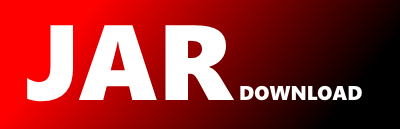
package.dist.services.scales-cartesian.d.ts Maven / Gradle / Ivy
import { ScaleTime, ScaleBand, ScaleLinear } from 'd3';
import { Service } from './service';
import { AxisPositions, CartesianOrientations, ScaleTypes } from '../interfaces/enums';
import { ThresholdOptions } from '../interfaces/components';
export type ScaleFunction = ScaleTime | ScaleBand | ScaleLinear;
export declare class CartesianScales extends Service {
protected scaleTypes: {
top: ScaleTypes;
right: ScaleTypes;
bottom: ScaleTypes;
left: ScaleTypes;
};
protected scales: {
top: ScaleLinear;
right: ScaleLinear;
bottom: ScaleLinear;
left: ScaleLinear;
};
protected domainAxisPosition: AxisPositions;
protected rangeAxisPosition: AxisPositions;
protected secondaryDomainAxisPosition: AxisPositions;
protected secondaryRangeAxisPosition: AxisPositions;
protected dualAxes: boolean;
protected orientation: CartesianOrientations;
getDomainAxisPosition({ datum }?: {
datum?: any;
}): AxisPositions;
getRangeAxisPosition({ datum, groups }?: {
datum?: any;
groups?: any;
}): AxisPositions;
getAxisOptions(position: AxisPositions): any;
getDomainAxisOptions(): any;
getRangeAxisOptions(): any;
getScaleLabel(position: AxisPositions): string;
getDomainLabel(): string;
getRangeLabel(): string;
update(): void;
findDomainAndRangeAxes(): void;
determineOrientation(): void;
isDualAxes(): boolean;
determineAxisDuality(): void;
getCustomDomainValuesByposition(axisPosition: AxisPositions): any;
getOrientation(): CartesianOrientations;
getScaleByPosition(axisPosition: AxisPositions): ScaleLinear;
getScaleTypeByPosition(axisPosition: AxisPositions): ScaleTypes;
getDomainAxisScaleType(): ScaleTypes;
getRangeAxisScaleType(): ScaleTypes;
getDomainScale(): ScaleLinear;
getRangeScale(): ScaleLinear;
getMainXAxisPosition(): AxisPositions;
getMainYAxisPosition(): AxisPositions;
getMainXScale(): ScaleLinear;
getMainYScale(): ScaleLinear;
getValueFromScale(scale: any, scaleType: ScaleTypes, axisPosition: AxisPositions, datum: any): number;
getBoundedScaledValues(datum: any): number[];
getValueThroughAxisPosition(axisPosition: AxisPositions, datum: any): number;
getDomainValue(d: string | object): number;
getRangeValue(d: number | string | object): number;
getMainXScaleType(): ScaleTypes;
getMainYScaleType(): ScaleTypes;
getDomainIdentifier(datum?: any): any;
getRangeIdentifier(datum?: any): any;
extendsDomain(axisPosition: AxisPositions, domain: any): number[] | Date[];
protected findVerticalAxesPositions(): {
primary: AxisPositions;
secondary: AxisPositions;
};
protected findHorizontalAxesPositions(): {
primary: AxisPositions;
secondary: AxisPositions;
};
protected findDomainAndRangeAxesPositions(verticalAxesPositions: any, horizontalAxesPositions: any): {
primaryDomainAxisPosition: AxisPositions;
secondaryDomainAxisPosition: AxisPositions;
primaryRangeAxisPosition: AxisPositions;
secondaryRangeAxisPosition: AxisPositions;
};
getScaleDomain(axisPosition: AxisPositions): any;
protected createScale(axisPosition: AxisPositions): ScaleFunction;
getDomainLowerBound(position: any): number;
getHighestDomainThreshold(): null | {
threshold: ThresholdOptions;
scaleValue: number;
};
getHighestRangeThreshold(): null | {
threshold: ThresholdOptions;
scaleValue: number;
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy