t\t\tnew Tooltip(this.model, this.services),\n\t\t\tnew Modal(this.model, this.services),\n\t\t\tnew LayoutComponent(this.model, this.services, topLevelLayoutComponents, {\n\t\t\t\tdirection: LayoutDirection.COLUMN\n\t\t\t})\n\t\t]\n\t}\n}\n","import { Chart } from './chart'\nimport { getProperty } from './tools'\nimport { ChartModelCartesian } from '@/model/cartesian-charts'\nimport { AxisChartOptions } from '@/interfaces/charts'\nimport { ChartConfig } from '@/interfaces/model'\nimport {\n\tAxisPositions,\n\tLayoutAlignItems,\n\tLayoutDirection,\n\tLayoutGrowth,\n\tLegendOrientations,\n\tLegendPositions,\n\tRenderTypes,\n\tScaleTypes\n} from '@/interfaces/enums'\nimport { ChartBrush } from '@/components/axes/grid-brush'\nimport { ChartClip } from '@/components/axes/chart-clip'\nimport { Toolbar } from '@/components/axes/toolbar'\nimport { ZoomBar } from '@/components/axes/zoom-bar'\nimport { LayoutComponent } from '@/components/layout'\nimport { Spacer } from '@/components/layout/spacer'\nimport { Modal } from '@/components/essentials/modal'\nimport { Legend } from '@/components/essentials/legend'\nimport { Threshold } from '@/components/essentials/threshold'\nimport { Highlight } from '@/components/essentials/highlights'\nimport { Title } from '@/components/essentials/title'\nimport { AxisChartsTooltip } from '@/components/essentials/tooltip-axis'\nimport { CartesianScales } from '@/services/scales-cartesian'\nimport { Curves } from '@/services/curves'\nimport { Zoom } from '@/services/zoom'\n\nexport class AxisChart extends Chart {\n\tservices: any = Object.assign(this.services, {\n\t\tcartesianScales: CartesianScales,\n\t\tcurves: Curves,\n\t\tzoom: Zoom\n\t})\n\tmodel: ChartModelCartesian = new ChartModelCartesian(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\t}\n\n\tprotected getAxisChartComponents(graphFrameComponents: any[], configs?: any) {\n\t\tconst options = this.model.getOptions()\n\t\tconst isZoomBarEnabled = getProperty(options, 'zoomBar', AxisPositions.TOP, 'enabled')\n\t\tconst toolbarEnabled = getProperty(options, 'toolbar', 'enabled')\n\n\t\tthis.services.cartesianScales.determineAxisDuality()\n\t\tthis.services.cartesianScales.findDomainAndRangeAxes() // need to do this before getMainXAxisPosition()\n\t\tthis.services.cartesianScales.determineOrientation()\n\n\t\tconst mainXAxisPosition = this.services.cartesianScales.getMainXAxisPosition()\n\t\tconst mainXScaleType = getProperty(options, 'axes', mainXAxisPosition, 'scaleType')\n\t\t// @todo - Zoom Bar only supports main axis at BOTTOM axis and time scale for now\n\t\tconst zoomBarEnabled =\n\t\t\tisZoomBarEnabled &&\n\t\t\tmainXAxisPosition === AxisPositions.BOTTOM &&\n\t\t\tmainXScaleType === ScaleTypes.TIME\n\n\t\t// @todo - should check if zoom bar in all axes are locked\n\t\tconst isZoomBarLocked = this.services.zoom.isZoomBarLocked(AxisPositions.TOP)\n\n\t\tconst titleAvailable = !!this.model.getOptions().title\n\t\tconst titleComponent = {\n\t\t\tid: 'title',\n\t\t\tcomponents: [new Title(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst toolbarComponent = {\n\t\t\tid: 'toolbar',\n\t\t\tcomponents: [new Toolbar(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst headerComponent = {\n\t\t\tid: 'header',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t// always add title to keep layout correct\n\t\t\t\t\t\ttitleComponent,\n\t\t\t\t\t\t...(toolbarEnabled ? [toolbarComponent] : [])\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: LayoutDirection.ROW,\n\t\t\t\t\t\talignItems: LayoutAlignItems.CENTER\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst legendComponent = {\n\t\t\tid: 'legend',\n\t\t\tcomponents: [new Legend(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\t// if all zoom bars are locked, no need to add chart brush\n\t\tif (zoomBarEnabled && !isZoomBarLocked) {\n\t\t\tgraphFrameComponents.push(\n\t\t\t\tnew ChartClip(this.model, this.services),\n\t\t\t\tnew ChartBrush(this.model, this.services)\n\t\t\t)\n\t\t}\n\n\t\tgraphFrameComponents.push(new Threshold(this.model, this.services))\n\t\tgraphFrameComponents.push(new Highlight(this.model, this.services))\n\n\t\tconst graphFrameComponent = {\n\t\t\tid: 'graph-frame',\n\t\t\tcomponents: graphFrameComponents,\n\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst isLegendEnabled =\n\t\t\tgetProperty(configs, 'legend', 'enabled') !== false &&\n\t\t\tthis.model.getOptions().legend.enabled !== false\n\n\t\t// Decide the position of the legend in reference to the chart\n\t\tlet fullFrameComponentDirection = LayoutDirection.COLUMN\n\t\tif (isLegendEnabled) {\n\t\t\tconst legendPosition = getProperty(this.model.getOptions(), 'legend', 'position')\n\t\t\tif (legendPosition === LegendPositions.LEFT) {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.ROW\n\n\t\t\t\tif (!this.model.getOptions().legend.orientation) {\n\t\t\t\t\tthis.model.getOptions().legend.orientation = LegendOrientations.VERTICAL\n\t\t\t\t}\n\t\t\t} else if (legendPosition === LegendPositions.RIGHT) {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.ROW_REVERSE\n\n\t\t\t\tif (!this.model.getOptions().legend.orientation) {\n\t\t\t\t\tthis.model.getOptions().legend.orientation = LegendOrientations.VERTICAL\n\t\t\t\t}\n\t\t\t} else if (legendPosition === LegendPositions.BOTTOM) {\n\t\t\t\tfullFrameComponentDirection = LayoutDirection.COLUMN_REVERSE\n\t\t\t}\n\t\t}\n\n\t\tconst legendSpacerComponent = {\n\t\t\tid: 'spacer',\n\t\t\tcomponents: [new Spacer(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst fullFrameComponent = {\n\t\t\tid: 'full-frame',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t...(isLegendEnabled ? [legendComponent] : []),\n\t\t\t\t\t\t...(isLegendEnabled ? [legendSpacerComponent] : []),\n\t\t\t\t\t\tgraphFrameComponent\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: fullFrameComponentDirection\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst zoomBarComponent = {\n\t\t\tid: 'zoom-bar',\n\t\t\tcomponents: [new ZoomBar(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst topLevelLayoutComponents = []\n\t\t// header component is required for either title or toolbar\n\t\tif (titleAvailable || toolbarEnabled) {\n\t\t\ttopLevelLayoutComponents.push(headerComponent)\n\n\t\t\tconst titleSpacerComponent = {\n\t\t\t\tid: 'spacer',\n\t\t\t\tcomponents: [\n\t\t\t\t\tnew Spacer(this.model, this.services, toolbarEnabled ? { size: 15 } : undefined)\n\t\t\t\t],\n\t\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t\t}\n\n\t\t\ttopLevelLayoutComponents.push(titleSpacerComponent)\n\t\t}\n\t\tif (zoomBarEnabled) {\n\t\t\ttopLevelLayoutComponents.push(zoomBarComponent)\n\t\t}\n\t\ttopLevelLayoutComponents.push(fullFrameComponent)\n\n\t\treturn [\n\t\t\tnew AxisChartsTooltip(this.model, this.services),\n\t\t\tnew Modal(this.model, this.services),\n\t\t\tnew LayoutComponent(this.model, this.services, topLevelLayoutComponents, {\n\t\t\t\tdirection: LayoutDirection.COLUMN\n\t\t\t})\n\t\t]\n\t}\n}\n","import { Chart } from '@/chart'\nimport { AlluvialChartModel } from '@/model/alluvial'\nimport { options } from '@/configuration'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { AlluvialChartOptions } from '@/interfaces/charts'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { Alluvial } from '@/components/graphs/alluvial'\nimport type { Component } from '@/components/component'\n\nexport class AlluvialChart extends Chart {\n\tmodel = new AlluvialChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.alluvialChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Alluvial(this.model, this.services)]\n\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents, {\n\t\t\texcludeLegend: true\n\t\t})\n\t\treturn components\n\t}\n}\n","import { cloneDeep } from 'lodash-es'\nimport { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { AreaChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Area } from '@/components/graphs/area'\nimport { Line } from '@/components/graphs/line'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Scatter } from '@/components/graphs/scatter'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class AreaChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(\n\t\t\tmergeDefaultChartOptions(cloneDeep(options.areaChart), chartConfigs.options)\n\t\t)\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Ruler(this.model, this.services),\n\t\t\tnew Line(this.model, this.services),\n\t\t\tnew Area(this.model, this.services),\n\t\t\tnew Scatter(this.model, this.services, {\n\t\t\t\tfadeInOnChartHolderMouseover: true,\n\t\t\t\thandleThresholds: true\n\t\t\t}),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { BoxplotChartModel } from '@/model/boxplot'\nimport type { BoxplotChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Boxplot } from '@/components/graphs/boxplot'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class BoxplotChart extends AxisChart {\n\tmodel = new BoxplotChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.boxplotChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Boxplot(this.model, this.services),\n\t\t\tnew ZeroLine(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.VERT_OR_HORIZ\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents, {\n\t\t\tlegend: {\n\t\t\t\tenabled: false\n\t\t\t}\n\t\t})\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { BubbleChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Bubble } from '@/components/graphs/bubble'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class BubbleChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.bubbleChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Ruler(this.model, this.services),\n\t\t\tnew Bubble(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { BulletChartModel } from '@/model/bullet'\nimport type { BulletChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport { Bullet } from '@/components/graphs/bullet'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class BulletChart extends AxisChart {\n\tmodel = new BulletChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.bulletChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Bullet(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options as configOptions } from '@/configuration'\nimport { getProperty, mergeDefaultChartOptions } from '@/tools'\nimport { ChoroplethModel } from '@/model/choropleth'\nimport type { ChoroplethChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { LayoutDirection, LayoutGrowth, RenderTypes, LayoutAlignItems } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Choropleth } from '@/components/graphs/choropleth'\nimport { Modal } from '@/components/essentials/modal'\nimport { LayoutComponent } from '@/components/layout/layout'\nimport { ColorScaleLegend } from '@/components/essentials/color-scale-legend'\nimport { Title } from '@/components/essentials/title'\nimport { Spacer } from '@/components/layout/spacer'\nimport { Toolbar } from '@/components/axes/toolbar'\nimport { Tooltip } from '@/components/essentials/tooltip'\n\nexport class ChoroplethChart extends Chart {\n\tmodel = new ChoroplethModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(\n\t\t\tmergeDefaultChartOptions(configOptions.choroplethChart, chartConfigs.options)\n\t\t)\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t// Custom getChartComponents - Implements getChartComponents\n\t// Removes zoombar support and additional `features` that are not supported in heatmap\n\tprotected getChartComponents(graphFrameComponents: any[], configs?: any) {\n\t\tconst options = this.model.getOptions()\n\t\tconst toolbarEnabled = getProperty(options, 'toolbar', 'enabled')\n\n\t\tconst titleAvailable = !!this.model.getOptions().title\n\t\tconst titleComponent = {\n\t\t\tid: 'title',\n\t\t\tcomponents: [new Title(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst toolbarComponent = {\n\t\t\tid: 'toolbar',\n\t\t\tcomponents: [new Toolbar(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst headerComponent = {\n\t\t\tid: 'header',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t// always add title to keep layout correct\n\t\t\t\t\t\ttitleComponent,\n\t\t\t\t\t\t...(toolbarEnabled ? [toolbarComponent] : [])\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: LayoutDirection.ROW,\n\t\t\t\t\t\talignItems: LayoutAlignItems.CENTER\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst legendComponent = {\n\t\t\tid: 'legend',\n\t\t\tcomponents: [\n\t\t\t\tnew ColorScaleLegend(this.model, this.services, {\n\t\t\t\t\tchartType: 'choropleth'\n\t\t\t\t})\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst graphFrameComponent = {\n\t\t\tid: 'graph-frame',\n\t\t\tcomponents: graphFrameComponents,\n\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst isLegendEnabled =\n\t\t\tgetProperty(configs, 'legend', 'enabled') !== false &&\n\t\t\tthis.model.getOptions().legend.enabled !== false &&\n\t\t\tthis.model.getData().length > 0\n\n\t\t// Decide the position of the legend in reference to the chart\n\t\tconst fullFrameComponentDirection = LayoutDirection.COLUMN_REVERSE\n\n\t\tconst legendSpacerComponent = {\n\t\t\tid: 'spacer',\n\t\t\tcomponents: [new Spacer(this.model, this.services, { size: 15 })],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst fullFrameComponent = {\n\t\t\tid: 'full-frame',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t...(isLegendEnabled ? [legendComponent] : []),\n\t\t\t\t\t\t...(isLegendEnabled ? [legendSpacerComponent] : []),\n\t\t\t\t\t\tgraphFrameComponent\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: fullFrameComponentDirection\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst topLevelLayoutComponents = []\n\t\t// header component is required for either title or toolbar\n\t\tif (titleAvailable || toolbarEnabled) {\n\t\t\ttopLevelLayoutComponents.push(headerComponent)\n\n\t\t\tconst titleSpacerComponent = {\n\t\t\t\tid: 'spacer',\n\t\t\t\tcomponents: [\n\t\t\t\t\tnew Spacer(this.model, this.services, toolbarEnabled ? { size: 15 } : undefined)\n\t\t\t\t],\n\t\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t\t}\n\n\t\t\ttopLevelLayoutComponents.push(titleSpacerComponent)\n\t\t}\n\t\ttopLevelLayoutComponents.push(fullFrameComponent)\n\n\t\treturn [\n\t\t\tnew Tooltip(this.model, this.services),\n\t\t\tnew Modal(this.model, this.services),\n\t\t\tnew LayoutComponent(this.model, this.services, topLevelLayoutComponents, {\n\t\t\t\tdirection: LayoutDirection.COLUMN\n\t\t\t})\n\t\t]\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Choropleth(this.model, this.services)]\n\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { CirclePackChartModel } from '@/model/circle-pack'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { CirclePackChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { CirclePack } from '@/components/graphs/circle-pack'\n\nexport class CirclePackChart extends Chart {\n\tmodel = new CirclePackChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.circlePackChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new CirclePack(this.model, this.services)]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { camelCase, flatten, merge } from 'lodash-es'\nimport { AxisChart } from '@/axis-chart'\nimport { options as configOptions } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { ChartTypes, Skeletons } from '@/interfaces/enums'\nimport type { ComboChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Line } from '@/components/graphs/line'\nimport { Skeleton } from '@/components/graphs/skeleton'\nimport { SimpleBar } from '@/components/graphs/bar-simple'\nimport { GroupedBar } from '@/components/graphs/bar-grouped'\nimport { StackedRuler } from '@/components/axes/ruler-stacked'\nimport { Area } from '@/components/graphs/area'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Scatter } from '@/components/graphs/scatter'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { StackedArea } from '@/components/graphs/area-stacked'\nimport { StackedBar } from '@/components/graphs/bar-stacked'\nimport { StackedScatter } from '@/components/graphs/scatter-stacked'\n\nconst graphComponentsMap = {\n\t[ChartTypes.LINE]: [Line, Scatter],\n\t[ChartTypes.SCATTER]: [Scatter],\n\t[ChartTypes.AREA]: [Area, Line, Scatter],\n\t[ChartTypes.STACKED_AREA]: [StackedArea, Line, StackedScatter, StackedRuler],\n\t[ChartTypes.SIMPLE_BAR]: [SimpleBar],\n\t[ChartTypes.GROUPED_BAR]: [GroupedBar, ZeroLine],\n\t[ChartTypes.STACKED_BAR]: [StackedBar, StackedRuler]\n}\n\nexport class ComboChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tconst chartOptions = mergeDefaultChartOptions(configOptions.comboChart, chartConfigs.options)\n\n\t\t// Warn user if no comboChartTypes defined\n\t\t// Use skeleton chart instead\n\t\tif (!chartConfigs.options.comboChartTypes) {\n\t\t\tconsole.error('No comboChartTypes defined for the Combo Chart!')\n\t\t\t// add a default chart to get an empty chart\n\t\t\tchartOptions.comboChartTypes = [{ type: ChartTypes.LINE, correspondingDatasets: [] }]\n\t\t}\n\n\t\t// set the global options\n\t\tthis.model.setOptions(chartOptions)\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\tgetGraphComponents() {\n\t\tconst { comboChartTypes }: { comboChartTypes: Component[] } = this.model.getOptions()\n\t\tlet counter = 0\n\t\tconst graphComponents: Component[] = comboChartTypes\n\t\t\t.map((graph: any) => {\n\t\t\t\tconst type = graph.type\n\t\t\t\tlet options: any\n\n\t\t\t\t// initializes the components using input strings with the base configs for each chart\n\t\t\t\tif (typeof graph.type === 'string') {\n\t\t\t\t\t// check if it is in the components map\n\t\t\t\t\t// if it isn't then it is not a valid carbon chart to use in combo\n\t\t\t\t\tif (!Object.keys(graphComponentsMap).includes(graph.type)) {\n\t\t\t\t\t\tconsole.error(\n\t\t\t\t\t\t\t`Invalid chart type \"${graph.type}\" specified for combo chart. Please refer to the ComboChart tutorial for more guidance.`\n\t\t\t\t\t\t)\n\t\t\t\t\t\treturn null\n\t\t\t\t\t}\n\t\t\t\t\tlet stacked = false\n\t\t\t\t\tconst key = `${camelCase(graph.type)}Chart` as keyof typeof configOptions\n\t\t\t\t\toptions = merge({}, configOptions[key], this.model.getOptions(), graph.options)\n\t\t\t\t\t// if we are creating a stacked area, the contained Line chart needs to know it is stacked\n\t\t\t\t\tif (graph.type === ChartTypes.STACKED_AREA) {\n\t\t\t\t\t\tstacked = true\n\t\t\t\t\t}\n\t\t\t\t\treturn graphComponentsMap[graph.type as keyof typeof graphComponentsMap].map(\n\t\t\t\t\t\t(Component: any) =>\n\t\t\t\t\t\t\tnew Component(this.model, this.services, {\n\t\t\t\t\t\t\t\tgroups: graph.correspondingDatasets,\n\t\t\t\t\t\t\t\tid: counter++,\n\t\t\t\t\t\t\t\toptions: options,\n\t\t\t\t\t\t\t\tstacked\n\t\t\t\t\t\t\t})\n\t\t\t\t\t)\n\t\t\t\t} else {\n\t\t\t\t\t// user has imported a type or custom component to instantiate\n\t\t\t\t\toptions = merge({}, this.model.getOptions(), graph.options)\n\t\t\t\t\treturn new type(this.model, this.services, {\n\t\t\t\t\t\tgroups: graph.correspondingDatasets,\n\t\t\t\t\t\tid: counter++,\n\t\t\t\t\t\toptions: options\n\t\t\t\t\t})\n\t\t\t\t}\n\t\t\t})\n\t\t\t.filter((item: any) => item !== null)\n\n\t\treturn flatten(graphComponents)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst { comboChartTypes } = this.model.getOptions()\n\t\t// don't add the regular ruler if stacked ruler is added\n\t\tconst stackedRulerEnabled = comboChartTypes.some(\n\t\t\t(chartObject: any) =>\n\t\t\t\tchartObject.type === ChartTypes.STACKED_BAR || chartObject.type === ChartTypes.STACKED_AREA\n\t\t)\n\n\t\t// Specify what to render inside the graph-frame\n\t\tconst graphFrameComponents = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t}),\n\t\t\t...(stackedRulerEnabled ? [] : [new Ruler(this.model, this.services)]),\n\t\t\t...this.getGraphComponents()\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { PieChartModel } from '@/model/pie'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { PieChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Pie } from '@/components/graphs/pie'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class PieChart extends Chart {\n\tmodel = new PieChartModel(this.services)\n\n\t// TODO - Optimize the use of \"extending\"\n\tconstructor(\n\t\tholder: HTMLDivElement,\n\t\tchartConfigs: ChartConfig,\n\t\textending = false\n\t) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// TODO - Optimize the use of \"extending\"\n\t\tif (extending) {\n\t\t\treturn\n\t\t}\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.pieChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew Pie(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.PIE\n\t\t\t})\n\t\t]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { PieChart } from './pie'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { PieChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Donut } from '@/components/graphs/donut'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class DonutChart extends PieChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs, true)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.donutChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew Donut(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.DONUT\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { GaugeChartOptions } from '@/interfaces/charts'\nimport { GaugeChartModel } from '@/model/gauge'\nimport type { Component } from '@/components/component'\nimport { Gauge } from '@/components/graphs/gauge'\n\nexport class GaugeChart extends Chart {\n\tmodel = new GaugeChartModel(this.services)\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.gaugeChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Gauge(this.model, this.services)]\n\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { BarChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { Skeleton } from '@/components/graphs/skeleton'\nimport { GroupedBar } from '@/components/graphs/bar-grouped'\n\nexport class GroupedBarChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.groupedBarChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew GroupedBar(this.model, this.services),\n\t\t\tnew ZeroLine(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.VERT_OR_HORIZ\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options as configOptions } from '@/configuration'\nimport { getProperty, mergeDefaultChartOptions } from '@/tools'\nimport { HeatmapModel } from '@/model/heatmap'\nimport type { HeatmapChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { LayoutDirection, LayoutGrowth, RenderTypes, LayoutAlignItems } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Heatmap } from '@/components/graphs/heatmap'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Modal } from '@/components/essentials/modal'\nimport { LayoutComponent } from '@/components/layout/layout'\nimport { ColorScaleLegend } from '@/components/essentials/color-scale-legend'\nimport { Title } from '@/components/essentials/title'\nimport { AxisChartsTooltip } from '@/components/essentials/tooltip-axis'\nimport { Spacer } from '@/components/layout/spacer'\nimport { Toolbar } from '@/components/axes/toolbar'\n\nexport class HeatmapChart extends AxisChart {\n\tmodel = new HeatmapModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(\n\t\t\tmergeDefaultChartOptions(configOptions.heatmapChart, chartConfigs.options)\n\t\t)\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t// Custom getChartComponents - Implements getChartComponents\n\t// Removes zoombar support and additional `features` that are not supported in heatmap\n\tprotected getAxisChartComponents(graphFrameComponents: any[], configs?: any) {\n\t\tconst options = this.model.getOptions()\n\t\tconst toolbarEnabled = getProperty(options, 'toolbar', 'enabled')\n\n\t\tthis.services.cartesianScales.determineAxisDuality()\n\t\tthis.services.cartesianScales.findDomainAndRangeAxes() // need to do this before getMainXAxisPosition()\n\t\tthis.services.cartesianScales.determineOrientation()\n\n\t\tconst titleAvailable = !!this.model.getOptions().title\n\t\tconst titleComponent = {\n\t\t\tid: 'title',\n\t\t\tcomponents: [new Title(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst toolbarComponent = {\n\t\t\tid: 'toolbar',\n\t\t\tcomponents: [new Toolbar(this.model, this.services)],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst headerComponent = {\n\t\t\tid: 'header',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t// always add title to keep layout correct\n\t\t\t\t\t\ttitleComponent,\n\t\t\t\t\t\t...(toolbarEnabled ? [toolbarComponent] : [])\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: LayoutDirection.ROW,\n\t\t\t\t\t\talignItems: LayoutAlignItems.CENTER\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst legendComponent = {\n\t\t\tid: 'legend',\n\t\t\tcomponents: [\n\t\t\t\tnew ColorScaleLegend(this.model, this.services, {\n\t\t\t\t\tchartType: 'heatmap'\n\t\t\t\t})\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.PREFERRED,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst graphFrameComponent = {\n\t\t\tid: 'graph-frame',\n\t\t\tcomponents: graphFrameComponents,\n\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\trenderType: RenderTypes.SVG\n\t\t}\n\n\t\tconst isLegendEnabled =\n\t\t\tgetProperty(configs, 'legend', 'enabled') !== false &&\n\t\t\tthis.model.getOptions().legend.enabled !== false &&\n\t\t\tthis.model.getData().length > 0\n\n\t\t// Decide the position of the legend in reference to the chart\n\t\tconst fullFrameComponentDirection = LayoutDirection.COLUMN_REVERSE\n\n\t\tconst legendSpacerComponent = {\n\t\t\tid: 'spacer',\n\t\t\tcomponents: [new Spacer(this.model, this.services, { size: 15 })],\n\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t}\n\n\t\tconst fullFrameComponent = {\n\t\t\tid: 'full-frame',\n\t\t\tcomponents: [\n\t\t\t\tnew LayoutComponent(\n\t\t\t\t\tthis.model,\n\t\t\t\t\tthis.services,\n\t\t\t\t\t[\n\t\t\t\t\t\t...(isLegendEnabled ? [legendComponent] : []),\n\t\t\t\t\t\t...(isLegendEnabled ? [legendSpacerComponent] : []),\n\t\t\t\t\t\tgraphFrameComponent\n\t\t\t\t\t],\n\t\t\t\t\t{\n\t\t\t\t\t\tdirection: fullFrameComponentDirection\n\t\t\t\t\t}\n\t\t\t\t)\n\t\t\t],\n\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t}\n\n\t\tconst topLevelLayoutComponents: any[] = []\n\t\t// header component is required for either title or toolbar\n\t\tif (titleAvailable || toolbarEnabled) {\n\t\t\ttopLevelLayoutComponents.push(headerComponent)\n\n\t\t\tconst titleSpacerComponent = {\n\t\t\t\tid: 'spacer',\n\t\t\t\tcomponents: [\n\t\t\t\t\tnew Spacer(this.model, this.services, toolbarEnabled ? { size: 15 } : undefined)\n\t\t\t\t],\n\t\t\t\tgrowth: LayoutGrowth.PREFERRED\n\t\t\t}\n\n\t\t\ttopLevelLayoutComponents.push(titleSpacerComponent)\n\t\t}\n\t\ttopLevelLayoutComponents.push(fullFrameComponent)\n\n\t\treturn [\n\t\t\tnew AxisChartsTooltip(this.model, this.services),\n\t\t\tnew Modal(this.model, this.services),\n\t\t\tnew LayoutComponent(this.model, this.services, topLevelLayoutComponents, {\n\t\t\t\tdirection: LayoutDirection.COLUMN\n\t\t\t})\n\t\t]\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Heatmap(this.model, this.services)\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { ChartModelBinned } from '@/model/binned-charts'\nimport type { HistogramChartOptions } from '@/interfaces/charts'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Histogram } from '@/components/graphs/histogram'\nimport { BinnedRuler } from '@/components/axes/ruler-binned'\n\nexport class HistogramChart extends AxisChart {\n\tmodel = new ChartModelBinned(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.histogramChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\n\t\tthis.update()\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew BinnedRuler(this.model, this.services),\n\t\t\tnew Histogram(this.model, this.services)\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { LineChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Line } from '@/components/graphs/line'\nimport { Scatter } from '@/components/graphs/scatter'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { SkeletonLines } from '@/components/graphs/skeleton-lines'\n\nexport class LineChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.lineChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Ruler(this.model, this.services),\n\t\t\tnew Line(this.model, this.services),\n\t\t\tnew Scatter(this.model, this.services, { handleThresholds: true }),\n\t\t\tnew SkeletonLines(this.model, this.services),\n\t\t\tnew ZeroLine(this.model, this.services)\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { LollipopChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Scatter } from '@/components/graphs/scatter'\nimport { Lollipop } from '@/components/graphs/lollipop'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class LollipopChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.lollipopChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Ruler(this.model, this.services),\n\t\t\tnew Lollipop(this.model, this.services),\n\t\t\tnew Scatter(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { cloneDeep, merge } from 'lodash-es'\nimport { Chart } from '@/chart'\nimport { options as configOptions } from '@/configuration'\nimport { getProperty } from '@/tools'\nimport { MeterChartModel } from '@/model/meter'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { MeterChartOptions } from '@/interfaces/charts'\nimport { LayoutGrowth, LayoutDirection, RenderTypes } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { LayoutComponent } from '@/components/layout'\nimport { Meter } from '@/components/graphs/meter'\nimport { MeterTitle } from '@/components/essentials/title-meter'\nimport { Spacer } from '@/components/layout/spacer'\n\nexport class MeterChart extends Chart {\n\tmodel = new MeterChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// use prop meter options or regular meter options\n\t\tconst options = chartConfigs.options.meter?.proportional\n\t\t\t? merge(cloneDeep(configOptions.proportionalMeterChart), chartConfigs.options)\n\t\t\t: merge(cloneDeep(configOptions.meterChart), chartConfigs.options)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(options)\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst showLabels = getProperty(this.model.getOptions(), 'meter', 'showLabels')\n\t\tconst meterComponents = [\n\t\t\t...(showLabels\n\t\t\t\t? [\n\t\t\t\t\t\t// Meter has a unique dataset title within the graph\n\t\t\t\t\t\t{\n\t\t\t\t\t\t\tid: 'meter-title',\n\t\t\t\t\t\t\tcomponents: [new MeterTitle(this.model, this.services)],\n\t\t\t\t\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\t\t\t\t\trenderType: RenderTypes.SVG\n\t\t\t\t\t\t},\n\t\t\t\t\t\t// Create the title spacer\n\t\t\t\t\t\t{\n\t\t\t\t\t\t\tid: 'spacer',\n\t\t\t\t\t\t\tcomponents: [\n\t\t\t\t\t\t\t\tnew Spacer(this.model, this.services, {\n\t\t\t\t\t\t\t\t\tsize: 8\n\t\t\t\t\t\t\t\t})\n\t\t\t\t\t\t\t],\n\t\t\t\t\t\t\tgrowth: LayoutGrowth.STRETCH\n\t\t\t\t\t\t}\n\t\t\t\t\t]\n\t\t\t\t: []),\n\t\t\t// Specify what to render inside the graph only\n\t\t\t{\n\t\t\t\tid: 'meter-graph',\n\t\t\t\tcomponents: [new Meter(this.model, this.services)],\n\t\t\t\tgrowth: LayoutGrowth.STRETCH,\n\t\t\t\trenderType: RenderTypes.SVG\n\t\t\t}\n\t\t]\n\n\t\t// the graph frame for meter includes the custom title (and spacer)\n\t\tconst graphFrame: Component[] = [\n\t\t\tnew LayoutComponent(this.model, this.services, meterComponents, {\n\t\t\t\tdirection: LayoutDirection.COLUMN\n\t\t\t})\n\t\t]\n\n\t\t// add the meter title as a top level component\n\t\tconst components: Component[] = this.getChartComponents(graphFrame, {\n\t\t\tgraphFrameRenderType: RenderTypes.HTML\n\t\t})\n\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { RadarChartModel } from '@/model/radar'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { RadarChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Radar } from '@/components/graphs/radar'\n\nexport class RadarChart extends Chart {\n\tmodel = new RadarChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.radarChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Radar(this.model, this.services)]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { ScatterChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { Ruler } from '@/components/axes/ruler'\nimport { Scatter } from '@/components/graphs/scatter'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class ScatterChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.scatterChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew Ruler(this.model, this.services),\n\t\t\tnew Scatter(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { TreeChartModel } from '@/model/tree'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { TreeChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Tree } from '@/components/graphs/tree'\n\nexport class TreeChart extends Chart {\n\tmodel = new TreeChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.treeChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Tree(this.model, this.services)]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents, {\n\t\t\texcludeLegend: true\n\t\t})\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { TreemapChartModel } from '@/model/treemap'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { TreemapChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Treemap } from '@/components/graphs/treemap'\n\nexport class TreemapChart extends Chart {\n\tmodel = new TreemapChartModel(this.services)\n\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.treemapChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [new Treemap(this.model, this.services)]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { BarChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Skeleton } from '@/components/graphs/skeleton'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { SimpleBar } from '@/components/graphs/bar-simple'\n\nexport class SimpleBarChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.simpleBarChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew SimpleBar(this.model, this.services),\n\t\t\tnew ZeroLine(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.VERT_OR_HORIZ\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { Component } from '@/components/component'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { AreaChartOptions } from '@/interfaces/charts'\nimport { Skeletons } from '@/interfaces/enums'\nimport { Grid } from '@/components/axes/grid'\nimport { StackedArea } from '@/components/graphs/area-stacked'\nimport { StackedRuler } from '@/components/axes/ruler-stacked'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { Line } from '@/components/graphs/line'\nimport { StackedScatter } from '@/components/graphs/scatter-stacked'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class StackedAreaChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.stackedAreaChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew StackedRuler(this.model, this.services),\n\t\t\tnew StackedArea(this.model, this.services),\n\t\t\tnew Line(this.model, this.services, { stacked: true }),\n\t\t\tnew StackedScatter(this.model, this.services, {\n\t\t\t\tfadeInOnChartHolderMouseover: true,\n\t\t\t\thandleThresholds: true,\n\t\t\t\tstacked: true\n\t\t\t}),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.GRID\n\t\t\t})\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { AxisChart } from '@/axis-chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport type { ChartConfig } from '@/interfaces/model'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { BarChartOptions } from '@/interfaces/charts'\nimport type { Component } from '@/components/component'\nimport { Grid } from '@/components/axes/grid'\nimport { StackedBar } from '@/components/graphs/bar-stacked'\nimport { StackedRuler } from '@/components/axes/ruler-stacked'\nimport { TwoDimensionalAxes } from '@/components/axes/two-dimensional-axes'\nimport { ZeroLine } from '@/components/axes/zero-line'\nimport { Skeleton } from '@/components/graphs/skeleton'\n\nexport class StackedBarChart extends AxisChart {\n\tconstructor(holder: HTMLDivElement, chartConfigs: ChartConfig) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.stackedBarChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew TwoDimensionalAxes(this.model, this.services),\n\t\t\tnew Grid(this.model, this.services),\n\t\t\tnew StackedRuler(this.model, this.services),\n\t\t\tnew StackedBar(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.VERT_OR_HORIZ\n\t\t\t}),\n\t\t\tnew ZeroLine(this.model, this.services)\n\t\t]\n\n\t\tconst components: Component[] = this.getAxisChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n","import { Chart } from '@/chart'\nimport { options } from '@/configuration'\nimport { mergeDefaultChartOptions } from '@/tools'\nimport { WordCloudModel } from '@/model/wordcloud'\nimport type { ChartConfig } from '@/interfaces/model'\nimport type { WordCloudChartOptions } from '@/interfaces/charts'\nimport type { WorldCloudChartOptions } from '@/interfaces'\nimport { Skeletons } from '@/interfaces/enums'\nimport type { Component } from '@/components/component'\nimport { Skeleton } from '@/components/graphs/skeleton'\nimport { WordCloud } from '@/components/graphs/wordcloud'\n\nexport class WordCloudChart extends Chart {\n\tmodel = new WordCloudModel(this.services)\n\n\tconstructor(\n\t\tholder: HTMLDivElement,\n\t\tchartConfigs: ChartConfig\n\t) {\n\t\tsuper(holder, chartConfigs)\n\n\t\t// Merge the default options for this chart\n\t\t// With the user provided options\n\t\tthis.model.setOptions(mergeDefaultChartOptions(options.wordCloudChart, chartConfigs.options))\n\n\t\t// Initialize data, services, components etc.\n\t\tthis.init(holder, chartConfigs)\n\t}\n\n\t/**\n\t * Retrieves the components to be rendered inside the graph frame.\n\t *\n\t * @returns {Component[]} An array of components to be rendered.\n\t */\n\tgetComponents() {\n\t\tconst graphFrameComponents: Component[] = [\n\t\t\tnew WordCloud(this.model, this.services),\n\t\t\tnew Skeleton(this.model, this.services, {\n\t\t\t\tskeleton: Skeletons.PIE\n\t\t\t})\n\t\t]\n\n\t\t// get the base chart components and export with tooltip\n\t\tconst components: Component[] = this.getChartComponents(graphFrameComponents)\n\t\treturn components\n\t}\n}\n"],"names":["castSlice","array","start","end","length","baseSlice","rsAstralRange","rsComboMarksRange","reComboHalfMarksRange","rsComboSymbolsRange","rsComboRange","rsVarRange","rsZWJ","reHasUnicode","hasUnicode","string","asciiToArray","rsAstral","rsCombo","rsFitz","rsModifier","rsNonAstral","rsRegional","rsSurrPair","reOptMod","rsOptVar","rsOptJoin","rsSeq","rsSymbol","reUnicode","unicodeToArray","stringToArray","createCaseFirst","methodName","toString","strSymbols","chr","trailing","upperFirst","capitalize","camelCase","createCompounder","result","word","index","Chart","holder","chartConfigs","CanvasZoom","DOMUtils","Events","Files","GradientUtils","Transitions","ChartModel","serviceName","serviceObj","ChartEvents","e","animate","getProperty","component","pendingTransitions","promises","transitionID","graphFrameComponents","configs","options","toolbarEnabled","legendComponent","Legend","LayoutGrowth","isZoomEnabled","CanvasChartClip","titleAvailable","titleComponent","Title","toolbarComponent","Toolbar","headerComponent","LayoutComponent","LayoutDirection","LayoutAlignItems","graphFrameComponent","RenderTypes","isLegendEnabled","fullFrameComponentDirection","legendPosition","LegendOrientations","legendSpacerComponent","Spacer","fullFrameComponent","topLevelLayoutComponents","titleSpacerComponent","Tooltip","Modal","AxisChart","CartesianScales","Curves","Zoom","ChartModelCartesian","isZoomBarEnabled","AxisPositions","mainXAxisPosition","mainXScaleType","zoomBarEnabled","ScaleTypes","isZoomBarLocked","ChartClip","ChartBrush","Threshold","Highlight","LegendPositions","zoomBarComponent","ZoomBar","AxisChartsTooltip","AlluvialChart","AlluvialChartModel","mergeDefaultChartOptions","Alluvial","AreaChart","cloneDeep","TwoDimensionalAxes","Grid","Ruler","Line","Area","Scatter","Skeleton","Skeletons","BoxplotChart","BoxplotChartModel","Boxplot","ZeroLine","BubbleChart","Bubble","BulletChart","BulletChartModel","Bullet","ChoroplethChart","ChoroplethModel","configOptions","ColorScaleLegend","Choropleth","CirclePackChart","CirclePackChartModel","CirclePack","graphComponentsMap","ChartTypes","StackedArea","StackedScatter","StackedRuler","SimpleBar","GroupedBar","StackedBar","ComboChart","chartOptions","comboChartTypes","counter","graphComponents","graph","type","stacked","key","merge","Component","item","flatten","stackedRulerEnabled","chartObject","PieChart","extending","PieChartModel","Pie","DonutChart","Donut","GaugeChart","GaugeChartModel","Gauge","GroupedBarChart","HeatmapChart","HeatmapModel","Heatmap","HistogramChart","ChartModelBinned","BinnedRuler","Histogram","LineChart","SkeletonLines","LollipopChart","Lollipop","MeterChart","MeterChartModel","_a","meterComponents","MeterTitle","Meter","graphFrame","RadarChart","RadarChartModel","Radar","ScatterChart","TreeChart","TreeChartModel","Tree","TreemapChart","TreemapChartModel","Treemap","SimpleBarChart","StackedAreaChart","StackedBarChart","WordCloudChart","WordCloudModel","WordCloud"],"mappings":";;;;;;;;;;;AAWA,SAASA,GAAUC,GAAOC,GAAOC,GAAK;AACpC,MAAIC,IAASH,EAAM;AACnB,SAAAE,IAAMA,MAAQ,SAAYC,IAASD,GAC3B,CAACD,KAASC,KAAOC,IAAUH,IAAQI,GAAUJ,GAAOC,GAAOC,CAAG;AACxE;ACdA,IAAIG,KAAgB,mBAChBC,KAAoB,mBACpBC,KAAwB,mBACxBC,KAAsB,mBACtBC,KAAeH,KAAoBC,KAAwBC,IAC3DE,KAAa,kBAGbC,KAAQ,WAGRC,KAAe,OAAO,MAAMD,KAAQN,KAAiBI,KAAeC,KAAa,GAAG;AASxF,SAASG,GAAWC,GAAQ;AAC1B,SAAOF,GAAa,KAAKE,CAAM;AACjC;AChBA,SAASC,GAAaD,GAAQ;AAC5B,SAAOA,EAAO,MAAM,EAAE;AACxB;ACRA,IAAIT,KAAgB,mBAChBC,KAAoB,mBACpBC,KAAwB,mBACxBC,KAAsB,mBACtBC,KAAeH,KAAoBC,KAAwBC,IAC3DE,KAAa,kBAGbM,KAAW,MAAMX,KAAgB,KACjCY,IAAU,MAAMR,KAAe,KAC/BS,IAAS,4BACTC,KAAa,QAAQF,IAAU,MAAMC,IAAS,KAC9CE,KAAc,OAAOf,KAAgB,KACrCgB,KAAa,mCACbC,KAAa,sCACbX,KAAQ,WAGRY,KAAWJ,KAAa,KACxBK,KAAW,MAAMd,KAAa,MAC9Be,KAAY,QAAQd,KAAQ,QAAQ,CAACS,IAAaC,IAAYC,EAAU,EAAE,KAAK,GAAG,IAAI,MAAME,KAAWD,KAAW,MAClHG,KAAQF,KAAWD,KAAWE,IAC9BE,KAAW,QAAQ,CAACP,KAAcH,IAAU,KAAKA,GAASI,IAAYC,IAAYN,EAAQ,EAAE,KAAK,GAAG,IAAI,KAGxGY,KAAY,OAAOV,IAAS,QAAQA,IAAS,OAAOS,KAAWD,IAAO,GAAG;AAS7E,SAASG,GAAef,GAAQ;AAC9B,SAAOA,EAAO,MAAMc,EAAS,KAAK,CAAA;AACpC;AC1BA,SAASE,GAAchB,GAAQ;AAC7B,SAAOD,GAAWC,CAAM,IACpBe,GAAef,CAAM,IACrBC,GAAaD,CAAM;AACzB;ACHA,SAASiB,GAAgBC,GAAY;AACnC,SAAO,SAASlB,GAAQ;AACtB,IAAAA,IAASmB,EAASnB,CAAM;AAExB,QAAIoB,IAAarB,GAAWC,CAAM,IAC9BgB,GAAchB,CAAM,IACpB,QAEAqB,IAAMD,IACNA,EAAW,CAAC,IACZpB,EAAO,OAAO,CAAC,GAEfsB,IAAWF,IACXnC,GAAUmC,GAAY,CAAC,EAAE,KAAK,EAAE,IAChCpB,EAAO,MAAM,CAAC;AAElB,WAAOqB,EAAIH,CAAU,EAAG,IAAGI;AAAA,EAC/B;AACA;ACXA,IAAIC,KAAaN,GAAgB,aAAa;ACD9C,SAASO,GAAWxB,GAAQ;AAC1B,SAAOuB,GAAWJ,EAASnB,CAAM,EAAE,YAAa,CAAA;AAClD;ACGA,IAAIyB,KAAYC,GAAiB,SAASC,GAAQC,GAAMC,GAAO;AAC7D,SAAAD,IAAOA,EAAK,eACLD,KAAUE,IAAQL,GAAWI,CAAI,IAAIA;AAC9C,CAAC;ACEM,MAAME,EAAM;AAAA;AAAA;AAAA;AAAA,EAelB,YAAYC,GAAwBC,GAA6C;AAdjF,SAAA,aAA0B,IACV,KAAA,WAAA;AAAA,MACf,YAAYC;AAAA,MACZ,UAAUC;AAAA,MACV,QAAQC;AAAA,MACR,OAAOC;AAAA,MACP,eAAeC;AAAA,MACf,aAAaC;AAAA,IAAA,GAEM,KAAA,QAAA,IAAIC,GAAW,KAAK,QAAQ;AAAA,EAOhD;AAAA;AAAA,EAGA,KAAKR,GAAwBC,GAA6C;AAEpE,SAAA,MAAM,IAAI,EAAE,QAAAD,KAAU,EAAE,YAAY,IAAM,GAG/C,OAAO,KAAK,KAAK,QAAQ,EAAE,QAAQ,CAAeS,MAAA;AAC3C,YAAAC,IAAa,KAAK,SAASD,CAAW;AACvC,WAAA,SAASA,CAAW,IAAI,IAAIC,EAAW,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA,CACrE,GAGD,KAAK,SAAS,OAAO,iBAAiBC,EAAY,MAAM,QAAQ,CAACC,MAAmB;AACnF,YAAMC,IAAU,CAAC,CAACC,EAAYF,GAAG,UAAU,SAAS;AACpD,WAAK,OAAOC,CAAO;AAAA,IAAA,CACnB,GAGI,KAAA,MAAM,QAAQZ,EAAa,IAAI,GAGpC,KAAK,SAAS,OAAO,iBAAiBU,EAAY,MAAM,QAAQ,MAAM;AACrE,WAAK,OAAO,EAAK;AAAA,IAAA,CACjB,GAEI,KAAA,aAAa,KAAK,iBAEvB,KAAK,OAAO;AAAA,EACb;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAA6B;AAC5B,mBAAQ,MAAM,2CAA2C,GAElD;EACR;AAAA,EAEA,OAAOE,IAAU,IAAM;AAElB,QAAA,CAAC,KAAK;AACT;AAID,WAAO,KAAK,KAAK,QAAQ,EAAE,QAAQ,CAACJ,MAAwB;AAE3D,MADmB,KAAK,SAASA,CAAW,EACjC,OAAO;AAAA,IAAA,CAClB,GAGD,KAAK,WAAW,QAAQ,CAAAM,MAAaA,EAAU,OAAOF,CAAO,CAAC;AAM9D,UAAMG,IAAqB,KAAK,SAAS,YAAY,sBAAsB,GACrEC,IAAW,OAAO,KAAKD,CAAkB,EAAE,IAAI,CAAgBE,MACjDF,EAAmBE,CAAY,EAChC,IAAI,EAAE,MAAM,CAACN,MAAWA,CAAC,CAC3C;AAEO,YAAA,IAAIK,CAAQ,EAAE;AAAA,MAAK,MAC1B,KAAK,SAAS,OAAO,cAAcN,EAAY,MAAM,eAAe;AAAA,IAAA;AAAA,EAEtE;AAAA,EAEA,UAAU;AAET,SAAK,WAAW,QAAQ,CAAaI,MAAAA,EAAU,SAAS,GAGxD,KAAK,SAAS,SAAS,UAAU,EAAE,OAAO,GAErC,KAAA,MAAM,IAAI,EAAE,WAAW,MAAQ,EAAE,YAAY,GAAA,CAAM;AAAA,EACzD;AAAA,EAEU,mBAAmBI,GAA6BC,GAAkB;AACrE,UAAAC,IAAU,KAAK,MAAM,WAAW,GAEhCC,IAAiBR,EAAYO,GAAS,WAAW,SAAS,GAE1DE,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,GAAO,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MAClD,QAAQC,EAAa;AAAA,IAAA,GAIhBC,IAAgBZ,EAAYO,GAAS,cAAc,SAAS;AAE9D,IAAAK,KAAiBA,MAAkB,MACtCP,EAAqB,KAAK,IAAIQ,GAAgB,KAAK,OAAO,KAAK,QAAQ,CAAC;AAGzE,UAAMC,IAAiB,CAAC,CAAC,KAAK,MAAM,WAAa,EAAA,OAC3CC,IAAiB;AAAA,MACtB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACjD,QAAQL,EAAa;AAAA,IAAA,GAGhBM,IAAmB;AAAA,MACxB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACnD,QAAQP,EAAa;AAAA,IAAA,GAGhBQ,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIC;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA;AAAA,YAECL;AAAA,YACA,GAAIP,IAAiB,CAACS,CAAgB,IAAI,CAAC;AAAA,UAC5C;AAAA,UACA;AAAA,YACC,WAAWI,EAAgB;AAAA,YAC3B,YAAYC,EAAiB;AAAA,UAC9B;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQX,EAAa;AAAA,IAAA,GAGhBY,IAAsB;AAAA,MAC3B,IAAI;AAAA,MACJ,YAAYlB;AAAA,MACZ,QAAQM,EAAa;AAAA,MACrB,YAAYX,EAAYM,GAAS,sBAAsB,KAAKkB,EAAY;AAAA,IAAA,GAGnEC,IACLzB,EAAYM,GAAS,eAAe,MAAM,MAAQC,EAAQ,OAAO,YAAY;AAG9E,QAAImB,IAA8BL,EAAgB;AAClD,QAAII,GAAiB;AACpB,YAAME,IAAiB3B,EAAYO,GAAS,UAAU,UAAU;AAChE,MAAIoB,MAAmB,UACtBD,IAA8BL,EAAgB,KAEzCd,EAAQ,OAAO,gBACXA,EAAA,OAAO,cAAcqB,EAAmB,aAEvCD,MAAmB,WAC7BD,IAA8BL,EAAgB,aAEzCd,EAAQ,OAAO,gBACXA,EAAA,OAAO,cAAcqB,EAAmB,aAEvCD,MAAmB,aAC7BD,IAA8BL,EAAgB;AAAA,IAEhD;AAEA,UAAMQ,IAAwB;AAAA,MAC7B,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAO,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MAClD,QAAQnB,EAAa;AAAA,IAAA,GAGhBoB,IAAqB;AAAA,MAC1B,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIX;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA,YACC,GAAIK,IAAkB,CAAChB,CAAe,IAAI,CAAC;AAAA,YAC3C,GAAIgB,IAAkB,CAACI,CAAqB,IAAI,CAAC;AAAA,YACjDN;AAAA,UACD;AAAA,UACA;AAAA,YACC,WAAWG;AAAA,UACZ;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQf,EAAa;AAAA,IAAA,GAIhBqB,IAAkC,CAAA;AAExC,QAAIlB,KAAkBN,GAAgB;AACrC,MAAAwB,EAAyB,KAAKb,CAAe;AAE7C,YAAMc,IAAuB;AAAA,QAC5B,IAAI;AAAA,QACJ,YAAY;AAAA,UACX,IAAIH,EAAO,KAAK,OAAO,KAAK,UAAUtB,IAAiB,EAAE,MAAM,GAAG,IAAI,MAAS;AAAA,QAChF;AAAA,QACA,QAAQG,EAAa;AAAA,MAAA;AAGtB,MAAAqB,EAAyB,KAAKC,CAAoB;AAAA,IACnD;AACA,WAAAD,EAAyB,KAAKD,CAAkB,GAEzC;AAAA,MACN,IAAIG,GAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,MACrC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIf,EAAgB,KAAK,OAAO,KAAK,UAAUY,GAA0B;AAAA,QACxE,WAAWX,EAAgB;AAAA,MAAA,CAC3B;AAAA,IAAA;AAAA,EAEH;AACD;ACtOO,MAAMe,UAAkBnD,EAAM;AAAA,EAQpC,YAAYC,GAAwBC,GAA6C;AAChF,UAAMD,GAAQC,CAAY,GARX,KAAA,WAAA,OAAO,OAAO,KAAK,UAAU;AAAA,MAC5C,iBAAiBkD;AAAA,MACjB,QAAQC;AAAA,MACR,MAAMC;AAAA,IAAA,CACN,GAC4B,KAAA,QAAA,IAAIC,GAAoB,KAAK,QAAQ;AAAA,EAIlE;AAAA,EAEU,uBAAuBnC,GAA6BC,GAAe;AACtE,UAAAC,IAAU,KAAK,MAAM,WAAW,GAChCkC,IAAmBzC,EAAYO,GAAS,WAAWmC,EAAc,KAAK,SAAS,GAC/ElC,IAAiBR,EAAYO,GAAS,WAAW,SAAS;AAE3D,SAAA,SAAS,gBAAgB,wBACzB,KAAA,SAAS,gBAAgB,0BACzB,KAAA,SAAS,gBAAgB;AAE9B,UAAMoC,IAAoB,KAAK,SAAS,gBAAgB,qBAAqB,GACvEC,IAAiB5C,EAAYO,GAAS,QAAQoC,GAAmB,WAAW,GAE5EE,IACLJ,KACAE,MAAsBD,EAAc,UACpCE,MAAmBE,GAAW,MAGzBC,IAAkB,KAAK,SAAS,KAAK,gBAAgBL,EAAc,GAAG,GAEtE5B,IAAiB,CAAC,CAAC,KAAK,MAAM,WAAa,EAAA,OAC3CC,IAAiB;AAAA,MACtB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACjD,QAAQL,EAAa;AAAA,IAAA,GAGhBM,IAAmB;AAAA,MACxB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACnD,QAAQP,EAAa;AAAA,IAAA,GAGhBQ,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIC;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA;AAAA,YAECL;AAAA,YACA,GAAIP,IAAiB,CAACS,CAAgB,IAAI,CAAC;AAAA,UAC5C;AAAA,UACA;AAAA,YACC,WAAWI,EAAgB;AAAA,YAC3B,YAAYC,EAAiB;AAAA,UAC9B;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQX,EAAa;AAAA,IAAA,GAGhBF,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,GAAO,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MAClD,QAAQC,EAAa;AAAA,IAAA;AAIlB,IAAAkC,KAAkB,CAACE,KACD1C,EAAA;AAAA,MACpB,IAAI2C,GAAU,KAAK,OAAO,KAAK,QAAQ;AAAA,MACvC,IAAIC,GAAW,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA,GAI1C5C,EAAqB,KAAK,IAAI6C,GAAU,KAAK,OAAO,KAAK,QAAQ,CAAC,GAClE7C,EAAqB,KAAK,IAAI8C,GAAU,KAAK,OAAO,KAAK,QAAQ,CAAC;AAElE,UAAM5B,IAAsB;AAAA,MAC3B,IAAI;AAAA,MACJ,YAAYlB;AAAA,MACZ,QAAQM,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBC,IACLzB,EAAYM,GAAS,UAAU,SAAS,MAAM,MAC9C,KAAK,MAAM,WAAA,EAAa,OAAO,YAAY;AAG5C,QAAIoB,IAA8BL,EAAgB;AAClD,QAAII,GAAiB;AACpB,YAAME,IAAiB3B,EAAY,KAAK,MAAM,cAAc,UAAU,UAAU;AAC5E,MAAA2B,MAAmByB,EAAgB,QACtC1B,IAA8BL,EAAgB,KAEzC,KAAK,MAAM,WAAW,EAAE,OAAO,gBACnC,KAAK,MAAM,WAAa,EAAA,OAAO,cAAcO,EAAmB,aAEvDD,MAAmByB,EAAgB,SAC7C1B,IAA8BL,EAAgB,aAEzC,KAAK,MAAM,WAAW,EAAE,OAAO,gBACnC,KAAK,MAAM,WAAa,EAAA,OAAO,cAAcO,EAAmB,aAEvDD,MAAmByB,EAAgB,WAC7C1B,IAA8BL,EAAgB;AAAA,IAEhD;AAEA,UAAMQ,KAAwB;AAAA,MAC7B,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAO,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MAClD,QAAQnB,EAAa;AAAA,IAAA,GAGhBoB,KAAqB;AAAA,MAC1B,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIX;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA,YACC,GAAIK,IAAkB,CAAChB,CAAe,IAAI,CAAC;AAAA,YAC3C,GAAIgB,IAAkB,CAACI,EAAqB,IAAI,CAAC;AAAA,YACjDN;AAAA,UACD;AAAA,UACA;AAAA,YACC,WAAWG;AAAA,UACZ;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQf,EAAa;AAAA,IAAA,GAGhB0C,KAAmB;AAAA,MACxB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,GAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACnD,QAAQ3C,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBQ,IAA2B,CAAA;AAEjC,QAAIlB,KAAkBN,GAAgB;AACrC,MAAAwB,EAAyB,KAAKb,CAAe;AAE7C,YAAMc,IAAuB;AAAA,QAC5B,IAAI;AAAA,QACJ,YAAY;AAAA,UACX,IAAIH,EAAO,KAAK,OAAO,KAAK,UAAUtB,IAAiB,EAAE,MAAM,GAAG,IAAI,MAAS;AAAA,QAChF;AAAA,QACA,QAAQG,EAAa;AAAA,MAAA;AAGtB,MAAAqB,EAAyB,KAAKC,CAAoB;AAAA,IACnD;AACA,WAAIY,KACHb,EAAyB,KAAKqB,EAAgB,GAE/CrB,EAAyB,KAAKD,EAAkB,GAEzC;AAAA,MACN,IAAIwB,GAAkB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAC/C,IAAIpB,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIf,EAAgB,KAAK,OAAO,KAAK,UAAUY,GAA0B;AAAA,QACxE,WAAWX,EAAgB;AAAA,MAAA,CAC3B;AAAA,IAAA;AAAA,EAEH;AACD;ACpMO,MAAMmC,WAAsBvE,EAAM;AAAA,EAGxC,YAAYC,GAAwBC,GAAiD;AACpF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIsE,GAAmB,KAAK,QAAQ,GAO3C,KAAK,MAAM,WAAWC,EAAyBnD,EAAQ,eAAepB,EAAa,OAAO,CAAC,GAGtF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAIsD,GAAS,KAAK,OAAO,KAAK,QAAQ,CAAC;AAK3E,WAHyB,KAAK,mBAAmBtD,GAAsB;AAAA,MAC7E,eAAe;AAAA,IAAA,CACf;AAAA,EAEF;AACD;ACpBO,MAAMuD,WAAkBxB,EAAU;AAAA,EACxC,YAAYlD,GAAwBC,GAA6C;AAChF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM;AAAA,MACVuE,EAAyBG,EAAUtD,EAAQ,SAAS,GAAGpB,EAAa,OAAO;AAAA,IAAA,GAIvE,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,GAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAQ,KAAK,OAAO,KAAK,UAAU;AAAA,QACtC,8BAA8B;AAAA,QAC9B,kBAAkB;AAAA,MAAA,CAClB;AAAA,MACD,IAAIC,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;ACxCO,MAAMiE,WAAqBlC,EAAU;AAAA,EAG3C,YAAYlD,GAAwBC,GAAgD;AACnF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIoF,GAAkB,KAAK,QAAQ,GAO1C,KAAK,MAAM,WAAWb,EAAyBnD,EAAQ,cAAcpB,EAAa,OAAO,CAAC,GAGrF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIS,GAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,MACrC,IAAIC,EAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,MACtC,IAAIL,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAQK,WALyB,KAAK,uBAAuBhE,GAAsB;AAAA,MACjF,QAAQ;AAAA,QACP,SAAS;AAAA,MACV;AAAA,IAAA,CACA;AAAA,EAEF;AACD;ACtCO,MAAMqE,WAAoBtC,EAAU;AAAA,EAC1C,YAAYlD,GAAwBC,GAA+C;AAClF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,aAAapB,EAAa,OAAO,CAAC,GAGpF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIW,GAAO,KAAK,OAAO,KAAK,QAAQ;AAAA,MACpC,IAAIP,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;AC/BO,MAAMuE,WAAoBxC,EAAU;AAAA,EAG1C,YAAYlD,GAAwBC,GAA+C;AAClF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAI0F,GAAiB,KAAK,QAAQ,GAOzC,KAAK,MAAM,WAAWnB,EAAyBnD,EAAQ,aAAapB,EAAa,OAAO,CAAC,GAGpF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIe,GAAO,KAAK,OAAO,KAAK,QAAQ;AAAA,MACpC,IAAIV,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;AC5BO,MAAM0E,WAAwB9F,EAAM;AAAA,EAG1C,YAAYC,GAAwBC,GAAmD;AACtF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAI6F,GAAgB,KAAK,QAAQ,GAOxC,KAAK,MAAM;AAAA,MACVtB,EAAyBuB,EAAc,iBAAiB9F,EAAa,OAAO;AAAA,IAAA,GAIxE,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA,EAIU,mBAAmBkB,GAA6BC,GAAe;AAClE,UAAAC,IAAU,KAAK,MAAM,WAAW,GAChCC,IAAiBR,EAAYO,GAAS,WAAW,SAAS,GAE1DO,IAAiB,CAAC,CAAC,KAAK,MAAM,WAAa,EAAA,OAC3CC,IAAiB;AAAA,MACtB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACjD,QAAQL,EAAa;AAAA,IAAA,GAGhBM,IAAmB;AAAA,MACxB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACnD,QAAQP,EAAa;AAAA,IAAA,GAGhBQ,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIC;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA;AAAA,YAECL;AAAA,YACA,GAAIP,IAAiB,CAACS,CAAgB,IAAI,CAAC;AAAA,UAC5C;AAAA,UACA;AAAA,YACC,WAAWI,EAAgB;AAAA,YAC3B,YAAYC,EAAiB;AAAA,UAC9B;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQX,EAAa;AAAA,IAAA,GAGhBF,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIyE,GAAiB,KAAK,OAAO,KAAK,UAAU;AAAA,UAC/C,WAAW;AAAA,QAAA,CACX;AAAA,MACF;AAAA,MACA,QAAQvE,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBD,IAAsB;AAAA,MAC3B,IAAI;AAAA,MACJ,YAAYlB;AAAA,MACZ,QAAQM,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBC,IACLzB,EAAYM,GAAS,UAAU,SAAS,MAAM,MAC9C,KAAK,MAAM,WAAW,EAAE,OAAO,YAAY,MAC3C,KAAK,MAAM,QAAA,EAAU,SAAS,GAGzBoB,IAA8BL,EAAgB,gBAE9CQ,IAAwB;AAAA,MAC7B,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAO,KAAK,OAAO,KAAK,UAAU,EAAE,MAAM,GAAG,CAAC,CAAC;AAAA,MAChE,QAAQnB,EAAa;AAAA,IAAA,GAGhBoB,IAAqB;AAAA,MAC1B,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIX;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA,YACC,GAAIK,IAAkB,CAAChB,CAAe,IAAI,CAAC;AAAA,YAC3C,GAAIgB,IAAkB,CAACI,CAAqB,IAAI,CAAC;AAAA,YACjDN;AAAA,UACD;AAAA,UACA;AAAA,YACC,WAAWG;AAAA,UACZ;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQf,EAAa;AAAA,IAAA,GAGhBqB,IAA2B,CAAA;AAEjC,QAAIlB,KAAkBN,GAAgB;AACrC,MAAAwB,EAAyB,KAAKb,CAAe;AAE7C,YAAMc,IAAuB;AAAA,QAC5B,IAAI;AAAA,QACJ,YAAY;AAAA,UACX,IAAIH,EAAO,KAAK,OAAO,KAAK,UAAUtB,IAAiB,EAAE,MAAM,GAAG,IAAI,MAAS;AAAA,QAChF;AAAA,QACA,QAAQG,EAAa;AAAA,MAAA;AAGtB,MAAAqB,EAAyB,KAAKC,CAAoB;AAAA,IACnD;AACA,WAAAD,EAAyB,KAAKD,CAAkB,GAEzC;AAAA,MACN,IAAIG,GAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,MACrC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIf,EAAgB,KAAK,OAAO,KAAK,UAAUY,GAA0B;AAAA,QACxE,WAAWX,EAAgB;AAAA,MAAA,CAC3B;AAAA,IAAA;AAAA,EAEH;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAhB,IAAoC,CAAC,IAAI8E,GAAW,KAAK,OAAO,KAAK,QAAQ,CAAC;AAG7E,WADyB,KAAK,mBAAmB9E,CAAoB;AAAA,EAE7E;AACD;ACvJO,MAAM+E,WAAwBnG,EAAM;AAAA,EAG1C,YAAYC,GAAwBC,GAAmD;AACtF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIkG,GAAqB,KAAK,QAAQ,GAO7C,KAAK,MAAM,WAAW3B,EAAyBnD,EAAQ,iBAAiBpB,EAAa,OAAO,CAAC,GAGxF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAIiF,GAAW,KAAK,OAAO,KAAK,QAAQ,CAAC;AAI7E,WADyB,KAAK,mBAAmBjF,CAAoB;AAAA,EAE7E;AACD;ACZA,MAAMkF,IAAqB;AAAA,EAC1B,CAACC,EAAW,IAAI,GAAG,CAACvB,GAAME,CAAO;AAAA,EACjC,CAACqB,EAAW,OAAO,GAAG,CAACrB,CAAO;AAAA,EAC9B,CAACqB,EAAW,IAAI,GAAG,CAACtB,IAAMD,GAAME,CAAO;AAAA,EACvC,CAACqB,EAAW,YAAY,GAAG,CAACC,IAAaxB,GAAMyB,IAAgBC,CAAY;AAAA,EAC3E,CAACH,EAAW,UAAU,GAAG,CAACI,EAAS;AAAA,EACnC,CAACJ,EAAW,WAAW,GAAG,CAACK,IAAYpB,CAAQ;AAAA,EAC/C,CAACe,EAAW,WAAW,GAAG,CAACM,IAAYH,CAAY;AACpD;AAEO,MAAMI,WAAmB3D,EAAU;AAAA,EACzC,YAAYlD,GAAwBC,GAA8C;AACjF,UAAMD,GAAQC,CAAY;AAI1B,UAAM6G,IAAetC,EAAyBuB,EAAc,YAAY9F,EAAa,OAAO;AAIxF,IAACA,EAAa,QAAQ,oBACzB,QAAQ,MAAM,iDAAiD,GAElD6G,EAAA,kBAAkB,CAAC,EAAE,MAAMR,EAAW,MAAM,uBAAuB,CAAC,EAAA,CAAG,IAIhF,KAAA,MAAM,WAAWQ,CAAY,GAG7B,KAAA,KAAK9G,GAAQC,CAAY;AAAA,EAC/B;AAAA,EAEA,qBAAqB;AACpB,UAAM,EAAE,iBAAA8G,EAAsD,IAAA,KAAK,MAAM,WAAW;AACpF,QAAIC,IAAU;AACd,UAAMC,IAA+BF,EACnC,IAAI,CAACG,MAAe;AACpB,YAAMC,IAAOD,EAAM;AACf,UAAA7F;AAGA,UAAA,OAAO6F,EAAM,QAAS,UAAU;AAG/B,YAAA,CAAC,OAAO,KAAKb,CAAkB,EAAE,SAASa,EAAM,IAAI;AAC/C,yBAAA;AAAA,YACP,uBAAuBA,EAAM,IAAI;AAAA,UAAA,GAE3B;AAER,YAAIE,IAAU;AACd,cAAMC,IAAM,GAAG3H,GAAUwH,EAAM,IAAI,CAAC;AAC1B7F,eAAAA,IAAAiG,EAAM,IAAIvB,EAAcsB,CAAG,GAAG,KAAK,MAAM,WAAA,GAAcH,EAAM,OAAO,GAE1EA,EAAM,SAASZ,EAAW,iBACnBc,IAAA,KAEJf,EAAmBa,EAAM,IAAuC,EAAE;AAAA,UACxE,CAACK,MACA,IAAIA,EAAU,KAAK,OAAO,KAAK,UAAU;AAAA,YACxC,QAAQL,EAAM;AAAA,YACd,IAAIF;AAAA,YAAA,SACJ3F;AAAAA,YACA,SAAA+F;AAAA,UAAA,CACA;AAAA,QAAA;AAAA,MACH;AAGU/F,eAAAA,IAAAiG,EAAM,CAAA,GAAI,KAAK,MAAM,WAAW,GAAGJ,EAAM,OAAO,GACnD,IAAIC,EAAK,KAAK,OAAO,KAAK,UAAU;AAAA,UAC1C,QAAQD,EAAM;AAAA,UACd,IAAIF;AAAA,UAAA,SACJ3F;AAAAA,QAAA,CACA;AAAA,IAEF,CAAA,EACA,OAAO,CAACmG,MAAcA,MAAS,IAAI;AAErC,WAAOC,GAAQR,CAAe;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAM,EAAE,iBAAAF,EAAoB,IAAA,KAAK,MAAM,WAAW,GAE5CW,IAAsBX,EAAgB;AAAA,MAC3C,CAACY,MACAA,EAAY,SAASrB,EAAW,eAAeqB,EAAY,SAASrB,EAAW;AAAA,IAAA,GAI3EnF,IAAuB;AAAA,MAC5B,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIK,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,MACD,GAAIuC,IAAsB,CAAK,IAAA,CAAC,IAAI5C,EAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACpE,GAAG,KAAK,mBAAmB;AAAA,IAAA;AAKrB,WAFyB,KAAK,uBAAuB3D,CAAoB;AAAA,EAGjF;AACD;AC1HO,MAAMyG,WAAiB7H,EAAM;AAAA;AAAA,EAInC,YACCC,GACAC,GACA4H,IAAY,IACX;AAID,IAHA,MAAM7H,GAAQC,CAAY,GARnB,KAAA,QAAA,IAAI6H,GAAc,KAAK,QAAQ,GAWlC,CAAAD,MAMJ,KAAK,MAAM,WAAWrD,EAAyBnD,EAAQ,UAAUpB,EAAa,OAAO,CAAC,GAGjF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAI4G,GAAI,KAAK,OAAO,KAAK,QAAQ;AAAA,MACjC,IAAI7C,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAKK,WADyB,KAAK,mBAAmBhE,CAAoB;AAAA,EAE7E;AACD;AC1CO,MAAM6G,WAAmBJ,GAAS;AAAA,EACxC,YAAY5H,GAAwBC,GAA4C;AACzE,UAAAD,GAAQC,GAAc,EAAI,GAIhC,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,YAAYpB,EAAa,OAAO,CAAC,GAGnF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAI8G,GAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAI/C,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,mBAAmBhE,CAAoB;AAAA,EAE7E;AACD;AC7BO,MAAM+G,WAAmBnI,EAAM;AAAA,EAErC,YAAYC,GAAwBC,GAA8C;AACjF,UAAMD,GAAQC,CAAY,GAFnB,KAAA,QAAA,IAAIkI,GAAgB,KAAK,QAAQ,GAMxC,KAAK,MAAM,WAAW3D,EAAyBnD,EAAQ,YAAYpB,EAAa,OAAO,CAAC,GAGnF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAIiH,GAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAIxE,WAFyB,KAAK,mBAAmBjH,CAAoB;AAAA,EAG7E;AACD;ACrBO,MAAMkH,WAAwBnF,EAAU;AAAA,EAC9C,YAAYlD,GAAwBC,GAA4C;AAC/E,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,iBAAiBpB,EAAa,OAAO,CAAC,GAGxF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAI8B,GAAW,KAAK,OAAO,KAAK,QAAQ;AAAA,MACxC,IAAIpB,EAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,MACtC,IAAIL,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;AC1BO,MAAMmH,WAAqBpF,EAAU;AAAA,EAG3C,YAAYlD,GAAwBC,GAAgD;AACnF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIsI,GAAa,KAAK,QAAQ,GAOrC,KAAK,MAAM;AAAA,MACV/D,EAAyBuB,EAAc,cAAc9F,EAAa,OAAO;AAAA,IAAA,GAIrE,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA,EAIU,uBAAuBkB,GAA6BC,GAAe;AACtE,UAAAC,IAAU,KAAK,MAAM,WAAW,GAChCC,IAAiBR,EAAYO,GAAS,WAAW,SAAS;AAE3D,SAAA,SAAS,gBAAgB,wBACzB,KAAA,SAAS,gBAAgB,0BACzB,KAAA,SAAS,gBAAgB;AAE9B,UAAMO,IAAiB,CAAC,CAAC,KAAK,MAAM,WAAa,EAAA,OAC3CC,IAAiB;AAAA,MACtB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACjD,QAAQL,EAAa;AAAA,IAAA,GAGhBM,IAAmB;AAAA,MACxB,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,MACnD,QAAQP,EAAa;AAAA,IAAA,GAGhBQ,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIC;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA;AAAA,YAECL;AAAA,YACA,GAAIP,IAAiB,CAACS,CAAgB,IAAI,CAAC;AAAA,UAC5C;AAAA,UACA;AAAA,YACC,WAAWI,EAAgB;AAAA,YAC3B,YAAYC,EAAiB;AAAA,UAC9B;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQX,EAAa;AAAA,IAAA,GAGhBF,IAAkB;AAAA,MACvB,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIyE,GAAiB,KAAK,OAAO,KAAK,UAAU;AAAA,UAC/C,WAAW;AAAA,QAAA,CACX;AAAA,MACF;AAAA,MACA,QAAQvE,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBD,IAAsB;AAAA,MAC3B,IAAI;AAAA,MACJ,YAAYlB;AAAA,MACZ,QAAQM,EAAa;AAAA,MACrB,YAAYa,EAAY;AAAA,IAAA,GAGnBC,IACLzB,EAAYM,GAAS,UAAU,SAAS,MAAM,MAC9C,KAAK,MAAM,WAAW,EAAE,OAAO,YAAY,MAC3C,KAAK,MAAM,QAAA,EAAU,SAAS,GAGzBoB,IAA8BL,EAAgB,gBAE9CQ,IAAwB;AAAA,MAC7B,IAAI;AAAA,MACJ,YAAY,CAAC,IAAIC,EAAO,KAAK,OAAO,KAAK,UAAU,EAAE,MAAM,GAAG,CAAC,CAAC;AAAA,MAChE,QAAQnB,EAAa;AAAA,IAAA,GAGhBoB,IAAqB;AAAA,MAC1B,IAAI;AAAA,MACJ,YAAY;AAAA,QACX,IAAIX;AAAA,UACH,KAAK;AAAA,UACL,KAAK;AAAA,UACL;AAAA,YACC,GAAIK,IAAkB,CAAChB,CAAe,IAAI,CAAC;AAAA,YAC3C,GAAIgB,IAAkB,CAACI,CAAqB,IAAI,CAAC;AAAA,YACjDN;AAAA,UACD;AAAA,UACA;AAAA,YACC,WAAWG;AAAA,UACZ;AAAA,QACD;AAAA,MACD;AAAA,MACA,QAAQf,EAAa;AAAA,IAAA,GAGhBqB,IAAkC,CAAA;AAExC,QAAIlB,KAAkBN,GAAgB;AACrC,MAAAwB,EAAyB,KAAKb,CAAe;AAE7C,YAAMc,IAAuB;AAAA,QAC5B,IAAI;AAAA,QACJ,YAAY;AAAA,UACX,IAAIH,EAAO,KAAK,OAAO,KAAK,UAAUtB,IAAiB,EAAE,MAAM,GAAG,IAAI,MAAS;AAAA,QAChF;AAAA,QACA,QAAQG,EAAa;AAAA,MAAA;AAGtB,MAAAqB,EAAyB,KAAKC,CAAoB;AAAA,IACnD;AACA,WAAAD,EAAyB,KAAKD,CAAkB,GAEzC;AAAA,MACN,IAAIwB,GAAkB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAC/C,IAAIpB,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIf,EAAgB,KAAK,OAAO,KAAK,UAAUY,GAA0B;AAAA,QACxE,WAAWX,EAAgB;AAAA,MAAA,CAC3B;AAAA,IAAA;AAAA,EAEH;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMhB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAI4D,GAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA;AAI/B,WADyB,KAAK,uBAAuBrH,CAAoB;AAAA,EAEjF;AACD;AC5JO,MAAMsH,WAAuBvF,EAAU;AAAA,EAG7C,YAAYlD,GAAwBC,GAAkD;AACrF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIyI,GAAiB,KAAK,QAAQ,GAOzC,KAAK,MAAM,WAAWlE,EAAyBnD,EAAQ,gBAAgBpB,EAAa,OAAO,CAAC,GAGvF,KAAA,KAAKD,GAAQC,CAAY,GAE9B,KAAK,OAAO;AAAA,EACb;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAI8D,GAAY,KAAK,OAAO,KAAK,QAAQ;AAAA,MACzC,IAAIC,GAAU,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA;AAKjC,WAFyB,KAAK,uBAAuBzH,CAAoB;AAAA,EAGjF;AACD;AC/BO,MAAM0H,WAAkB3F,EAAU;AAAA,EACxC,YAAYlD,GAAwBC,GAA6C;AAChF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,WAAWpB,EAAa,OAAO,CAAC,GAGlF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIE,EAAQ,KAAK,OAAO,KAAK,UAAU,EAAE,kBAAkB,IAAM;AAAA,MACjE,IAAI6D,GAAc,KAAK,OAAO,KAAK,QAAQ;AAAA,MAC3C,IAAIvD,EAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA;AAIhC,WADyB,KAAK,uBAAuBpE,CAAoB;AAAA,EAEjF;AACD;AC/BO,MAAM4H,WAAsB7F,EAAU;AAAA,EAC5C,YAAYlD,GAAwBC,GAAiD;AACpF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,eAAepB,EAAa,OAAO,CAAC,GAGtF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIkE,GAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,MACtC,IAAI/D,EAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,MACrC,IAAIC,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;AChCO,MAAM8H,WAAmBlJ,EAAM;AAAA,EAGrC,YAAYC,GAAwBC,GAA8C;;AACjF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIiJ,GAAgB,KAAK,QAAQ;AAMxC,UAAM7H,KAAU8H,IAAAlJ,EAAa,QAAQ,UAArB,QAAAkJ,EAA4B,eACzC7B,EAAM3C,EAAUoB,EAAc,sBAAsB,GAAG9F,EAAa,OAAO,IAC3EqH,EAAM3C,EAAUoB,EAAc,UAAU,GAAG9F,EAAa,OAAO;AAI7D,SAAA,MAAM,WAAWoB,CAAO,GAGxB,KAAA,KAAKrB,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AAEf,UAAMmJ,IAAkB;AAAA,MACvB,GAFkBtI,EAAY,KAAK,MAAM,cAAc,SAAS,YAAY,IAGzE;AAAA;AAAA,QAEA;AAAA,UACC,IAAI;AAAA,UACJ,YAAY,CAAC,IAAIuI,GAAW,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,UACtD,QAAQ5H,EAAa;AAAA,UACrB,YAAYa,EAAY;AAAA,QACzB;AAAA;AAAA,QAEA;AAAA,UACC,IAAI;AAAA,UACJ,YAAY;AAAA,YACX,IAAIM,EAAO,KAAK,OAAO,KAAK,UAAU;AAAA,cACrC,MAAM;AAAA,YAAA,CACN;AAAA,UACF;AAAA,UACA,QAAQnB,EAAa;AAAA,QACtB;AAAA,MAAA,IAEA,CAAC;AAAA;AAAA,MAEJ;AAAA,QACC,IAAI;AAAA,QACJ,YAAY,CAAC,IAAI6H,GAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAAA,QACjD,QAAQ7H,EAAa;AAAA,QACrB,YAAYa,EAAY;AAAA,MACzB;AAAA,IAAA,GAIKiH,IAA0B;AAAA,MAC/B,IAAIrH,EAAgB,KAAK,OAAO,KAAK,UAAUkH,GAAiB;AAAA,QAC/D,WAAWjH,EAAgB;AAAA,MAAA,CAC3B;AAAA,IAAA;AAQK,WAJyB,KAAK,mBAAmBoH,GAAY;AAAA,MACnE,sBAAsBjH,EAAY;AAAA,IAAA,CAClC;AAAA,EAGF;AACD;AC5EO,MAAMkH,WAAmBzJ,EAAM;AAAA,EAGrC,YAAYC,GAAwBC,GAA8C;AACjF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAIwJ,GAAgB,KAAK,QAAQ,GAOxC,KAAK,MAAM,WAAWjF,EAAyBnD,EAAQ,YAAYpB,EAAa,OAAO,CAAC,GAGnF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAIuI,GAAM,KAAK,OAAO,KAAK,QAAQ,CAAC;AAIxE,WADyB,KAAK,mBAAmBvI,CAAoB;AAAA,EAE7E;AACD;ACtBO,MAAMwI,WAAqBzG,EAAU;AAAA,EAC3C,YAAYlD,GAAwBC,GAAgD;AACnF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,cAAcpB,EAAa,OAAO,CAAC,GAGrF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAIC,EAAM,KAAK,OAAO,KAAK,QAAQ;AAAA,MACnC,IAAIG,EAAQ,KAAK,OAAO,KAAK,QAAQ;AAAA,MACrC,IAAIC,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;ACnCO,MAAMyI,WAAkB7J,EAAM;AAAA,EAGpC,YAAYC,GAAwBC,GAA6C;AAChF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAI4J,GAAe,KAAK,QAAQ,GAOvC,KAAK,MAAM,WAAWrF,EAAyBnD,EAAQ,WAAWpB,EAAa,OAAO,CAAC,GAGlF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAI2I,GAAK,KAAK,OAAO,KAAK,QAAQ,CAAC;AAMvE,WAHyB,KAAK,mBAAmB3I,GAAsB;AAAA,MAC7E,eAAe;AAAA,IAAA,CACf;AAAA,EAEF;AACD;AC5BO,MAAM4I,WAAqBhK,EAAM;AAAA,EAGvC,YAAYC,GAAwBC,GAAgD;AACnF,UAAMD,GAAQC,CAAY,GAHnB,KAAA,QAAA,IAAI+J,GAAkB,KAAK,QAAQ,GAO1C,KAAK,MAAM,WAAWxF,EAAyBnD,EAAQ,cAAcpB,EAAa,OAAO,CAAC,GAGrF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACT,UAAAkB,IAAoC,CAAC,IAAI8I,GAAQ,KAAK,OAAO,KAAK,QAAQ,CAAC;AAI1E,WADyB,KAAK,mBAAmB9I,CAAoB;AAAA,EAE7E;AACD;ACtBO,MAAM+I,WAAuBhH,EAAU;AAAA,EAC7C,YAAYlD,GAAwBC,GAA4C;AAC/E,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,gBAAgBpB,EAAa,OAAO,CAAC,GAGvF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAI6B,GAAU,KAAK,OAAO,KAAK,QAAQ;AAAA,MACvC,IAAInB,EAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,MACtC,IAAIL,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;AC7BO,MAAMgJ,WAAyBjH,EAAU;AAAA,EAC/C,YAAYlD,GAAwBC,GAA6C;AAChF,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,kBAAkBpB,EAAa,OAAO,CAAC,GAGzF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAI4B,EAAa,KAAK,OAAO,KAAK,QAAQ;AAAA,MAC1C,IAAIF,GAAY,KAAK,OAAO,KAAK,QAAQ;AAAA,MACzC,IAAIxB,EAAK,KAAK,OAAO,KAAK,UAAU,EAAE,SAAS,IAAM;AAAA,MACrD,IAAIyB,GAAe,KAAK,OAAO,KAAK,UAAU;AAAA,QAC7C,8BAA8B;AAAA,QAC9B,kBAAkB;AAAA,QAClB,SAAS;AAAA,MAAA,CACT;AAAA,MACD,IAAItB,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAIK,WADyB,KAAK,uBAAuBhE,CAAoB;AAAA,EAEjF;AACD;ACtCO,MAAMiJ,WAAwBlH,EAAU;AAAA,EAC9C,YAAYlD,GAAwBC,GAA4C;AAC/E,UAAMD,GAAQC,CAAY,GAI1B,KAAK,MAAM,WAAWuE,EAAyBnD,EAAQ,iBAAiBpB,EAAa,OAAO,CAAC,GAGxF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIyD,EAAmB,KAAK,OAAO,KAAK,QAAQ;AAAA,MAChD,IAAIC,EAAK,KAAK,OAAO,KAAK,QAAQ;AAAA,MAClC,IAAI4B,EAAa,KAAK,OAAO,KAAK,QAAQ;AAAA,MAC1C,IAAIG,GAAW,KAAK,OAAO,KAAK,QAAQ;AAAA,MACxC,IAAI1B,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,MACD,IAAII,EAAS,KAAK,OAAO,KAAK,QAAQ;AAAA,IAAA;AAIhC,WADyB,KAAK,uBAAuBpE,CAAoB;AAAA,EAEjF;AACD;AClCO,MAAMkJ,WAAuBtK,EAAM;AAAA,EAGzC,YACCC,GACAC,GACC;AACD,UAAMD,GAAQC,CAAY,GANnB,KAAA,QAAA,IAAIqK,GAAe,KAAK,QAAQ,GAUvC,KAAK,MAAM,WAAW9F,EAAyBnD,EAAQ,gBAAgBpB,EAAa,OAAO,CAAC,GAGvF,KAAA,KAAKD,GAAQC,CAAY;AAAA,EAC/B;AAAA;AAAA;AAAA;AAAA;AAAA;AAAA,EAOA,gBAAgB;AACf,UAAMkB,IAAoC;AAAA,MACzC,IAAIoJ,GAAU,KAAK,OAAO,KAAK,QAAQ;AAAA,MACvC,IAAIrF,EAAS,KAAK,OAAO,KAAK,UAAU;AAAA,QACvC,UAAUC,EAAU;AAAA,MAAA,CACpB;AAAA,IAAA;AAKK,WADyB,KAAK,mBAAmBhE,CAAoB;AAAA,EAE7E;AACD;","x_google_ignoreList":[0,1,2,3,4,5,6,7,8]}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy