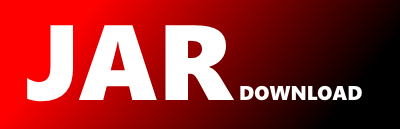
package.dist.model.model.d.ts Maven / Gradle / Ivy
import { ColorClassNameTypes } from '../interfaces/enums';
import { ChartTabularData } from '../interfaces/model';
export type StackKeysParams = {
bins?: any;
groups?: any;
percentage?: any;
divergent?: any;
};
/** The charting model layer which includes mainly the chart data and options,
* as well as some misc. information to be shared among components */
export declare class ChartModel {
protected services: any;
protected state: any;
/**
* A list of all the data groups that have existed within the lifetime of the chart
* @type string[]
*/
protected allDataGroups: string[];
protected colorScale: any;
protected colorClassNames: any;
constructor(services: any);
formatTable({ headers, cells }: {
headers: any;
cells: any;
}): any[];
getAllDataFromDomain(groups?: any): any;
/**
* Charts that have group configs passed into them, only want to retrieve the display data relevant to that chart
* @param groups the included datasets for the particular chart
*/
getDisplayData(groups?: any): any;
getData(): any;
isDataEmpty(): boolean;
/**
* Sets the data for the current instance.
*
* This method sanitizes the provided data, generates data groups,
* and updates the instance's state with the sanitized data and data groups.
*
* @param {any} newData - The new data to be set. This data will be cloned and sanitized.
* @returns {any} - The sanitized version of the provided data.
*/
setData(newData: any): any;
getDataGroups(groups?: any): any;
getActiveDataGroups(groups?: any): any;
getDataGroupNames(groups?: any): any;
getActiveDataGroupNames(groups?: any): any;
private aggregateBinDataByGroup;
getBinConfigurations(): {
bins: import('d3-array').Bin[];
binsDomain: any[];
};
getBinnedStackedData(): import('d3-shape').SeriesPoint<{
[key: string]: number;
}>[][];
getGroupedData(groups?: any): {
name: string;
data: any;
}[];
getStackKeys({ bins, groups }?: StackKeysParams): any;
getDataValuesGroupedByKeys({ bins, groups }: StackKeysParams): any;
getStackedData({ percentage, groups, divergent }: StackKeysParams): any[][];
/**
* Retrieves the current options from the instance's state.
*
* @returns {any} - The current options stored in the instance's state.
*/
getOptions(): any;
set(newState: any, configs?: any): void;
get(property?: string): any;
/**
* Updates the current options for the instance.
*
* This method retrieves the existing options, updates the legend additional items,
* and merges the new options with the existing ones. The instance's state is then updated
* with the merged options.
*
* @param {any} newOptions - The new options to be set. These options will be merged with the existing options.
*/
setOptions(newOptions: any): void;
/**
*
* Updates miscellanous information within the model
* such as the color scales, or the legend data labels
*/
update(animate?: boolean): void;
toggleDataLabel(changedLabel: string): void;
/**
* Should the data point be filled?
* @param group
* @param key
* @param data
* @param defaultFilled the default for this chart
*/
getIsFilled(group: any, key?: any, data?: any, defaultFilled?: boolean): any;
getFillColor(group: any, key?: any, data?: any): any;
getStrokeColor(group: any, key?: any, data?: any): any;
isUserProvidedColorScaleValid(): any;
getColorClassName(configs: {
classNameTypes?: ColorClassNameTypes[];
dataGroupName?: string | number;
originalClassName?: string;
value?: number;
}): string;
/**
* For charts that might hold an associated status for their dataset
*/
getStatus(): any;
getAllDataGroupsNames(): string[];
/**
* Converts data provided in the older format to tabular
*
*/
protected transformToTabularData(data: any): ChartTabularData;
getTabularDataArray(): ChartTabularData;
exportToCSV(): void;
protected getTabularData(data: any): any[];
protected sanitize(data: any): any;
protected updateAllDataGroups(): void;
protected generateDataGroups(data: any): {
name: unknown;
status: number;
}[];
protected setCustomColorScale(): void;
protected setColorClassNames(): void;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy