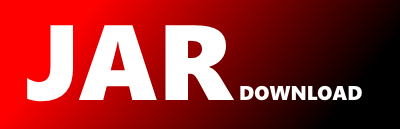
package.dist.cjs.utils.merge.cjs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react Show documentation
Show all versions of react Show documentation
Responsive and accessible React UI components built with React and Emotion
The newest version!
"use strict";
'use strict';
var is = require('./is.cjs');
function merge(target, source) {
if (source == null) return target;
for (const key of Object.keys(source)) {
if (source[key] === void 0 || key === "__proto__") continue;
if (!is.isObject(target[key]) && is.isObject(source[key])) {
Object.assign(target, { [key]: source[key] });
} else if (target[key] && is.isObject(source[key])) {
merge(target[key], source[key]);
} else if (Array.isArray(source[key]) && Array.isArray(target[key])) {
let i = 0;
for (; i < source[key].length; i++) {
if (is.isObject(target[key][i]) && is.isObject(source[key][i])) {
merge(target[key][i], source[key][i]);
} else {
target[key][i] = source[key][i];
}
}
} else {
Object.assign(target, { [key]: source[key] });
}
}
return target;
}
function mergeWith(target, ...sources) {
for (const source of sources) {
merge(target, source);
}
return target;
}
exports.mergeWith = mergeWith;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy