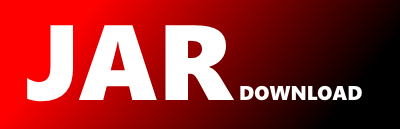
package.dist.src.internal.cache-data-client.d.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
Client SDK for Momento services
The newest version!
import { CacheDecreaseTtl, CacheDelete, CacheDictionaryFetch, CacheDictionaryGetField, CacheDictionaryGetFields, CacheDictionaryIncrement, CacheDictionaryLength, CacheDictionaryRemoveField, CacheDictionaryRemoveFields, CacheDictionarySetField, CacheDictionarySetFields, CacheGet, CacheGetBatch, CacheIncreaseTtl, CacheIncrement, CacheItemGetTtl, CacheItemGetType, CacheKeyExists, CacheKeysExist, CacheListConcatenateBack, CacheListConcatenateFront, CacheListFetch, CacheListLength, CacheListPopBack, CacheListPopFront, CacheListPushBack, CacheListPushFront, CacheListRemoveValue, CacheListRetain, CacheSet, CacheSetAddElements, CacheSetBatch, CacheSetContainsElement, CacheSetContainsElements, CacheSetFetch, CacheSetIfAbsent, CacheSetIfAbsentOrEqual, CacheSetIfEqual, CacheSetIfNotEqual, CacheSetIfNotExists, CacheSetIfPresent, CacheSetIfPresentAndNotEqual, CacheSetRemoveElements, CacheSetSample, CacheSortedSetFetch, CacheSortedSetGetRank, CacheSortedSetGetScore, CacheSortedSetGetScores, CacheSortedSetIncrementScore, CacheSortedSetLength, CacheSortedSetLengthByScore, CacheSortedSetPutElement, CacheSortedSetPutElements, CacheSortedSetRemoveElement, CacheSortedSetRemoveElements, CacheUpdateTtl, CollectionTtl, SortedSetOrder } from '..';
import { Semaphore } from '@gomomento/sdk-core/dist/src/internal/utils';
import { IDataClient } from '@gomomento/sdk-core/dist/src/internal/clients';
import { CacheClientAllProps } from './cache-client-all-props';
import { GetBatchCallOptions, GetCallOptions, SetBatchCallOptions, SetBatchItem, SetCallOptions, SetIfAbsentCallOptions } from '@gomomento/sdk-core/dist/src/utils';
import { CacheSetLength, CacheSetPop } from '@gomomento/sdk-core';
export declare const CONNECTION_ID_KEY: unique symbol;
export declare class CacheDataClient implements IDataClient {
private readonly clientWrapper;
private readonly textEncoder;
private readonly configuration;
private readonly credentialProvider;
private readonly defaultTtlSeconds;
private readonly requestTimeoutMs;
private static readonly DEFAULT_REQUEST_TIMEOUT_MS;
private readonly logger;
private readonly cacheServiceErrorMapper;
private readonly interceptors;
private readonly streamingInterceptors;
private readonly compressionDetails?;
private readonly requestConcurrencySemaphore;
/**
* @param {CacheClientProps} props
* @param dataClientID
* @param semaphore
*/
constructor(props: CacheClientAllProps, dataClientID: string, semaphore: Semaphore | undefined);
close(): void;
connect(timeoutSeconds?: number): Promise;
private connectionStateToString;
private connectWithinDeadline;
getEndpoint(): string;
private validateRequestTimeout;
private convertECacheResult;
private convertItemTypeResult;
private rateLimited;
/**
* Returns the TTL in milliseconds for a collection.
* If the provided TTL is not set, it defaults to the instance's default TTL.
* @param ttl - The CollectionTttl object containing the TTL value.
* @returns The TTL in milliseconds.
*/
private collectionTtlOrDefaultMilliseconds;
/**
* Returns the TTL in milliseconds.
* If the provided TTL is not set, it defaults to the instance's default TTL.
* @param ttl
* @returns The TTL in milliseconds.
*/
private ttlOrDefaultMilliseconds;
set(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, options?: SetCallOptions): Promise;
private sendSet;
setFetch(cacheName: string, setName: string): Promise;
private sendSetFetch;
setAddElements(cacheName: string, setName: string, elements: string[] | Uint8Array[], ttl?: CollectionTtl): Promise;
private sendSetAddElements;
setContainsElement(cacheName: string, setName: string, element: string | Uint8Array): Promise;
private sendSetContainsElement;
setContainsElements(cacheName: string, setName: string, elements: string[] | Uint8Array[]): Promise;
private sendSetContainsElements;
setRemoveElements(cacheName: string, setName: string, elements: string[] | Uint8Array[]): Promise;
private sendSetRemoveElements;
setSample(cacheName: string, setName: string, limit: number): Promise;
private sendSetSample;
setPop(cacheName: string, setName: string, count: number): Promise;
private sendSetPop;
setLength(cacheName: string, setName: string): Promise;
private sendSetLength;
setIfNotExists(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, ttl?: number): Promise;
private sendSetIfNotExists;
setIfAbsent(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, options?: SetIfAbsentCallOptions): Promise;
private sendSetIfAbsent;
setIfPresent(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, ttl?: number): Promise;
private sendSetIfPresent;
setIfEqual(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, equal: string | Uint8Array, ttl?: number): Promise;
private sendSetIfEqual;
setIfNotEqual(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, notEqual: string | Uint8Array, ttl?: number): Promise;
private sendSetIfNotEqual;
setIfPresentAndNotEqual(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, notEqual: string | Uint8Array, ttl?: number): Promise;
private sendSetIfPresentAndNotEqual;
setIfAbsentOrEqual(cacheName: string, key: string | Uint8Array, value: string | Uint8Array, equal: string | Uint8Array, ttl?: number): Promise;
private sendSetIfAbsentOrEqual;
delete(cacheName: string, key: string | Uint8Array): Promise;
private sendDelete;
get(cacheName: string, key: string | Uint8Array, options?: GetCallOptions): Promise;
private sendGet;
getBatch(cacheName: string, keys: Array, options?: GetBatchCallOptions): Promise;
private sendGetBatch;
setBatch(cacheName: string, items: Record | Map | Array, options?: SetBatchCallOptions): Promise;
private sendSetBatch;
listConcatenateBack(cacheName: string, listName: string, values: string[] | Uint8Array[], truncateFrontToSize?: number, ttl?: CollectionTtl): Promise;
private sendListConcatenateBack;
listConcatenateFront(cacheName: string, listName: string, values: string[] | Uint8Array[], truncateBackToSize?: number, ttl?: CollectionTtl): Promise;
private sendListConcatenateFront;
listFetch(cacheName: string, listName: string, startIndex?: number, endIndex?: number): Promise;
private sendListFetch;
listRetain(cacheName: string, listName: string, startIndex?: number, endIndex?: number, ttl?: CollectionTtl): Promise;
private sendListRetain;
listLength(cacheName: string, listName: string): Promise;
private sendListLength;
listPopBack(cacheName: string, listName: string): Promise;
private sendListPopBack;
listPopFront(cacheName: string, listName: string): Promise;
private sendListPopFront;
listPushBack(cacheName: string, listName: string, value: string | Uint8Array, truncateFrontToSize?: number, ttl?: CollectionTtl): Promise;
private sendListPushBack;
listPushFront(cacheName: string, listName: string, value: string | Uint8Array, truncateBackToSize?: number, ttl?: CollectionTtl): Promise;
private sendListPushFront;
listRemoveValue(cacheName: string, listName: string, value: string | Uint8Array): Promise;
private sendListRemoveValue;
dictionaryFetch(cacheName: string, dictionaryName: string): Promise;
private sendDictionaryFetch;
dictionarySetField(cacheName: string, dictionaryName: string, field: string | Uint8Array, value: string | Uint8Array, ttl?: CollectionTtl): Promise;
private sendDictionarySetField;
dictionarySetFields(cacheName: string, dictionaryName: string, elements: Map | Record | Array<[string, string | Uint8Array]>, ttl?: CollectionTtl): Promise;
private sendDictionarySetFields;
dictionaryGetField(cacheName: string, dictionaryName: string, field: string | Uint8Array): Promise;
private sendDictionaryGetField;
dictionaryGetFields(cacheName: string, dictionaryName: string, fields: string[] | Uint8Array[]): Promise;
private sendDictionaryGetFields;
dictionaryRemoveField(cacheName: string, dictionaryName: string, field: string | Uint8Array): Promise;
private sendDictionaryRemoveField;
dictionaryRemoveFields(cacheName: string, dictionaryName: string, fields: string[] | Uint8Array[]): Promise;
private sendDictionaryRemoveFields;
dictionaryLength(cacheName: string, dictionaryName: string): Promise;
private sendDictionaryLength;
increment(cacheName: string, field: string | Uint8Array, amount?: number, ttl?: number): Promise;
private sendIncrement;
dictionaryIncrement(cacheName: string, dictionaryName: string, field: string | Uint8Array, amount?: number, ttl?: CollectionTtl): Promise;
private sendDictionaryIncrement;
sortedSetPutElement(cacheName: string, sortedSetName: string, value: string | Uint8Array, score: number, ttl?: CollectionTtl): Promise;
private sendSortedSetPutElement;
sortedSetPutElements(cacheName: string, sortedSetName: string, elements: Map | Record | Array<[string, number]>, ttl?: CollectionTtl): Promise;
private sendSortedSetPutElements;
sortedSetFetchByRank(cacheName: string, sortedSetName: string, order: SortedSetOrder, startRank: number, endRank?: number): Promise;
private sendSortedSetFetchByRank;
sortedSetFetchByScore(cacheName: string, sortedSetName: string, order: SortedSetOrder, minScore?: number, maxScore?: number, offset?: number, count?: number): Promise;
private sendSortedSetFetchByScore;
sortedSetGetRank(cacheName: string, sortedSetName: string, value: string | Uint8Array, order?: SortedSetOrder): Promise;
private sendSortedSetGetRank;
sortedSetGetScore(cacheName: string, sortedSetName: string, value: string | Uint8Array): Promise;
sortedSetGetScores(cacheName: string, sortedSetName: string, values: string[] | Uint8Array[]): Promise;
private sendSortedSetGetScores;
sortedSetIncrementScore(cacheName: string, sortedSetName: string, value: string | Uint8Array, amount?: number, ttl?: CollectionTtl): Promise;
private sendSortedSetIncrementScore;
sortedSetRemoveElement(cacheName: string, sortedSetName: string, value: string | Uint8Array): Promise;
private sendSortedSetRemoveElement;
sortedSetRemoveElements(cacheName: string, sortedSetName: string, values: string[] | Uint8Array[]): Promise;
private sendSortedSetRemoveElements;
sortedSetLength(cacheName: string, sortedSetName: string): Promise;
private sendSortedSetLength;
sortedSetLengthByScore(cacheName: string, sortedSetName: string, minScore?: number, maxScore?: number): Promise;
private sendSortedSetLengthByScore;
private initializeInterceptors;
private initializeStreamingInterceptors;
private convert;
private convertArray;
private convertElements;
private convertSortedSetMapOrRecord;
private convertSetBatchElements;
itemGetType(cacheName: string, key: string | Uint8Array): Promise;
private sendItemGetType;
itemGetTtl(cacheName: string, key: string | Uint8Array): Promise;
private sendItemGetTtl;
keyExists(cacheName: string, key: string | Uint8Array): Promise;
private sendKeyExists;
updateTtl(cacheName: string, key: string | Uint8Array, ttlMilliseconds: number): Promise;
private sendUpdateTtl;
keysExist(cacheName: string, keys: string[] | Uint8Array[]): Promise;
private sendKeysExist;
increaseTtl(cacheName: string, key: string | Uint8Array, ttlMilliseconds: number): Promise;
private sendIncreaseTtl;
decreaseTtl(cacheName: string, key: string | Uint8Array, ttlMilliseconds: number): Promise;
private sendDecreaseTtl;
private createMetadata;
private toSingletonFieldValuePair;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy